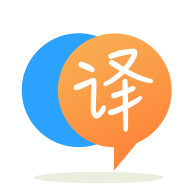
[英]Save State of a radio button in dialog when ok is pressed and dialog is dismissed
[英]JavaFX Dialog: Run onClosingRequest only when Ok button pressed
我有一個表單對話框,我想在對話框關閉之前添加一個確認警報。
在確認 Ok 之前,該對話框不會關閉。 我可以通過消耗事件來防止這種情況。
它應該只在單擊對話框確定時確認,而不是在取消時確認。 問題是,我看不到事件上點擊了哪個按鈕。 因此,對話框上的 OK 和 CANCEL 按鈕都顯示了警報確認。
如何防止取消按鈕的 onClosingReqeust?
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Alert.AlertType;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Dialog;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.StackPane;
import javafx.stage.Modality;
import javafx.stage.Stage;
import javafx.stage.Window;
public class DialogTestApplication extends Application {
public static void main(String[] args) {
launch(args);
}
class MyDialog extends Dialog<ButtonType> {
public MyDialog(Window window) {
setTitle("MyDialog");
initModality(Modality.WINDOW_MODAL);
initOwner(window);
setResizable(true);
GridPane contentPane = new GridPane();
contentPane.add(new TextField(), 0, 0);
getDialogPane().setContent(contentPane);
getDialogPane().getButtonTypes().addAll(ButtonType.OK, ButtonType.CANCEL);
setOnCloseRequest(event -> {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.showAndWait().ifPresent(response -> {
if (response == ButtonType.CANCEL) {
event.consume();
}
});
});
}
}
@Override
public void start(Stage primaryStage) throws Exception {
final StackPane root = new StackPane();
final Label rootLabel = new Label("DialogTestApplication");
root.getChildren().add(rootLabel);
final Scene scene = new Scene(root, 400, 150);
primaryStage.setScene(scene);
primaryStage.setTitle("DialogTestApplication");
primaryStage.show();
Platform.setImplicitExit(true);
MyDialog dialog = new MyDialog(primaryStage);
dialog.showAndWait().ifPresent(response -> {
if (response == ButtonType.OK) {
System.out.println("OK");
}
Platform.exit();
});
}
}
在閱讀了 Dialog Javadoc 之后,我找到了一個有效的解決方案。 https://docs.oracle.com/javase/8/javafx/api/javafx/scene/control/Dialog.html
Button button = (Button) getDialogPane().lookupButton(ButtonType.OK);
button.addEventFilter(ActionEvent.ACTION, event -> {
final Alert alert = new Alert(AlertType.CONFIRMATION);
alert.initOwner(window);
alert.showAndWait().ifPresent(response -> {
if (response == ButtonType.CANCEL) {
event.consume();
}
});
});
final Button btnCancel = (Button) dialog.getDialogPane().lookupButton( ButtonType.CANCEL);
btnCancel.addEventFilter(ActionEvent.ACTION, event -> {
// handle cancel button code here
event.consume();
});
public boolean displayDialog() {
Optional<ButtonType> result = dialog.showAndWait();
if(result.get().getText().equals("Ok")) {
//handle OK action. The dialog panel will close automatically.
}
return true;
}
在實例化警報時處理取消按鈕事件。 event.consume()
方法將阻止警報對話框在按下CANCEL
時關閉。 您可以添加一個方法來顯示您的警報,在該方法中處理 OK 操作。
您可以處理 OK 按鈕的動作事件,而不是處理對話框的關閉請求。 像這樣的東西
// I tested with onMouseClicked but the event doesn't get fired
// onAction on the other hand works normally but we need to cast the node to a button
Button button = (Button) getDialogPane().lookupButton(ButtonType.OK);
button.setOnAction(e -> {
Alert alert = new Alert(AlertType.CONFIRMATION);
alert.showAndWait().ifPresent(response -> {
if (response == ButtonType.CANCEL) {
event.consume();
}
});
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.