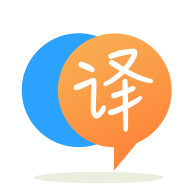
[英]How to replace first number from each line of a ".txt" file in python?
[英]how to replace first line of a file using python or other tools?
我有一個名為hanlp.properties
的文件:
root=/Users/pan/Documents
other content
我想傳遞一個參數“/User/a/b”並替換根路徑
root=/User/a/b
other content
/User/a/b
是一個參數。
如何使用 python 或任何其他工具實現該目標?
使用 Python 3:
import argparse
from sys import exit
from os.path import getsize
# collect command line arguments
parser = argparse.ArgumentParser()
parser.add_argument('-p', '--parameter', required=True, type=str)
parser.add_argument('-f', '--file', required=True, type=str)
args = parser.parse_args()
# check if file is empty
if getsize(args.file) == 0:
print('Error: %s is empty' % args.file)
exit(1)
# collect all lines first
lines = []
with open(args.file) as file:
first_line = next(file)
# Check if '=' sign exists in first line
if '=' not in first_line:
print('Error: first line is invalid')
exit(1)
# split first line and append new line
root, parameter = first_line.split('=')
lines.append('%s=%s\n' % (root, args.parameter))
# append rest of lines normally
for line in file:
lines.append(line)
# rewrite new lines back to file
with open(args.file, 'w') as out:
for line in lines:
out.write(line)
其工作原理如下:
$ cat hanlp.properties
root=/Users/pan/Documents
other content
$ python3 script.py --file hanlp.properties --parameter /Users/a/b
$ cat hanlp.properties
root=/User/a/b
other content
這可能對你有幫助。
import os
infile=os.path.join(os.getcwd(),"text.txt")
data = open(infile).readlines()
print(data)
data[0] = 'new content of;lkdsajv;ldsahbv;ikj'+'\n'
print(data)
with open(infile, 'w') as fw:
fw.writelines(data)
編輯:由於 OP 已更改要求,因此現在也添加此解決方案。 用 GNU awk
對此進行了測試。 附加> temp_file && mv temp_file Input_file
以防您想將輸出保存到 Input_file 本身。
awk -F'=' 'FNR==NR{if($0~/root/){value=$2};nextfile} /root/{$2=value} 1' OFS="=" parameter_file Input_file
說明:在此處也添加對上述代碼的說明。
awk -F'=' ' ##Mentioning field separator as = here for all lines of all mentioned passed Input_files to awk.
FNR==NR{ ##Checking condition FNR==NR which will be TRUE when parameter_file is being read.
if($0~/root/){ ##Checking if a line has root string in it then do following.
value=$2 ##Assigning value of $2 to variable value here.
}
nextfile ##nextfile will jump to next passed Input_file and all further lines for parameter file will be skipped.
}
/root/{ ##Checking if a line has string root in it then do following.
$2=value ##Setting 2nd field value as value variable here.
}
1 ##By mentioning 1 telling awk to print edited/no-edited lines here.
' OFS="=" parameter_file Input_file ##Mentioning OFS value as = here and mentioning Input_file(s) name here.
它應該是簡單的sed
程序。 如果您想將輸出保存到 Input_file 本身,請使用sed -i
選項。
sed '/root=/s/path1/path2/' Input_file
如果您想使用awk
那么以下內容可能會對您有所幫助。
awk '/root=/{sub("path1","path2")} 1' Input_file > temp_file && mv temp_file Input_file
我寫了一個shell a.sh
if [ ! -n "$1" ] ;then
echo "Please input hanlp data path!"
else
echo "The hanlp data path you input is $1"
var="root="
path=$var$1
sed -i '/^root/c'$path'' hanlp.properties
fi
然后
chmod 777 a.sh
跑:
./a.sh /User/a/b
從 HanLP v1.7 開始,您可以使用除配置文件之外的許多方法來設置根。 看到這個問題,你可能需要谷歌翻譯。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.