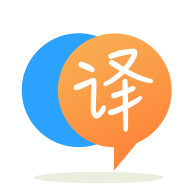
[英]Multidimensional array in php( have same key different values) how can I create a new name for the key?
[英]I have a below array with some same keys with different values. How can I combine same keys values with that key in PHP? I am using WAMP, Codeigniter
我從導入CSV文件獲取這些數據。 在CSV文件中,我的第一列是Years,所有其他列是Make。 我正在使用csvreader庫從CSV文件解析數據。
我的CSVReader庫。
class CI_Csvreader {
var $fields; /** columns names retrieved after parsing */
var $separator = ','; /** separator used to explode each line */
/**
* Parse a text containing CSV formatted data.
*
* @access public
* @param string
* @return array
*/
function parse_text($p_Text) {
$lines = explode("\n", $p_Text);
return $this->parse_lines($lines);
}
/**
* Parse a file containing CSV formatted data.
*
* @access public
* @param string
* @return array
*/
function parse_file($p_Filepath) {
$lines = file($p_Filepath);
return $this->parse_lines($lines);
}
/**
* Parse an array of text lines containing CSV formatted data.
*
* @access public
* @param array
* @return array
*/
function parse_lines($p_CSVLines) {
$content = FALSE;
foreach( $p_CSVLines as $line_num => $line ) {
if( $line != '' ) { // skip empty lines
$elements = explode($this->separator, $line);
if( !is_array($content) ) { // the first line contains fields names
$this->fields = $elements;
$content = array();
} else {
$item = array();
foreach( $this->fields as $id => $field ) {
if( isset($elements[$id]) ) {
$item[$field] = $elements[$id];
}
}
$content[] = $item;
}
}
}
return $content;
}
我的CSV文件數據=>
Years Make Make Make
2001 Acura Honda Toyota
2002 Acura Honda
2003 Acura Toyota
2004
在上面的文件Years and Make中,Excel / CSV表格中的數據以后可以更改。
我的輸出是一個數組。=>
Array
(
[0] => Array
(
[Years] => 2001
[Make] => Acura
[Make] => Honda
[Make] => Toyota
)
[1] => Array
(
[Years] => 2002
[Make] => Acura
[Make] => Honda
[Make] =>
)
[2] => Array
(
[Years] => 2003
[Make] => Acura
[Make] =>
[Make] => Toyota
)
[3] => Array
(
[Years] => 2004
[Make] =>
[Make] =>
[Make] =>
)
)
我想要這樣的結果數組=>我想保留空值。
Array
(
[0] => Array
(
[Years] => 2001
[Make] => Array(
[0]=>Acura
[1]=>Honda
[2]=>Toyota
)
)
[1] => Array
(
[Years] => 2002
[Make] => Array(
[0]=>Acura
[1]=>Honda
[2]=>
)
)
[2] => Array
(
[Years] => 2003
[Make] => Array(
[0]=>Acura
[1]=>
[2]=>Toyota
)
)
[3] => Array
(
[Years] => 2004
[Make] => Array(
[0]=>
[1]=>
[2]=>
)
)
)
另外,請告訴我如何獲取沒有空值的結果。
如果還有其他方法可以以我想要的格式從CSV文件中獲取數據,那就可以了。
誰能幫幫我嗎。 非常感謝你。
您可以使用此功能來解析CSV文件
function CSV_parse($string='', $has_header=true, $row_delimiter=PHP_EOL, $delimiter = "," , $enclosure = '"' , $escape = "\\" )
{
$rows = array_filter(explode($row_delimiter, $string));
$firstline = true;
$data = array();
foreach($rows as $row)
{
if($firstline && $has_header)
{
$firstline=false;
continue;
}
$row = str_getcsv ($row, $delimiter, $enclosure , $escape);
$dtrow=array('Years'=>$row[0], 'Makes'=>array());
for($i=1;$i<count($row);$i++)
{
$make=trim($row[$i]);
if($make=='')continue;
$dtrow['Makes'][]=$make;
}
$data[] =$dtrow;
}
return $data;
}
您應該將CSV文件內容作為第一個參數傳遞。 對於第二個參數,如果csv文件沒有標題,則應傳遞false
編輯==>
您可以只修改此類中的幾行以獲得所需的結果。 看一下PTK ==>和<== PTK之間的界線:
class CI_Csvreader {
var $fields; /** columns names retrieved after parsing */
var $separator = ','; /** separator used to explode each line */
/**
* Parse a text containing CSV formatted data.
*
* @access public
* @param string
* @return array
*/
function parse_text($p_Text) {
$lines = explode("\n", $p_Text);
return $this->parse_lines($lines);
}
/**
* Parse a file containing CSV formatted data.
*
* @access public
* @param string
* @return array
*/
function parse_file($p_Filepath) {
$lines = file($p_Filepath);
return $this->parse_lines($lines);
}
/**
* Parse an array of text lines containing CSV formatted data.
*
* @access public
* @param array
* @return array
*/
function parse_lines($p_CSVLines) {
$content = FALSE;
foreach( $p_CSVLines as $line_num => $line ) {
if( $line != '' ) { // skip empty lines
$elements = explode($this->separator, $line);
if( !is_array($content) ) { // the first line contains fields names
$this->fields = $elements;
$content = array();
} else {
//PTK: ==> new code
$item=array('Years'=>$elements[0], 'Makes'=>array());
for($i=1;$i<count($elements);$i++)
{
$make=trim($elements[$i]);
//if($make=='')continue; //PTK: if you want to remove empty lines uncoment this line, but in this case your array may will have rows with different lengthes
$item['Makes'][]=$make;
}
//PTK: <== new code
/*
//PTK ==> original code
$item = array();
foreach( $this->fields as $id => $field ) {
if( isset($elements[$id]) ) {
$item[$field] = $elements[$id];
}
}
//PTK <== original code
*/
$content[] = $item;
}
}
}
return $content;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.