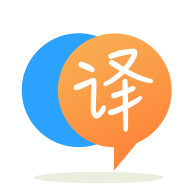
[英]How to get Id of selected value in Mat-Select Option in Angular 5
[英]Angular 7 and angular material how to get the selected option text of mat-select instead of its value
我需要獲取材料下拉列表<mat-select>
的選定文本而不是其值:
<ng-container matColumnDef="fr">
<th mat-header-cell *matHeaderCellDef> Family Rel. </th>
<td mat-cell *matCellDef="let element; let i = index;">
<div [formGroupName]="i">
<mat-form-field color="warn" appearance="outline">
<mat-label>Family Relation</mat-label>
<mat-select #familyRelation (selectionChange)="onChange(element, i, 'hh')" id="family_relation"
formControlName="fr" placeholder="Family Relation">
<mat-option *ngFor="let familyRelation of familyRelationArray;" [value]="familyRelation.family_relation_id">
{{familyRelation.family_relation_type}}
</mat-option>
</mat-select>
</mat-form-field>
</div>
</td>
</ng-container>
這是我正在嘗試做的事情:
@ViewChild('familyRelation') familyRel: ElementRef;
並更改下拉列表的選擇:
onChange(data, i, type) {
let c = this.familyRel.nativeElement.innerText;
console.log(c)
}
我有以下錯誤:
錯誤類型錯誤:無法讀取未定義的屬性“innerText”
當我刪除innerText
時,安慰值是:
不明確的
正如您在stackblitz中看到的那樣,我需要的是,如果我選擇了Parent
,我想讓Parent
成為一個變量而不是1
,這是它的id
。
請注意, (selectionChange)=onChange(element,...)
中的element
、 i
和hh
稍后會在函數中使用,所以現在不要管它。
很抱歉來晚了。 我真的很害怕閱讀上面的所有答案......
該解決方案比任何建議的答案都更容易和直接,因為選擇組件只是將所選模型作為selectionChange
參數的一部分傳遞。
但首先,對您的示例進行一些更正。 你已經聲明了一個接口,所以使用它:
export interface FamilyRelation {
id: number;
type: string;
}
所以,在你的構造函數中:
constructor() {
this.familyRelationArray=[
{
id: 1,
type: 'Parent'
},
{
id: 2,
type: 'Sister'
}
]
}
而不是你在 StackBlitz 中放入的內容......那么你的視圖將變成這樣:
<mat-select (selectionChange)="onChange($event)" id="family_relation" placeholder="Family Relation">
<mat-option *ngFor="let familyRelation of familyRelationArray;" [value]="familyRelation.id">
{{familyRelation.type}}
</mat-option>
</mat-select>
不需要每個 mat-option 的 (click) 處理程序,因為這不是必需的,如果您有很多選項,可能會導致性能問題。 現在,在控制器上:
onChange(ev: any) {
let optionText = ev.source.selected.viewValue;
console.log(optionText);
}
或者,如果您願意,輸入變體:
onChange(ev: MatSelectChange) {
let optionText = (ev.source.selected as MatOption).viewValue; //use .value if you want to get the key of Option
console.log(optionText);
}
但不要忘記進口...
import { MatSelectChange } from '@angular/material/select';
import { MatOption } from '@angular/material/core';
您可以使用 mat-option 添加索引到循環,然后將其傳遞給 onChange() 方法,它將允許您從數組中獲取選定的元素。
<mat-select #familyRelation (selectionChange)="onChange($event.value, element, i, 'hh')" id="family_relation" placeholder="Family Relation">
<mat-option *ngFor="let familyRelation of familyRelationArray; let i=index" [value]="i">
{{familyRelation.family_relation_type}}
</mat-option>
</mat-select>
onChange(index, data, i, type) {
console.log(this.familyRelationArray[index].family_relation_type);
}
這里有更新代碼:鏈接
更新了代碼,並在options
上添加了click
事件
https://stackblitz.com/edit/angular-material-w89kwc?embed=1&file=app/app.component.ts
增加了一項功能
getInnerText(innerText){
console.log(innerText)
}
在視圖中添加點擊事件
<mat-option *ngFor="let familyRelation of familyRelationArray;" [value]="familyRelation.family_relation_id" (click)="getInnerText(familyRelation.family_relation_type)">
{{familyRelation.family_relation_type}}
</mat-option>
使用compareWith
<mat-select #familyRelation [compareWith]="compareFn" id="family_relation" formControlName="fr" placeholder="Family Relation">
<mat-option *ngFor="let familyRelation of familyRelationArray;" [value]="familyRelation.family_relation_id">
{{familyRelation.family_relation_type}}
</mat-option>
</mat-select>
compareFn(data1 , data2){
}
compareWith
監聽 'change' 事件,因為在 Firefox 中不會為選擇觸發 'input' 事件。 文檔。
雖然有點晚了,但答案對我有點啟發,這里有一個想法:
export interface IFamilyGroup{
id: number;
name: string;
address: string;
}
familyGroup: IFamilyGroup[];
getSelectOption( family: IFamilyGroup ): void {
console.log(family);
}
<mat-select (selectionChange)="getSelectOption( familyGroup[$event.value] )">
<mat-option>none</mat-option>
<mat-option
*ngFor="let family of familyGroup; let i = index"
value="{{i}}">
{{ family.name }}
</mat-option>
</mat-select>
這種方法的缺點是不能使用formControlName,因為mat-option是使用index作為value的,所以如果要匹配formgroup,可以改成這樣:
// this is formgroup data model
export interface IFamilyGroup{
id: number;
name: string;
address: string;
}
export interface IFormGroupDataForm{
family: FormControl;
whatever1?: FormControl;
whatever2?: FormControl;
}
dataForm: FormGroup;
family = new FormControl();
familyGroup: IFamilyGroup[];
constructor(
private formBuilder: FormBuilder
) { }
ngOnInit(): void {
this.initFormGroup();
}
private initFormGroup() {
dataForm = this.fromFormGroupModel({
family: this.family
// any you need...
});
}
private fromFormGroupModel( model: IFormGroupDataForm ): FormGroup {
return this.formBuilder.group(model);
}
getSelectOption( family: IFamilyGroup ): void {
this.family.setValue(family); // set family data object be value
}
<div [formGroup]="dataForm">
<mat-select (selectionChange)="getSelectOption( familyGroup[$event.value] )">
<mat-option>none</mat-option>
<mat-option
*ngFor="let family of familyGroup; let i = index"
value="{{i}}">
{{ family.name }}
</mat-option>
</mat-select>
//...
</div>
你可以使用這個方法。
<mat-select [(ngModel)]="job" #jobMatSelect placeholder="-Select Job-" class="form-control" required>
<mat-option *ngFor="let item of Jobs" [value]="item.jobId" [disabled]="item.status == false" [ngClass]="{'col-red':item.status == false}"> ({{item.viewValue}})</mat-option>
</mat-select>
組件.ts
@ViewChild('jobMatSelect') private jobMatSelected: MatSelect;
this.jobSelected = this.jobMatSelected.triggerValue;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.