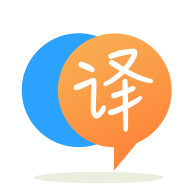
[英]Android Tutorials — Why does my app crash when I try to access an existing Text View
[英]Getting Error and App crash When I try to Access current location
public void onMapReady(GoogleMap googleMap) {
Toast.makeText(this, "Map is Ready", Toast.LENGTH_SHORT).show();
Log.d(TAG, "onMapReady: map is ready");
mMap = googleMap;
if (mLocationPermissionsGranted) {
getDeviceLocation();
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this,
Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
return;
}
mMap.setBuildingsEnabled(true);
mMap.getUiSettings().setMyLocationButtonEnabled(false);
}
}
private void getDeviceLocation() {
Log.d(TAG, "getDeviceLocation: Getting the current location");
mFusedLocationProviderClient = LocationServices.getFusedLocationProviderClient(this);
try {
if (mLocationPermissionsGranted) {
final com.google.android.gms.tasks.Task<Location> location = mFusedLocationProviderClient.getLastLocation();
location.addOnCompleteListener(new OnCompleteListener<Location>() {
@Override
public void onComplete(@NonNull com.google.android.gms.tasks.Task<Location> task) {
if(task.isSuccessful()){
Log.d(TAG, "onComplete: found Location");
Location currentLocation = (Location) task.getResult();
moveCamera(new LatLng(currentLocation.getLatitude() ,currentLocation.getLongitude()),DEFAULT_ZOOM);
}else{
Log.d(TAG, "onComplete: current location is null");
Toast.makeText(MapActivity.this,"Unable to get current location", Toast.LENGTH_SHORT).show();
}
}
});
}
} catch (SecurityException e) {
Log.e(TAG, "getDeviceLocation: SecurityException " + e.getMessage());
}
}
錯誤:E / AndroidRuntime:致命異常:主進程:com.uws.pnai.mapsapi,PID:15830 java.lang.NullPointerException:嘗試在空對象上調用虛擬方法'double android.location.Location.getLatitude()' com.uws.pnai.mapsapi.MapActivity $ 1.onComplete(MapActivity.java:77)上的com.google.android.gms.tasks.zzf.run(未知源)上的參考android.os.Handler.handleCallback(Handler。 java:751)位於android.os.Handler.dispatchMessage(Handler.java:95)位於android.os.Looper.loop(Looper.java:154)位於android.app.ActivityThread.main(ActivityThread.java:6780)位於com.android.internal.os.ZygoteInit.main(ZygoteInit.java)上的java.lang.reflect.Method.invoke(本機方法)com.android.internal.os.ZygoteInit $ MethodAndArgsCaller.run(ZygoteInit.java:1500) :1390)
首先將此實現添加到您的build.gradle
文件中
compile 'com.google.android.gms:play-services-location:11.2.0'
在實現之后,在您的活動或片段中實現(實現LocationListener
),然后實現其功能,然后
在您的onCreate()
getLocation();
調用此方法getLocation();
然后在此函數中添加這些行
protected void getLocation() {
if (isLocationEnabled(MainActivity.this)) {
locationManager = (LocationManager) this.getSystemService(Context.LOCATION_SERVICE);
criteria = new Criteria();
bestProvider = String.valueOf(locationManager.getBestProvider(criteria, true)).toString();
//You can still do this if you like, you might get lucky:
Location location = locationManager.getLastKnownLocation(bestProvider);
if (location != null) {
Log.e("TAG", "GPS is on");
latitude = location.getLatitude();
longitude = location.getLongitude();
Toast.makeText(MainActivity.this, "latitude:" + latitude + " longitude:" + longitude, Toast.LENGTH_SHORT).show();
searchNearestPlace(voice2text);
}
else{
//This is what you need:
locationManager.requestLocationUpdates(bestProvider, 1000, 0, this);
}
}
else
{
//prompt user to enable location....
//.................
}
}
之后,在您的onLocationChanged(Location location)中添加以下代碼行
@Override
public void onLocationChanged(Location location) {
//Hey, a non null location! Sweet!
//remove location callback:
locationManager.removeUpdates(this);
//open the map:
latitude = location.getLatitude();
longitude = location.getLongitude();
Toast.makeText(MainActivity.this, "latitude:" + latitude + " longitude:" + longitude, Toast.LENGTH_SHORT).show();
searchNearestPlace(voice2text);
}
然后您就可以出發了!!! 歡呼快樂編碼
如果要持續更新位置,可以使用后台服務。下面的代碼用於位置服務
public class LocationTracking extends Service {
private static final String TAG = "BOOMBOOMTESTGPS";
private LocationManager mLocationManager = null;
private static final int LOCATION_INTERVAL = 10000;
private static final float LOCATION_DISTANCE = 0;
double latitude; // latitude
double longitude; // longitude
String emp_id=Login.strUserID;;
View view;
private class LocationListener implements android.location.LocationListener
{
Location mLastLocation;
public LocationListener(String provider)
{
Log.e(TAG, "LocationListener " + provider);
mLastLocation = new Location(provider);
}
@Override
public void onLocationChanged(Location location)
{
ConnectivityManager cn = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo nf = cn.getActiveNetworkInfo();
if (nf != null && nf.isConnected() == true) {
Log.e(TAG, "onLocationChanged: " + location);
latitude=location.getLatitude();
longitude=location.getLongitude();
SendQueryString(latitude,longitude);
Log.d("tag","lat"+latitude+"Long"+longitude+"");
Toast.makeText(LocationTracking.this,"Lat and Long"+location+"emp_id"+emp_id+"",Toast.LENGTH_LONG).show();
mLastLocation.set(location);
}else{
Toast.makeText(LocationTracking.this,"Bad network",Toast.LENGTH_LONG).show();
showErrorToast(view);
}
}
public void showErrorToast(View view) {
MDToast.makeText(LocationTracking.this, "Bad Network Connection", MDToast.LENGTH_LONG, MDToast.TYPE_ERROR);
}
@Override
public void onProviderDisabled(String provider)
{
Log.e(TAG, "onProviderDisabled: " + provider);
}
@Override
public void onProviderEnabled(String provider)
{
Log.e(TAG, "onProviderEnabled: " + provider);
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras)
{
Log.e(TAG, "onStatusChanged: " + provider);
}
}
LocationListener[] mLocationListeners = new LocationListener[] {
new LocationListener(LocationManager.GPS_PROVIDER),
//new LocationListener(LocationManager.NETWORK_PROVIDER)
};
@Override
public IBinder onBind(Intent arg0)
{
return null;
}
@Override
public int onStartCommand(Intent intent, int flags, int startId)
{
Log.e(TAG, "onStartCommand");
super.onStartCommand(intent, flags, startId);
return START_STICKY;
}
@Override
public void onCreate()
{
Log.e(TAG, "onCreate");
initializeLocationManager();
/* try {
mLocationManager.requestLocationUpdates(
LocationManager.NETWORK_PROVIDER, LOCATION_INTERVAL, LOCATION_DISTANCE,
mLocationListeners[1]);
} catch (java.lang.SecurityException ex) {
Log.i(TAG, "fail to request location update, ignore", ex);
} catch (IllegalArgumentException ex) {
Log.d(TAG, "network provider does not exist, " + ex.getMessage());
}*/
try {
mLocationManager.requestLocationUpdates(
LocationManager.GPS_PROVIDER, LOCATION_INTERVAL, LOCATION_DISTANCE,
mLocationListeners[0]);
} catch (java.lang.SecurityException ex) {
Log.i(TAG, "fail to request location update, ignore", ex);
} catch (IllegalArgumentException ex) {
Log.d(TAG, "gps provider does not exist " + ex.getMessage());
}
}
@Override
public void onDestroy()
{
Log.e(TAG, "onDestroy");
super.onDestroy();
if (mLocationManager != null) {
for (int i = 0; i < mLocationListeners.length; i++) {
try {
mLocationManager.removeUpdates(mLocationListeners[i]);
} catch (Exception ex) {
Log.i(TAG, "fail to remove location listners, ignore", ex);
}
}
}
}
private void initializeLocationManager() {
Log.e(TAG, "initializeLocationManager");
if (mLocationManager == null) {
mLocationManager = (LocationManager)getApplicationContext().getSystemService(Context.LOCATION_SERVICE);
}
}
像這樣在MainActivity中調用此服務
try {
startService(new Intent(RegularCustomerBilling.this, LocationTracking.class));
} catch (Exception e) {
}
And in manifest mention that service
<service
android:name=".LocationTracking"
android:enabled="true"
android:exported="true" />
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.