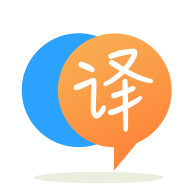
[英]How to combine two array of objects of different sizes, based on a property in Javascript?
[英]how to combine two different array of objects into one new array of object in javascript?
我有兩個對象數組,它們具有相同的news_id,但屬性不同,我只是想知道如何組合它們並獲得新的對象數組?
例如:
let arr1 = [{
news_id: 1,
title: "title1"
},
{
news_id: 2,
title: "title2"
},
{
news_id: 3,
title: "title3"
},
{
news_id: 4,
title: "title4"
},
]
let arr2 = [{
news_id: 3,
count: 3
},
{
news_id: 4,
count: 4
}
]
我想得到:
[
{news_id: 1, title: "title1", count: 0},
{news_id: 2, title: "title2", count: 0},
{news_id: 3, title: "title3", count: 3},
{news_id: 4, title: "title4", count: 4}
]
這可以如下進行。 同樣,這是通用的,它將為相同的news_id連接所有屬性,而不僅僅是計數和標題。
let arr1 = [
{news_id: 1, title: "title1"},
{news_id: 2, title: "title2"},
{news_id: 3, title: "title3"},
{news_id: 4, title: "title4"},
]
let arr2 = [
{news_id: 3, count: 3},
{news_id: 4, count: 4}
]
let result = Object.values(([...arr1, ...arr2].reduce((acc, d) => (acc[d.news_id] = { count:0, ...acc[d.news_id], ...d }, acc) ,{})))
您可以使用forEach
並按如下所示進行filter
:
arr1.forEach(i => i.count = (arr2.find(j => j.news_id == i.news_id) || { count: 0 }).count)
請在下面嘗試。
let arr1 = [{ news_id: 1, title: "title1" }, { news_id: 2, title: "title2" }, { news_id: 3, title: "title3" }, { news_id: 4, title: "title4" }, ] let arr2 = [{ news_id: 3, count: 3 }, { news_id: 4, count: 4 } ] arr1.forEach(i => i.count = (arr2.find(j => j.news_id == i.news_id) || { count: 0 }).count); console.log(arr1);
在您的情況下,以獲得理想的結果。我建議您嘗試使用老式的JS forEach()方法 。
這是完成的示例,請在JS Fiddle示例中進行檢查
let arr1 = [{
news_id: 1,
title: "title1"
},
{
news_id: 2,
title: "title2"
},
{
news_id: 3,
title: "title3"
},
{
news_id: 4,
title: "title4"
}
]
let arr2 = [
{
news_id: 3,
count: 3
},
{
news_id: 4,
count: 4
}
]
arr1.forEach(function (e,i) {
var flag = false;
arr2.forEach(function (obj, j) {
if (e.news_id === obj.news_id) {
e.count = obj.count;
flag = true;
}
});
if(!flag){
e.count = 0;
}
});
這是一個更簡單的解決方案。 我只是簡單地迭代arr1
並添加匹配的arr2
項(如果有)中的count
。
for (item of arr1) {
let arr2item = arr2.find(item2 => item2.news_id === item.news_id);
item['count'] = arr2item ? arr2item.count : 0;
};
@Nitish的回答看起來不錯,但是意圖不是很清楚。
您可以使用Map
來收集所有給定的數據和計數。 然后渲染最終結果。
var array1 = [{ news_id: 1, title: "title1" }, { news_id: 2, title: "title2" }, { news_id: 3, title: "title3" }, { news_id: 4, title: "title4" }], array2 = [{ news_id: 3, count: 3 }, { news_id: 4, count: 4 }], result = Array.from( array2 .reduce( (m, { news_id, count }) => (m.get(news_id).count += count, m), array1.reduce((m, o) => m.set(o.news_id, Object.assign({}, o, { count: 0 })), new Map) ) .values() ); console.log(result);
.as-console-wrapper { max-height: 100% !important; top: 0; }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.