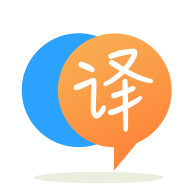
[英]Mongoose findOneAndUpdate doesn't find (or update) in Node
[英]MongoDB - Mongoose query findOneAndUpdate() doesn't update/duplicates the DB
我正在嘗試保存和更新findOneAndUpdate()
( {upsert: true}
-如果該對象不存在,則創建該對象)Web Api的結果,該Web Api包含多個數據數組以填充股票圖表。 每次輸入符號並單擊“獲取報價”按鈕,它都應從Web api獲取數據,然后在數據庫的“子模式”下保存/更新它。 我該如何使用nodejs和mongoose? 這是我嘗試過的代碼。
文件夾-模型-Stock.js
const mongoose = require('mongoose')
mongoose.Promise = global.Promise
mongoose.connect('mongodb://localhost:27017/myapp', { useNewUrlParser: true })
const slug = require('slug')
const childSchemaData = new mongoose.Schema({
date: mongoose.Decimal128,
open: mongoose.Decimal128,
high: mongoose.Decimal128,
low: mongoose.Decimal128,
close: mongoose.Decimal128,
volume: mongoose.Decimal128
})
const parentSchemaSymbol = new mongoose.Schema({
symbol: {
type: String,
trim: true,
minlength: 2,
maxlength: 4,
required: 'Plese enter a valid symbol, min 2 characters and max 4'
},
// Array of subdocuments
data: [childSchemaData],
slug: String
});
//we have to PRE-save slug before save the parentSchemaSymbol into DB
parentSchemaSymbol.pre('save', function (next) {
if (!this.isModified('symbol')) {
next()//skip it
return//stop this function from running
}
this.slug = slug(this.symbol)
next()
//TODO make more resiliant soslug are unique
})
module.exports = mongoose.model('Stock', parentSchemaSymbol)
控制器-webApiController.js
const mongoose = require('mongoose')
const axios = require('axios')
require('../models/Stock')
const parentSchemaSymbol = mongoose.model('Stock')
mongoose.Promise = global.Promise // Tell Mongoose to use ES6 promises
// Connect to our Database and handle any bad connections
mongoose.connect('mongodb://localhost:27017/myapp', { useNewUrlParser: true })
mongoose.connection.on('error', (err) => {
console.error(`🙅 🚫 🙅 🚫 🙅 🚫 🙅 🚫 → ${err.message}`)
})
exports.webApi = (req, res) => {
let curValue = req.params.symbol
axios.get(`https://www.alphavantage.co/query?function=TIME_SERIES_DAILY&symbol=${curValue}&outputsize=compact&apikey=TUVR`)
.then(response => {
return highLow = Object.keys(response.data['Time Series (Daily)']).map(date => {
return {
date: Date.parse(date),
open: Math.round(parseFloat(response.data['Time Series (Daily)'][date]['1. open']) * 100) / 100,
high: Math.round(parseFloat(response.data['Time Series (Daily)'][date]['2. high']) * 100) / 100,
low: Math.round(parseFloat(response.data['Time Series (Daily)'][date]['3. low']) * 100) / 100,
close: Math.round(parseFloat(response.data['Time Series (Daily)'][date]['4. close']) * 100) / 100,
volume: parseInt(response.data['Time Series (Daily)'][date]['5. volume'])
}
})
})
.then(_ => {
let curValueSchema = new parentSchemaSymbol()
curValueSchema.symbol = curValue
highLow.map(item => {
curValueSchema.data.push(item)
})
const query = { symbol: `${curValue.toUpperCase()}` }
const update = { $addToSet: { data: [curValueSchema.data] } }
const options = { upsert: true, new: true }
curValueSchema.findOneAndUpdate(query, update, options).then(doc => {
console.log('Saved the symbol', doc)
return res.send(highLow)
}).catch(e => {
console.log(e)
})
})
.catch(error => {
console.log(error)
})
}
這是我要修復的代碼。 其余的工作:
let curValueSchema = new parentSchemaSymbol()
curValueSchema.symbol = curValue
highLow.map(item => {
curValueSchema.data.push(item)
})
const query = { symbol: `${curValue.toUpperCase()}` }
const update = curValueSchema
const options = { upsert: true, new: true }
curValueSchema.findOneAndUpdate(query, update, options).then(doc => {
console.log('Saved the symbol', doc)
return res.send(highLow)
}).catch(e => {
console.log(e)
})
這是我得到的錯誤
TypeError: curValueSchema.findOneAndUpdate is not a function
at axios.get.then.then._ (/mnt/c/Users/john/Desktop/node/controllers/webApiController.js:55:22)
at process._tickCallback (internal/process/next_tick.js:178:7)
這是數據= highLow
解
因為默認情況下,Mongoose每次傳遞一個Javascript對象來更新文檔字段時,都會默認創建一個新的MongoDB ObjectId(此隱藏的_id字段)。
要遍歷,可以通過確保您的貓鼬模式如下來告訴Mongoose不要創建新的ObjectId:
文件夾-模型-Stock.js
const mongoose = require('mongoose')
mongoose.Promise = global.Promise
mongoose.connect('mongodb://localhost:27017/myapp', { useNewUrlParser: true })
const slug = require('slug')
const childSchemaData = new mongoose.Schema({
"_id": false,
date: mongoose.Decimal128,
open: mongoose.Decimal128,
high: mongoose.Decimal128,
low: mongoose.Decimal128,
close: mongoose.Decimal128,
volume: mongoose.Decimal128
})
const parentSchemaSymbol = new mongoose.Schema({
"_id": false,
symbol: {
type: String,
trim: true,
minlength: 2,
maxlength: 4,
required: 'Plese enter a valid symbol, min 2 characters and max 4'
},
// Array of subdocuments
data: [childSchemaData],
slug: String
});
//we have to PRE-save slug before save the parentSchemaSymbol into DB
parentSchemaSymbol.pre('save', function (next) {
if (!this.isModified('symbol')) {
next()//skip it
return//stop this function from running
}
this.slug = slug(this.symbol)
next()
//TODO make more resiliant soslug are unique
})
module.exports = mongoose.model('Stock', parentSchemaSymbol)
控制器-webApiController.js
let curValueSchema = new parentSchemaSymbol()
curValueSchema.symbol = curValue
highLow.map(item => {
curValueSchema.data.push(item)
})
const query = { symbol: `${curValue.toUpperCase()}` }
const update = curValueSchema
const options = { upsert: true, new: true }
parentSchemaSymbol.findOneAndUpdate(query, update, options).then(doc => {
console.log('Saved the symbol', doc)
return res.send(highLow)
}).catch(e => {
console.log(e)
})
代替:
curValueSchema.findOneAndUpdate
做:
parentSchemaSymbol.findOneAndUpdate
這是模型的方法,而不是實例。 用方案名稱替換實例名稱。
let curValueSchema = new parentSchemaSymbol() curValueSchema.symbol = curValue highLow.map(item = > { curValueSchema.data.push(item) }) const query = { symbol: `$ { curValue.toUpperCase() }` } const update = curValueSchema const options = { upsert: true, new: true } parentSchemaSymbol.findOneAndUpdate(query, update, options).then(doc = > { console.log('Saved the symbol', doc) return res.send(highLow) }). catch (e = > { console.log(e) })
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.