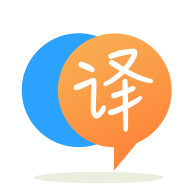
[英]Java - Reflection - How to get @RequestHeader value using reflection
[英]How to get particular Value by Key from JSON request by using Java Reflection
格式化的JSON數據
{
"userId":"123",
"userName":"user",
"age":12
}
從上述JSON請求中,我只想使用Java Reflection提取要存儲在數據庫中的userName
數據。
您可以使用jackson
json解析器。
下面只是一個代碼示例(不能保證它將為您編譯和工作),您應該將其放入接收請求的controler方法中。
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
//put below codes in your request handling method
String jsonStr = req.getReader().lines().collect(Collectors.joining(
System.lineSeparator()));
System.out.println(jsonStr);
JsonNode rootNode = new ObjectMapper().readTree(new StringReader(jsonStr));
JsonNode userName=rootNode.get("userName");
String userNameStr=userName.asText();
// store in data base
您可以參考https://fasterxml.github.io/jackson-databind/javadoc/2.2.0/com/fasterxml/jackson/databind/package-summary.html了解api詳細信息。
您可以使用以下代碼從json數據中獲取用戶名。
JSONObject obj = new JSONObject(YOUR_JSON.toString());
String userName = obj.getString("userName");
有兩種解決方案:
jackson
, gson
, json.org
...並從JsonNode獲取用戶名。 如果您只需要獲取用戶名,則可以使用正則表達式來檢索它。
"userName":"((\\\\"|[^"])+)"
group(1)
是您的用戶。
您可以使用JSON庫解析字符串並檢索值。
基本示例:
import org.json.JSONObject;
public class Main {
public static void main(String[] args) {
String jsonString = "{ \"userId\":\"123\", \"userName\":\"user\", \"age\":12 }";
JSONObject jsonObject = new JSONObject(jsonString);
String userName = jsonObject.getString("userName");
System.out.println("UserName is: " + userName);
}
}
輸出:
UserName is: user
注意:不要忘記在POM文件中添加json依賴關系。
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20160810</version>
</dependency>
盡管有更多的JSON庫可用,例如jackson , google-gson等等。 您可以使用其中任何一個。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.