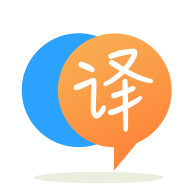
[英]JavaScript String.split(): Split by ; that is NOT preceded by a Backslash?
[英]JavaScript - String.split() but for Arrays?
假設我有這個字符串數組(它們是HTML元素,但是我們可以使用字符串來保持簡單):
["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]
我需要一種快速的方法來用"d"
拆分該數組。 有點像String.split()
,除了數組。 最終結果應該是這樣的:
[["something", "else", "and"], ["more", "things", "in", "the"], ["array", "etc"]]
有沒有簡單的一線工具呢? 也許JS中內置了一個函數,我只是想念它?
一種選擇是用空格連接,然后用' d '
分割,然后用空格分割每個子數組:
const input = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; const output = input .join(' ') .split(' d ') .map(str => str.split(' ')); console.log(output);
或者,不進行聯接,就找出每個d
的索引,然后將輸入的每個部分slice
d
s:
const input = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; const dIndicies = input.reduce((a, item, i) => { if (item === 'd') a.push(i); return a; }, []); const output = dIndicies.reduce((a, dIndex, i, arr) => { const nextDIndex = arr[i + 1]; a.push(input.slice(dIndex + 1, nextDIndex)); return a; }, [input.slice(0, dIndicies[0] - 1)]); console.log(output);
好吧,如果您想要的是單線飛機,那么您就可以開始:
var myArray = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; const result = myArray.reduce((a, c) => c === "d" ? (a.arr[++ai] = []) && a : a.arr[ai].push(c) && a, {arr: [[]], i: 0}).arr; console.log(result);
從具有一個包含空數組的數組的累加器開始使用reduce。 如果當前項目是拆分值,則在末尾添加一個額外的空數組,否則將當前項目散布到最后一個數組中。
const arr = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; const splitArray = (array, val) => array && array.length ? array.reduce( (results, item) => item === val ? [...results, []] : [...results.filter((_, i) => i < results.length - 1), [...results[results.length - 1], item]], [[]] ) : array; console.log(splitArray(arr, 'd'));
let myArray = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; let splitArray = [], tempArray = []; myArray.forEach((ele, index) => { if(ele !== 'd') { tempArray.push(ele); } if(ele === 'd' || index === myArray.length - 1) { splitArray.push(tempArray); tempArray = []; } }) console.log(': ', splitArray);
一個簡單的forEach
方法就足夠了。
var arr = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"]; var result = [], temp = []; arr.forEach(function(elem, index){ elem !=='d' ? temp.push(elem) : (result.push(temp), temp = []); index==arr.length-1 && (result.push(temp)); }); console.log(result)
要回答您的問題,我不會想到任何簡潔的單行代碼,但是您可以通過迭代幾行值以及如果單詞不是'd'
存儲它,只需用幾行代碼即可完成所需的操作; 如果是,則創建一個新數組來保存下一個非“ d”值:
const words = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"] let grouped = words.reduce((response,word)=>{ if (word!=='d') response[response.length-1].push(word) else response[response.length]=[] return response },[[]]) console.log(grouped)
您可以使用以下類似的代碼制作一個非常優雅的遞歸函數:
let arr = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"] const spliton = (v, arr, i = arr.indexOf(v)) => (i < 0) ? [arr] : [arr.slice(0, i), ...spliton(v, arr.slice(i+1))] console.log(spliton('d', arr))
這是適用於任何可迭代輸入(包括數組)的功能編碼
const None = Symbol () const prepend = (xs, x) => [ x ] .concat (xs) const split = (f, [ x = None, ...xs ], then = prepend) => x === None ? then ([], []) : split ( f , xs , (l, r) => f (x) ? then (prepend (l, r), []) : then (l, prepend (r, x)) ) const data = [ 'something', 'else', 'and', 'd', 'more', 'things', 'in', 'the', 'd', 'array', 'etc' ] console .log ( split (x => x === 'd', data) ) // [ [ 'something', 'else', 'and' ] // , [ 'more', 'things', 'in', 'the' ] // , [ 'array', 'etc' ] // ]
優化適用於任何類似數組的輸入
const prepend = (xs, x) =>
[ x ] .concat (xs)
const split = (f, xs = [], i = 0, then = prepend) =>
i >= xs.length
? then ([], [])
: split
( f
, xs
, i + 1
, (l, r) =>
f (xs[i])
? then (prepend (l, r), [])
: then (l, prepend (r, xs[i]))
)
const data =
[ 'something', 'else', 'and', 'd', 'more', 'things', 'in', 'the', 'd', 'array', 'etc' ]
console .log
( split (x => x === 'd', data)
)
// [ [ 'something', 'else', 'and' ]
// , [ 'more', 'things', 'in', 'the' ]
// , [ 'array', 'etc' ]
// ]
兩種實現都是O(n) 。
如果您不關心更改數組,那么使用while
和Array.shift
也是很簡單的:
let r = [[]], data = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"] while(data.length) { let item = data.shift() item != 'd' ? r[r.length-1].push(item) : r.push([]) } console.log(r)
如果這樣做,則使用Array.reduce
可以更短:
let arr = ["something", "else", "and", "d", "more", "things", "in", "the", "d", "array", "etc"] let f = arr.reduce((r,c) => (c!='d' ? r[r.length-1].push(c) : r.push([]),r),[[]]) console.log(f)
兩者的想法都是從[[]]
,然后您唯一需要檢查的是迭代的當前元素是否為d
,如果是,則推入新數組或推入r[r.length-1]
,即前一個sub array
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.