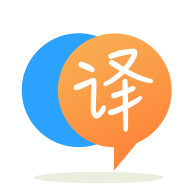
[英]How do I create a dynamic key to be added to a JavaScript object in forEach loop using spread operator
[英]How do I return the object I want by using a key in a forEach loop?
考慮這個代碼:
const year = 1910;
const items = [
{
name: 'gallon of gas',
year: 1910,
price: .12
},
{
name: 'gallon of gas',
year: 1960,
price: .30
},
{
name: 'gallon of gas',
year: 2010,
price: 2.80
}
]
如何顯示與上面定義的年份對應的對象的價格?
items.forEach(d => {
if (d.year === year) {
return d.price;
}
});
^ 為什么那個解決方案不起作用?
無論您在回調函數中返回什么, forEach()
函數都不會返回值。 使用find()
來查找符合您條件的項目:
const year = '1910'; const items = [ { name: 'gallon of gas', year: 1910, price: .12 }, { name: 'gallon of gas', year: 1960, price: .30 }, { name: 'gallon of gas', year: 2010, price: 2.80 } ]; const item = items.find(i => i.year == year); console.log(item.price);
注意:您不能在find()
的回調中使用嚴格比較 ( ===
),因為您將年份字符串與年份數字進行比較。 解決這個問題可能是個好主意。
因為該return
語句位於函數forEach
的處理程序內,所以基本上,您返回的是處理程序執行而不是主函數。
您需要做的是使用 for 循環或函數find
如下:
let found = items.find(d => d.year === year);
if (found) return found.price;
或香草 for 循環:
for (let i = 0; i < items.length; i++)
if (items[i].year === year) return items[i].price;
這需要 ES6 (babel)。 希望這可以幫助! 從https://zellwk.com/blog/looping-through-js-objects/得到它。
const year = 1910; const items = [ { name: 'gallon of gas', year: 1910, price: .12 }, { name: 'gallon of gas', year: 1960, price: .30 }, { name: 'gallon of gas', year: 2010, price: 2.80 } ] const values = Object.values(items) for (const value of values) { //you can't return, but you can use the value or store it in a variable/array console.log(value.price); }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.