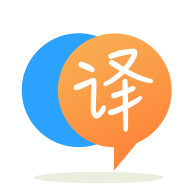
[英]Calling a javascript function from a global file and return it to append to html
[英]Calling a Function in JavaScript to Return HTML
我正在嘗試使用函數checkLength來測試用戶輸入的數字是否為 16 位數字。 如果是 16 位,我想繼續我的函數main ; 否則我想輸出錯誤消息“請輸入 16 位數字” 。 我的代碼如下:
function main() { "use strict"; // set variable for user-entered number var userNum = document.getElementById("userNum"), // use that variable to initialize array array = [userNum.value], // convert array to string values = array.toString(); // call function "checkLength" with parameter "values" checkLength(values); // more code below that is excluded for simplification } function checkLength(x) { "use strict"; if (x.length !== 16) { return document.getElementById("output").innerHTML = "Please enter a 16-digit number."; } else { return false; } }
這段代碼的問題是每次執行時,我都會得到輸出“請輸入 16 位數字”。 ,即使我輸入了 16 位數字。
我已經嘗試調試了幾個小時,但無法確定我哪里出錯了。 我不一定在尋找解決方案,而只是我應該尋找的一般方向(不正確的范圍、返回語句等)。 我非常感謝您的幫助!
在這里我可以看到,當輸入不是 16 位長的數字時設置了輸出,並且它會保留在 DOM 中。
我假設 main() 在文本框或任何按鈕的任何事件上被調用。
解決方案是,如果輸入長度為 16 位,您需要重置“輸出”元素。
請更改您的 checkLength 函數,如下所示,
function checkLength(x) {
"use strict";
if (x.length !== 16) {
return document.getElementById("output").innerHTML = "Please enter a 16-digit number.";
}
else {
//Added below line of code.
document.getElementById("output").innerHTML="";
return false;
}
}
======================================
另一個改進應該是使用文本的“長度”屬性。
使用 length 屬性獲取文本框值的長度,如下所示,
function main() {
"use strict";
// set variable for user-entered number
var userNum = document.getElementById("userNum");
//variable to hold length of input box
inputLength = userNum.value.length;
// call function "checkLength" with parameter "values"
checkLength(inputLength);
// more code below that is excluded for simplification
}
正如@Parth Lukhi 的其他答案一樣,您必須將 html 放在checkLength
,並且您還必須放置像if(!checkLength(values))
然后它應該return
而不是進一步執行。
function main() { "use strict"; // set variable for user-entered number var userNum = document.getElementById("userNum"), // use that variable to initialize array array = [userNum.value], // convert array to string values = array.toString(); // call function "checkLength" with parameter "values" if(!checkLength(values)) return; // more code below that is excluded for simplification } function checkLength(x) { "use strict"; if (x.length !== 16) { document.getElementById("output").innerHTML = "Please enter a 16-digit number."; return true; } else { return document.getElementById("output").innerHTML = ""; return false; } }
function main() { "use strict"; var a = document.getElementById("userNum").value; checkLength(a); } function checkLength(x) { "use strict"; if (x.length != 16) { return document.getElementById("output").innerHTML = "Please enter a 16-digit number."; } else { document.getElementById("output").innerHTML = " entered value is 16 digit"; } }
<input type="text" id="userNum" value="Mickey are you ?"> <button onclick="main()">click me</button><div id="output"></div>
希望這對你有幫助:)
這是因為您的checkLength()
只返回false
並且沒有寫入 html 消息
function main() { "use strict"; // set variable for user-entered number var userNum = document.getElementById("userNum"), // use that variable to initialize array array = [userNum.value], // convert array to string values = array.toString(); // call function "checkLength" with parameter "values" checkLength(values); // more code below that is excluded for simplification } function checkLength(x) { "use strict"; var message, isValid = false; if (x.length !== 16) { message = "Please enter a 16-digit number. (" + x.length + ")"; } else { isValid = true; message = "Correct: " + x; } document.getElementById("output").innerHTML = message; return isValid; }
<input type="number" id="userNum" oninput="main()"> <p id="output"> 0 </p>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.