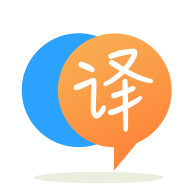
[英]How to consume or call another Rest API in Spring Boot application?
[英]How to consume HornetMQ message in spring boot application
我正在嘗試將HornetMQ Consumer集成到Springboot應用程序中。 我看到了不同的示例,但是所有示例都指向ActiveMQ實現,這使我有些困惑。 我已經用Java編寫了一個標准的HornetQ Consumer。 這是一個代碼:
public class HornetQClient {
private String JMS_QUEUE_NAME;
private String MESSAGE_PROPERTY_NAME;
private ClientSessionFactory sf = null;
private static final Logger LOGGER = LoggerFactory.getLogger(TCPClient.class);
public HornetQClient(String hostName, String hostPort, String queueName, String propertyName) {
try {
Map<String, Object> map = new HashMap<String, Object>();
map.put("host", hostName);
map.put("port", hostPort);
this.JMS_QUEUE_NAME = queueName;
this.MESSAGE_PROPERTY_NAME = propertyName;
ServerLocator serverLocator = HornetQClient.createServerLocatorWithoutHA(new TransportConfiguration(NettyConnectorFactory.class.getName(), map));
sf = serverLocator.createSessionFactory();
startReadMessages();
} catch (Exception e) {
e.printStackTrace();
}
}
private void startReadMessages() {
ClientSession session = null;
try {
if (sf != null) {
session = sf.createSession(true, true);
while (true) {
ClientConsumer messageConsumer = session.createConsumer(JMS_QUEUE_NAME);
session.start();
ClientMessage messageReceived = messageConsumer.receive(1000);
if (messageReceived != null && messageReceived.getStringProperty(MESSAGE_PROPERTY_NAME) != null) {
System.out.println("Received JMS TextMessage:" + messageReceived.getStringProperty(MESSAGE_PROPERTY_NAME));
messageReceived.acknowledge();
} else
System.out.println("no message available");
messageConsumer.close();
Thread.sleep(500);
}
}
} catch (Exception e) {
LOGGER.error("Error while adding message by producer.", e);
} finally {
try {
session.close();
} catch (HornetQException e) {
LOGGER.error("Error while closing producer session,", e);
}
}
}
這個工作正常,但是有什么標准方法可以在Spring Boot應用程序中編寫Message Consumer,或者我應該直接創建此客戶端的Bean並在Springboot應用程序中使用
--------------- hornetq-jms.xml ---------
<?xml version="1.0"?>
<configuration xsi:schemaLocation="urn:hornetq /schema/hornetq-jms.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="urn:hornetq">
<!--the connection factory used by the example -->
<connection-factory name="ConnectionFactory">
<connectors>
<connector-ref connector-name="netty-connector" />
</connectors>
<entries>
<entry name="ConnectionFactory" />
</entries>
<consumer-window-size>0</consumer-window-size>
<connection-ttl>-1</connection-ttl>
</connection-factory>
<queue name="trackerRec">
<entry name="trackerRec" />
</queue>
</configuration>
您可能為此使用Spring JMS和JmsTemplate
。 Spring引導的默認設置是使用ActiveMQ連接工廠,但是如果將其交換為HornetQConnetionFactory,則應該很好:
@Configuration
@EnableJms
public class JmsConfig {
@Bean
public ConnectionFactory connectionFactory() {
final Map<String, Object> properties = new HashMap<>();
properties.put("host", "127.0.0.1");
properties.put("port", "5445");
final org.hornetq.api.core.TransportConfiguration configuration =
new org.hornetq.api.core.TransportConfiguration("org.hornetq.core.remoting.impl.netty.NettyConnectorFactory", properties);
return new org.hornetq.jms.client.HornetQJMSConnectionFactory(false, configuration);
}
@Bean
public JmsListenerContainerFactory<?> myFactory(ConnectionFactory connectionFactory,
DefaultJmsListenerContainerFactoryConfigurer configurer) {
DefaultJmsListenerContainerFactory factory = new DefaultJmsListenerContainerFactory();
configurer.configure(factory, connectionFactory);
return factory;
}
}
為了清楚起見,我添加了全限定類名。
然后,在某些bean中,您可以執行以下操作以消耗一條消息:
@JmsListener(destination = "some.queue", containerFactory = "myFactory")
public void receiveMessage(@Header("some.header") final String something) {
System.out.println("Received <" + something + ">");
}
免責聲明:我實際上並沒有嘗試過此操作,它基於我對Spring和ActiveMQ的經驗以及以下來源: https : //dzone.com/articles/connecting-spring https://spring.io/guides / GS /消息JMS /
您可能需要進行一些挖掘才能使此方法按您想要的方式工作,但是我認為這種方法比您要使用的方法更具“高級”。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.