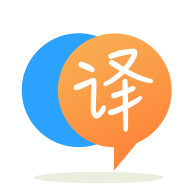
[英]Spring Boot injecting Map values from .properties and .yaml using ConfigurationProperties
[英]Add YAML values to std map using YAML-CPP
我有一個yaml文件,里面有嵌套的地圖:
SOLAR-SYSTEM:
my/planet:
earth: blue
my/satellite:
moon: white
我正在使用yaml-cpp來解析這些值。
有什么辦法可以從 yaml 文件中提取這些值並將它們添加到stl 映射中?
這讓我想到了問題的第二部分。
我對 C++ 相當陌生,所以不太確定地圖在其中是如何工作的。
在 Java 中,我使用snakeyaml解析相同的 yaml 文件。
它將映射添加到三重哈希映射數據結構中:
HashMap<String, Map<String, Map<String, String>>>
我可以很方便地完成這個。
有沒有什么簡單的方法可以在 C++ 中做這樣的事情?
我已經將這個很棒的庫用於 YAML 解析器 2 天了。 所以,我可能也有一些錯誤。 我使用 yaml-cpp ver0.6.2。
主要思想是建立自己的結構。 之后,模板特化用於特定類型的轉換。
我覺得你的文檔結構不是很好。 感覺就像是為 std::map 嵌套。 我覺得你可以看這個例子只針對 yaml 文件,因為這些 API 都是舊的
最后,您可以將值拉入您構建的結構中。
對不起,我英語不好。 所以給你看我的代碼。 如果你有新的問題,你可以再問我。
另外,代碼作者在這里。 你可能會從他那里得到更准確的答案。
struct Planet {
std::string earth;
};
struct Satellite {
std::string moon;
};
struct SolarSystem {
Planet p;
Satellite s;
};
namespace YAML {
template<>
struct convert<Planet> {
static Node encode(const Planet &rhs) {
Node node;
node["earth"] = rhs.earth;
return node;
}
static bool decode(const Node &node, Planet &rhs) {
if (!node.IsMap())
return false;
rhs.earth = node["earth"].as<std::string>();
return true;
}
};
template<>
struct convert<Satellite> {
static Node encode(const Satellite &rhs) {
Node node;
node["moon"] = rhs.moon;
return node;
}
static bool decode(const Node &node, Satellite &rhs) {
if (!node.IsMap())
return false;
rhs.moon = node["moon"].as<std::string>();
return true;
}
};
template<>
struct convert<SolarSystem> {
static Node encode(const SolarSystem &rhs) {
Node node;
node["my/planet"] = rhs.p;
node["my/satellite"] = rhs.s;
return node;
}
static bool decode(const Node &node, SolarSystem &rhs) {
if (!node.IsMap())
return false;
rhs.p = node["my/planet"].as<Planet>();
rhs.s = node["my/satellite"].as<Satellite>();
return true;
}
};
}
int main(void)
{
YAML::Node doc = YAML::LoadFile("path/to/your/file");
SolarSystem ss = doc["SOLAR-SYSTEM"].as<SolarSystem>();
std::cout << ss.p.earth << std::endl; // "blue"
std::cout << ss.s.moon << std::endl; // "white"
return 0;
}
不知道為什么它被否決了。 感謝@Crow 的回答。 我無法使用它,因為它涉及對值進行硬編碼。 這是我設計的解決方案:
try {
YAML::Node firstTierNode = YAML::LoadFile("config.yml");
std::map<std::string, std::map<std::string, std::map<std::string, std::string>>> newMap1;
for (YAML::const_iterator it = firstTierNode.begin(); it != firstTierNode.end(); ++it) {
string level1First = it->first.as<std::string>();
YAML::Node secondTierNode = it->second;
std::map<std::string, std::map<std::string, std::string>> newMap2;
for (YAML::const_iterator it = secondTierNode.begin(); it != secondTierNode.end(); ++it) {
string level2First = it->first.as<std::string>();
YAML::Node thirdTierNode = it->second;
std::map<std::string, std::string> newMap3;
for (YAML::const_iterator it = thirdTierNode.begin(); it != thirdTierNode.end(); ++it) {
string level3First = it->first.as<std::string>();
string level3SecondString = it->second.as<std::string>();
newMap3.insert(std::pair<std::string, std::string>(level3First, level3SecondString));
}
newMap2.insert(std::pair<std::string, std::map<string, string>>(level2First, newMap3));
}
newMap1.insert(std::pair<std::string, std::map<string, std::map<string, string>>>(level1First, newMap2));
}
for (const auto& x : newMap1) {
std::cout <<x.first << endl << endl;
for (const auto& y : x.second) {
std::cout << y.first << endl << endl;
for (const auto& z : y.second) {
std::cout << z.first << endl << z.second << endl << endl << endl;
}
}
}
return 1;
}
catch (exception& e) {
cerr <<e.what()<< endl;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.