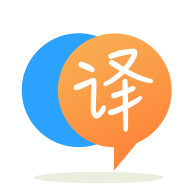
[英]Is it possible to set a bean name using annotations in Spring Framework?
[英]Is it possible in Spring to create beans using annotations if they have properties set to different bean lists?
假設我有A,B,C,D,E類:
@Component
public class A {
}
@Component
public class B {
}
@Component
public class D {
}
@Component
public class E {
}
public class C {
private List myList;
public List getMyList() {
return myList;
}
public void setMyList(List myList) {
this.myList = myList;
}
}
這是我的應用程序上下文:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd">
<util:list id="myList1">
<ref bean="a"/>
<ref bean="b"/>
</util:list>
<util:list id="myList2">
<ref bean="d"/>
<ref bean="e"/>
</util:list>
<bean id="myFirstC" class="com.app.C">
<property name="myList" ref="myList1"></property>
</bean>
<bean id="mySecondC" class="com.app.C">
<property name="myList" ref="myList2"></property>
</bean>
<context:component-scan base-package="com.app"></context:component-scan>
</beans>
是否可以使用C類內部的注釋來創建bean“ myFirstC”和“ mySecondC”? 我試圖自動連接C類中的列表,然后使用@Bean批注創建bean並設置列表,但會出錯。
因此,我嘗試通過這種方式進行操作:
@Component
@Configuration
public class C {
private List myList;
@Autowired
@Qualifier("myList1")
private List myList1;
@Autowired
@Qualifier("myList2")
private List myList2;
@Bean
public C getNewCBean1() {
C c = new C();
c.setMyList(myList1);
return c;
}
@Bean
public C getNewCBean2() {
C c = new C();
c.setMyList(myList2);
return c;
}
但是得到這樣的錯誤:
Exception in thread "main" org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'c': Injection of autowired dependencies failed; nested exception is org.springframework.beans.factory.BeanCreationException: Could not autowire field: private java.util.List com.app.C.myList1; nested exception is org.springframework.beans.FatalBeanException: No element type declared for collection [java.util.List]
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor.postProcessPropertyValues(AutowiredAnnotationBeanPostProcessor.java:288)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.populateBean(AbstractAutowireCapableBeanFactory.java:1116)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.doCreateBean(AbstractAutowireCapableBeanFactory.java:519)
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.createBean(AbstractAutowireCapableBeanFactory.java:458)
at org.springframework.beans.factory.support.AbstractBeanFactory$1.getObject(AbstractBeanFactory.java:295)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.getSingleton(DefaultSingletonBeanRegistry.java:223)
at org.springframework.beans.factory.support.AbstractBeanFactory.doGetBean(AbstractBeanFactory.java:292)
at org.springframework.beans.factory.support.AbstractBeanFactory.getBean(AbstractBeanFactory.java:194)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.preInstantiateSingletons(DefaultListableBeanFactory.java:626)
at org.springframework.context.support.AbstractApplicationContext.finishBeanFactoryInitialization(AbstractApplicationContext.java:932)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:479)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:139)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:83)
at com.app.Start.main(Start.java:9)
Caused by: org.springframework.beans.factory.BeanCreationException: Could not autowire field: private java.util.List com.app.C.myList1; nested exception is org.springframework.beans.FatalBeanException: No element type declared for collection [java.util.List]
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor$AutowiredFieldElement.inject(AutowiredAnnotationBeanPostProcessor.java:514)
at org.springframework.beans.factory.annotation.InjectionMetadata.inject(InjectionMetadata.java:87)
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor.postProcessPropertyValues(AutowiredAnnotationBeanPostProcessor.java:285)
... 13 more
Caused by: org.springframework.beans.FatalBeanException: No element type declared for collection [java.util.List]
at org.springframework.beans.factory.support.DefaultListableBeanFactory.doResolveDependency(DefaultListableBeanFactory.java:807)
at org.springframework.beans.factory.support.DefaultListableBeanFactory.resolveDependency(DefaultListableBeanFactory.java:768)
at org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor$AutowiredFieldElement.inject(AutowiredAnnotationBeanPostProcessor.java:486)
... 15 more
我在文檔和論壇中找不到解決方案。 任何幫助將不勝感激。 謝謝
您的Java代碼與xml代碼根本沒有做相同的事情:
<util:list id="myList1">
<ref bean="a"/>
<ref bean="b"/>
</util:list>
<util:list id="myList2">
<ref bean="d"/>
<ref bean="e"/>
</util:list>
<bean id="myFirstC" class="com.app.C">
<property name="myList" ref="myList1"></property>
</bean>
<bean id="mySecondC" class="com.app.C">
<property name="myList" ref="myList2"></property>
</bean>
這定義了兩個bean myFirstC和mySecondC,分別包含bean a,b和d,e。 因此,在Java中,您需要兩個@Bean
方法來創建相同的C bean:
@Configuration
public class Config {
@Bean
public C myFirstC(A a, B b) {
return new C(Arrays.asList(a, b));
}
@Bean
public C mySecondC(D d, E e) {
return new C(Arrays.asList(d, e));
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.