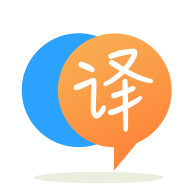
[英]multidimensional json data into different input text boxes through jquery/ajax
[英]Dynamic dependency of select boxes via Ajax on Laravel but getting data through different tables, how to?
我試圖以國家->州->自治市鎮的方式,從上面的一個表格中選擇一組3個選擇框。
我正在關注本教程 , 該教程成功獲取數據並填充了第一個選擇框(在本例中為Country)。
問題在於,在該教程中,數據位於單個表中 ,而在我的應用程序中,數據位於多個表中。
我想知道如何在與上述選擇框中的所選項目的ID相對應的多個表中獲取數據? 但是以LARAVEL的方式?
我的HTML(全部基於上面鏈接的教程):
{{-- Muestra los estados sacados de la base de datos. --}}
<div id="inputs-estado">
<div class="form-group">
{{-- Estados --}}
<label for="">Estado</label>
<select name="state" id="state" class="form-control dynamic" data-dependant="state">
@foreach ($estados as $estado)
<option value="{{ $estado->state }}">{{ $estado->state }}</option>
@endforeach
</select>
<br>
{{-- Municipio/Delegación --}}
<label for="">Ciudad</label>
<select name="state" id="state" class="form-control dynamic" data-dependant="city">
<option value="">Selecciona la ciudad</option>
</select>
<br>
{{-- Colonia --}}
<label for="">Municipo</label>
<select name="state" id="state" class="form-control dynamic" data-dependant="municipality">
<option value="">Selecciona el municipio</option>
</select>
</div>
</div>
JS:
formDynamic.change(function () {
if ($(this).val() != '') {
let select = $(this).attr('id');
let value = $(this).val();
let dependent = $(this).data('dependent');
let _token = $('input[name="_token"]').val();
$.ajax({
url: "{{ route('postscontroller.fetch') }}",
method: "POST",
data: {
select: select,
value: value,
_token: _token,
dependent: dependent
},
success: function (result) {
$('#' + dependent).html(result);
}
})
}
});
控制器:
public function create()
{
// Toma los estados de la base de datos.
$estados = DB::connection('db_postalcodes')
->table('state')
->groupBy('state')
->get();
// El with hace que se adjunten variables al view.
return view('admin.posts.create')->with('estados', $estados);
}
public function fetch(Request $request)
{
$state_id = DB::connection('db_postalcodes')->table('city')->get();
$select = $request->get('select');
$value = $request->get('value');
$dependent = $request->get('dependent');
$data = DB::connection('db_postalcodes')
->table('city')
->where($select, $state_id->state_id)
->groupBy($dependent)
->get();
$output = '<option value="">Select '.ucfirst($dependent).'</option>';
foreach($data as $row){
$output .= '<option value="'.$row->$dependent.'">'.$row->$dependent.'</option>';
}
echo $output;
}
routes.php文件
Route::group(['prefix' => 'admin', 'namespace' => 'Admin', 'middleware' => 'auth'], function () {
Route::get('/', 'AdminController@index')->name('admin');
Route::get('posts', 'PostsController@index')->name('admin.posts.index');
Route::get('posts/create', 'PostsController@create')->name('admin.posts.create');
Route::post('posts/create', 'PostsController@fetch')->name('postscontroller.fetch');
Route::post('posts', 'PostsController@store')->name('admin.posts.store');
});
我的桌子:
Laravel的模型和關系可以在這里大有幫助。 特別是有hasManyThrough
。 查看文檔以獲取更多詳細說明。
您將需要三個模型:國家/地區,州和城市。 您可以使用artisan通過php artisan make:model modelName
制作,也可以在您的項目中手動創建它們。 無論哪種方式,它都應如下所示:
國家模型
use Illuminate\Database\Eloquent\Model;
class Country extends Model {
// A Country can have many Municipalities but they do not directly belong
// to the Country they belong to the State -- Which belongs to the Country
public function municipalities() {
return $this->hasManyThrough('App\Municipality', 'App\State');
}
// Each Country can have many States
public function states() {
return $this->hasMany('App\State');
}
}
狀態模型
use Illuminate\Database\Eloquent\Model;
class State extends Model {
// Assuming each State can only belong to One Country
public function country() {
return $this->belongsTo('App\Country');
}
// Each State can have many Municipalities
public function municipalities() {
return $this->hasMany('App\Municipalities');
}
}
市政模型
use Illuminate\Database\Eloquent\Model;
class Municipality extends Model {
// Assuming each Municipality can belong to only one State
public function state() {
return $this->belongsTo('App\State');
}
// Should you ever need this Municipality's Country
public function country() {
return $this->state->country;
}
}
這些都在您具有類似於以下表格結構的假設下進行:
國家:
| id | name | another_column |
-----------------------------
1 | USA |
狀態:
| id | country_id | name | another_col |
----------------------------------------
1 | 1 | OK |
直轄市:
| id | state_id | postalcode_id | name | another_col |
------------------------------------------------------
1 | 1 | 1 | OKC |
郵政編碼:
| id | state_id | postal_code |
-------------------------------
1 | 1 | 73102 |
至於您的控制器,您可以將其分為3個端點: getCountries
, getStatesByCountry
, getCitiesByState
每個端點都基於傳遞給它的ID獲取數據。
public function getCountries(Request $request) {
$id = $request->get('id');
if ( $id ) {
// Or return some string you want to return
return response()->json(Country::find($id));
}
$countries = Country::all();
// or loop over all $countries and make a string
return response()->json($countries);
}
public function getStatesByCountry(Request $request) {
$id = $request->get('country_id');
return response()->json(Country::find($id)->states);
// Or
// return response()->json(State::where('country_id', '=', $id)->get());
}
public function getCitiesByState(Request $request) {
$id = $request->get('state_id');
return response()->json(State::find($id)->municipalities);
// or return response()->json(Municipality::where('state_id', '=', $id)->get());
}
每次更改一個動態選項時,您都要求降低一級。 因此,如果更改國家/地區,則將請求getStatesByCountry
如果狀態更改,則將請求getCitiesByState
。
最后,如果您想要按國家/地區划分所有城市
public function getCitiesByCountry(Request $request) {
$id = $request->get('country_id');
return response()->json(Country::find($id)->municipalities);
}
您可以將這些功能中的每一個放置在控制器中以處理請求。 您還需要更新路由web.php
,並為每個函數添加路由和處理程序。
// {id?} signifies an optional parameter. Based on the func. passing
// no ID gets all Countries - specifying one only gets the one.
Route::get('/posts/get-countries/{id?}', 'PostController@getCountries');
Route::get('/posts/get-states-by-country/{id}', 'PostController@getStatesByCountry');
Route::get('/posts/get-cities-by-state/{id}', 'PostController@getCitiesByState');
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.