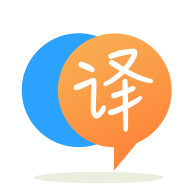
[英]Request object sent to django form ok when sent via GET but empty when sent via POST
[英]Django Post request not sent on button click
我正在嘗試在 Django 中創建一個簡單的 Web 應用程序並嘗試使用 Ajax,因此頁面不會刷新。 這個應用程序的唯一目標是讓表單接受一些用戶輸入,並且在提交表單時不會刷新。 但是,由於某種原因,當我單擊按鈕時不會發生這種情況。 這是索引頁面:
<!DOCTYPE html>
<html>
<body>
<h2>Create product here</h2>
<div>
<form id="new_user_form">
<div>
<label for="name" > Name:<br></label>
<input type="text" id="name"/>
</div>
<br/>
<div>
<label for="email"> email:<br></label>
<input type="text" id="email"/>
</div>
<div>
<label for="password" > password:<br></label>
<input type="text" id="password"/>
</div>
<div>
<input type="submit" value="submitme"/>
</div>
</form>
</body>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type = "text/text/javascript">
$(document).on('submitme', '#new_user_form', function(e)){
e.preventDefault()
$.ajax({
type: 'POST',
url:'/user/create',
data:{
name:$('#name').val(),
email:$('#email').val(),
password:$('#password').val(),
}
success.function(){
alert('created')
}
})
}
</script>
</html>
這是我的主要 urls.py 文件:
from django.contrib import admin
from django.urls import path
from django.conf.urls import include, url
from testapp import views
import testapp
from django.views.decorators.csrf import csrf_exempt
urlpatterns = [
path('admin/', admin.site.urls),
url(r'^$', testapp.views.index),
url(r'^user/create/$', csrf_exempt(testapp.views.create_user))
]
我的views.py 文件:
from django.shortcuts import render
from testapp.models import User
from django.http import HttpResponse
# Create your views here.
def index(request):
return render(request, 'index.html')
def create_user(request):
if request.method == 'POST':
name = request.POST['name']
email = request.POST['email']
password = request.POST['password']
User.objects.create(
name = name,
email = email,
password = password
)
return HttpResponse('')
最后是 models.py 文件:
from django.db import models
# Create your models here.
class User(models.Model):
name = models.CharField(max_length = 32)
email = models.EmailField()
password = models.CharField(max_length = 128)
這樣做的目的是當單擊按鈕時,它應該向后端發送一個 POST 請求,該請求創建一個 User 類型的對象並將其保存到數據庫中。 但是,由於某種原因,當我單擊提交時,根據 Chrome 上的網絡工具,沒有發送任何 POST 請求。 有人可以幫我嗎?
def create_user(request):
if request.method == 'POST':
form = SignUpForm(request.POST)
if form.is_valid():
form.save()
username = form.cleaned_data.get('username')
raw_password = form.cleaned_data.get('password')
user = authenticate(username=username, password=raw_password)
auth_login(request, user)
return render(request, 'accounts/index.html')
else:
form = SignUpForm()
return render(request, 'accounts/signup.html', {'form': form})
您的代碼應該看起來更像這樣。 在這里,我使用默認的 django 身份驗證系統,因此不需要你的model.py
,至少現在不需要。 另請查看我添加的渲染 - 使用HttpReponse
您的頁面將重新加載。 電子郵件會自動保存為form.submit()
SignUpForm 應該很簡單:
class SignUpForm(UserCreationForm):
email = forms.EmailField(max_length=254, help_text='Required.')
您的代碼看起來不錯,但我想幫助您進行以下更改:我編輯並更新了我的帖子,因為我之前的建議中的一些部分不適用於您的情況(因為 ajax contentType 在您的情況下是可以的等等.. .),所以我為您的目的清除了我的答案:
1.
在HTML 表單中,輸入名稱應該在輸入字段中給出,因為這樣更容易使用 AJAX 提交輸入的 HTML 表單值:
<input type="text" name="name" id="name"/>
<input type="email" name="email" id="email"/>
<input type="password" name="password" id="password"/>
提交按鈕應更改如下:
<button type="button" name="submitme" id="submitme">Submit</button>
2.
您的AJAX 調用應該像這樣重新制定(我認為您的url中缺少尾部斜杠,並且數據的格式可能比您制作的更簡單,並且單擊功能現在在按鈕 id 上。並且代碼上的右括號已清除:
<script type = "text/text/javascript">
var $ = jQuery.noConflict();
$( document ).ready(function() {
$('#submitme').on('click', function(e){
e.preventDefault();
$.ajax({
type: 'POST',
url:'/user/create/',
data: $('form').serialize(),
success: function(){
alert('created');
}
})
})
});
</script>
您的視圖應該如下所示,以獲取 ajax 提交的數據並保存為新用戶(修改非常小):
def create_user(request):
if request.method == 'POST':
name = request.POST.get('name')
email = request.POST.get('email')
password = request.POST.get('password')
new_user = User.objects.create(
name = name,
email = email,
password = password
)
new_user.save()
return HttpResponse('')
這樣它現在必須工作。 我希望這會對你有所幫助。
不確定這個答案有多相關。 但是沒有為您創建“POST”請求的唯一原因是因為您在 JS 中編寫了<script type = "text/text/javascript">
而不是<script type = "text/javascript">
嘗試將 method="POST" 和 action="{% url 'url_name' %}" 添加到 html 表單。 還將名稱添加到 url 以創建用戶。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.