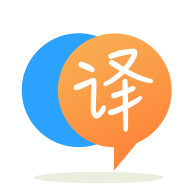
[英]get value from HTML form with Javascript and then display them on html
[英]How to get Javascript to display specific data based on HTML form value
我有一個 html 表單,它有一個包含四個名字的下拉列表。
window.onload = function(){ document.getElementById("submit").onclick = showStudents; } function showStudents(){ if(document.getElementById("mary jones").value == "mary jones"){ document.getElementById("students").innerHTML = "Mary Jones"; } else if(document.getElementById("jane doe").value == "jane doe"){ document.getElementById("students").innerHTML = "Jane Doe"; } else if(document.getElementById("henry miller").value == "henry miller"){ document.getElementById("students").innerHTML = "Henry Miller"; } else if(document.getElementById("john smith").value == "john smith"){ document.getElementById("students").innerHTML = "John Smith"; } }
<div id="students"> <form id="getStudent" action="" method="GET"> <select name="students"> <option value="john smith" id="john smith">John Smith</option> <option value="jane doe" id="jane doe">Jane Doe</option> <option value="henry miller" id="henry miller">Henry Miller</option> <option value="mary jones" id="mary jones">Mary Jones</option> </select> <br><br> <input id="submit" type="submit"> </form>
當我點擊提交時,一個 Javascript 函數被調用,我想顯示我選擇的學生的名字,但它只顯示第一個 if 語句的結果。 我的想法是我需要將表單數據的值傳遞到函數中,但不知道該怎么做。 這是我提出的 javascript 代碼。
您需要使用選定的選項 - 目前,它只會將其設置為“Mary Jones”,因為<option id="mary jones" value="mary jones">
值始終為“瑪麗·瓊斯”。 使用<select>
元素的.value
屬性來獲取所選選項的值:
function showStudents() {
var selected = document.getElementById("getStudent")["students"].value;
var output = document.getElementById("students");
if (selected == "mary jones") {
output.innerHTML = "Mary Jones";
} else if (selected == "jane doe") {
output.innerHTML = "Jane Doe";
} else if (selected == "henry miller") {
output.innerHTML = "Henry Miller";
} else {
output.innerHTML = "John Smith";
}
}
還要記住,ID 名稱中不能有空格 - 所以你的<option>
應該看起來像這樣:
<option value="mary jones" id="maryJones">Mary Jones</option>
您可以從select
的options
獲取選定的option
,然后顯示文本內容。
window.onload = function() { document.getElementById("submit").onclick = showStudents; } function showStudents( e ) { e.preventDefault(); var selectField = document.querySelector("select"); var displayText = selectField.options[ selectField.selectedIndex ].innerText; document.getElementById("students").innerText = displayText; }
<div id="students"></div> <form id="getStudent" action="" method="GET"> <select name="students"> <option value="john smith" id="john smith">John Smith</option> <option value="jane doe" id="jane doe">Jane Doe</option> <option value="henry miller" id="henry miller">Henry Miller</option> <option value="mary jones" id="mary jones">Mary Jones</option> </select> <br><br> <input id="submit" type="submit"> </form>
您的代碼有很多問題:
第一:避免為不同的元素提供重復的 ID。 ID 在所有 HTML 中應該/必須是唯一的。 任何兩個元素都不應具有相同的 ID。 因為如果你這樣做,如果你調用.getElementById()
,你會得到意想不到的結果。
第二:沒有必要為<option>
元素提供 id,除非你真的必須這樣做。 在您的情況下,這樣做沒有任何好處。
第三:即使需要給<option>
元素分配不同的 ID,也要避免 id 字符串中出現空格,因為那樣也會導致不良結果。
第三:如果您只想打印選定的值,則不需要到處都使用 if-then 語句來檢查每個可能的值。 僅當您以不同方式處理每種情況時才使用 if-then。 在您的情況下,switch 語句將使您的代碼更具可讀性和更短。
我對您的代碼進行了一些更改,以反映我上面提到的要點。
window.onload = function() { document.getElementById("submit").onclick = showStudents; } function showStudents() { var n = document.getElementById("sel_students"); var name = n.options[n.selectedIndex].value; var output = document.getElementById("div_students"); output.innerHTML = name; switch (name) { case "mary jones": output.innerHTML += " <br> Hi Mary.."; break; case "jane doe": output.innerHTML += " <br> Good bye Jane"; break; case "henry miller": output.innerHTML += "<br> Good afternoon"; break; case "john smith": output.innerHTML += "<br> no pokahontis here"; break; } }
<div id="div_students"> <form id="form_students" action="" method="GET"> <select name="students" id="sel_students"> <option value="john smith">John Smith</option> <option value="jane doe">Jane Doe</option> <option value="henry miller">Henry Miller</option> <option value="mary jones">Mary Jones</option> </select> <br><br> <input id="submit" type="submit"> </form> </div>
請嘗試這樣做
window.onload = function(){ document.getElementById("submit").onclick = showStudents; } function showStudents(){ document.getElementById("students").innerHTML = document.getElementById("student-list").value; }
<div id="students"> <form id="getStudent" action="" method="GET"> <select name="students" id="student-list"> <option value="john smith" id="john smith">John Smith</option> <option value="jane doe" id="jane doe">Jane Doe</option> <option value="henry miller" id="henry miller">Henry Miller</option> <option value="mary jones" id="mary jones">Mary Jones</option> </select> <br><br> <input id="submit" type="submit"> </form>
你為什么不使用jquery?
通過id
獲取
var value = $('select#dropDownId option:selected').val();
<div id="students">
<form id="getStudent" action="" method="GET">
<select name="students" id="students_value">
<option value="john smith">John Smith</option>
<option value="jane doe">Jane Doe</option>
<option value="henry miller">Henry Miller</option>
<option value="mary jones">Mary Jones</option>
</select>
<br><br>
<input id="submit" type="submit">
</form>
function showStudents(){
var selected = document.getElementById("students_value");
document.getElementById("students").innerHTML = selected;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.