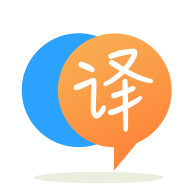
[英]How do I use __str__() and __repr__() for a singly linked list in Python 3.6?
[英]How do I use __str__ method to print list?
class Deck:
def __init__(self):
self.cards=[]
for suit in range(4):
for rank in range(1,14):
card=Card( suit, rank )
self.cards.append(card)
def __str__ (self):
res=[]
for card in self.cards:
res.append(str(card))
return '\n'.join(res)
def pick_card(self):
from random import shuffle
shuffle(self.cards)
return self.cards.pop()
def add_card(self,card):
if isinstance(card, Card): #check if card belongs to card Class!!
self.cards.append(card)
def move_cards(self, gen_hand, num):
for i in range(num):
gen_hand.add_card(self.pick_card())
class Hand(Deck):
def __init__(self, label=''):
self.cards = []
self.label = label
def __str__(self):
return 'The {} is composed by {}'.format(self.label, self.cards)
mazzo_uno = Decks()
hand = Hand('New Hand')
mazzo_uno.move_cards(hand, 5)
print(hand)
我正在嘗試學習面向對象的編程。 當我嘗試從子類Hand()打印對象hand時遇到了這個問題。 我打印了這樣的< main .Card object at 0x10bd9f978>而不是self.cards
list中5張卡的正確字符串名稱:
The New Hand is composed by [<__main__.Card object at 0x10bd9f978>,
<__main__.Card object at 0x10bd9fd30>, <__main__.Card object at 0x10bd9fe80>,
<__main__.Card object at 0x10bcce0b8>, <__main__.Card object at 0x10bd9fac8>]
我也嘗試這樣做來轉換字符串中的self.cards,但是得到了"TypeError: sequence item 0: expected str instance, Card found"
。
def __str__(self):
hand_tostr = ', '.join(self.cards)
return 'The {} is composed by {}'.format(self.label, hand_tostr)
我在該站點上的其他答案上讀到我應該使用__repr__
但是我不知道如何在Hand類中添加它。
__repr__
和__str__
具有不同的用途,但工作方式相同。
您可以閱讀此內容,以幫助您在兩種方法之間進行選擇。
您可以像這樣更改Hand類的__str__
方法:
class Hand:
def __str__(self):
hand_tostr = ', '.join(map(str, self.cards)) # I use map to apply str() to each element of self.cards
return 'The {} is composed by {}'.format(self.label, hand_tostr)
如果要更改Card類的__repr__
方法,可以嘗試這樣的操作(您未提供Card類的代碼)
class Card:
#your code
def __repr__(self):
return <some string>
現在,如果執行str(<list of Card objects>)
,它將在每個卡實例上使用__repr__
方法顯示所需內容。 我不是這種解決方案的忠實擁護者,對於您的情況,我將使用第一個解決方案,因為您可能希望在其他情況下保留卡對象的默認表示形式。
請注意以下代碼:
def add_card(self,card):
if isinstance(card, Card): #check if card belongs to card Class!!
self.cards.append(card)
如果card不是Card的實例,則不會籌集任何資金。 這意味着,如果使用錯誤的參數使用此方法,錯誤將被隱藏,並且您將不知道卡座沒有更改。 這是非常危險的。 您可以改為執行以下操作:
def add_card(self,card):
assert(isinstance(card, Card)), "card parameter of add_card must be an instance of Card class"
self.cards.append(card)
以更Python的方式,您可以使用typehint通知您的類用戶card應該是Card的實例。 然后,相信python的鴨子風格,或者使用mypy之類的工具來驗證該方法是否正確使用。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.