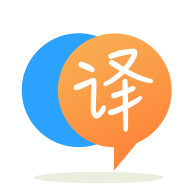
[英]Why is sorting a std::vector of std::tuple's faster than sorting a vector of std::arrays?
[英]Sorting a std::vector of struct's in ascending order
假設我們有一個struct
和一個std::vector
,定義為:
struct example {
int x;
int height;
std::string type;
};
std::vector<example> objects;
首先,我們基於x
值以升序對objects
進行排序,可以很容易地實現它:
std::sort(objects.begin(), objects.end(), [](const example &a, const example &b) {
return a.x < b.x;
});
但是,如果x
值相同,則需要基於其他屬性來處理三個條件。 將以下內容視為我們的對象:
x:0,height:3,type:"start"
x:7,height:11,type:"start"
x:5,height:8,type:"end"
x:1,height:4,type:"start"
x:3,height:6,type:"start"
x:4,height:7,type:"start"
x:1,height:5,type:"start"
x:5,height:9,type:"end"
x:7,height:12,type:"end"
x:6,height:10,type:"start"
插入后,我使用上面編寫的代碼基於x
值對它們進行排序,結果將如下所示:
x:0,height:3,type:"start"
x:1,height:4,type:"start"
x:1,height:5,type:"start"
x:3,height:6,type:"start"
x:4,height:7,type:"start"
x:5,height:8,type:"end"
x:5,height:9,type:"end"
x:6,height:10,type:"start"
x:7,height:11,type:"end"
x:7,height:12,type:"start"
現在,我需要對上述向量進行修改(僅根據x
值對其進行排序。
條件1 :如果x
和type
值相同,並且type
等於start ,那么height
較大的對象必須在前面。
條件2 :如果x
和type
值相同,並且type
等於end ,則height
較小的對象必須位於前面。
條件3 :如果x
的值相同,而type
的值不同,則必須使用具有start type
的對象。
因此,最終排序的向量應如下所示:
x:0,height:3,type:"start"
x:1,height:5,type:"start"
x:1,height:4,type:"start"
x:3,height:6,type:"start"
x:4,height:7,type:"start"
x:5,height:8,type:"end"
x:5,height:9,type:"end"
x:6,height:10,type:"start"
x:7,height:12,type:"start"
x:7,height:11,type:"end"
這些條件如何實現?
不用寫就return ax < bx;
,插入所有條件。 需要注意的重要一點是表達式ax < bx
計算結果為boolean
值:true或false。 如果表達式為true,則第一個元素(在本例中為a)將排在排序數組的首位。
您可以這樣創建條件:
if(a.x == b.x) {
if(a.type.compare(b.type) == 0){ //the types are equal
//condition one
if(a.type.compare("start") == 0) {
return a.height > b.height;
}
//condition two
if(a.type.compare("end") == 0) {
return a.height < b.height;
}
}
else { //types are not equal, condition three
if(a.type.compare("start") == 0) //the type of a is start
return true;
else
return false;
}
}
else {
return a.x < b.x;
}
最好將此代碼放在一個compare函數中:
bool compare(const exampleStruct& a, const exampleStruct& b)
{
//the code written above
}
並將std :: sort稱為:
std::sort(objectVector.begin(), objectVector.end(), compare);
非常簡單。 只需遵循比較器中的邏輯即可:
std::sort(objectVector.begin(), objectVector.end(), [](exampleStruct a, exampleStruct b) {
if (a.x != b.x)
return a.x < b.x;
// a.x == b.x
if (a.type == b.type)
{
if (a.type == "start")
return a.height > b.height;
if (a.type == "end")
return a.height < b.height;
throw std::invalid_argument("invalid type");
}
// a.x == b.x && a.type != b.type
if (a.type == "start")
return true;
if (b.type == "start")
return false;
throw std::invalid_argument("invalid type");
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.