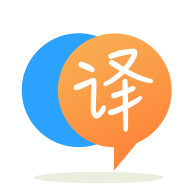
[英]cannot bind non-const lvalue reference of type 'int&' to an rvalue of type 'int'
[英]Error: cannot bind non-const lvalue reference of type ‘int&’ to an rvalue of type ‘int’
我需要創建一個Bar
對象,它有一個私有對象Foo f
。
但是, Foo
對象參數的值應通過特定方法int genValue()
傳遞。
如果我在構造函數范圍Bar(){...}
初始化f
,編譯器會大喊錯誤,就像沒有構造函數Foo()
。
如果我像這樣構造Bar(): f(genValue())
,編譯器會大喊錯誤:
test.cpp: In constructor ‘Bar::Bar()’:
test.cpp:16:19: error: cannot bind non-const lvalue reference of type ‘int&’ to an rvalue of type ‘int’
Bar(): f(genValue()){
~~~~~~~~^~
test.cpp:7:2: note: initializing argument 1 of ‘Foo::Foo(int&)’
Foo(int &x) {
^~~
示例代碼:
class Foo {
public:
Foo(int &x) {
this->x = x;
}
private:
int x;
};
class Bar {
public:
Bar(): f(genValue()){
}
private:
Foo f;
int genValue(){
int x;
// do something ...
x = 1;
return x;
}
};
int main() {
Bar bar ();
return 0;
}
如果我不想修改Foo
類並且它的參數值應該從genValue()
傳遞,我該如何解決這個問題? 而且,我不想使用純指針 (*),但是使用智能指針的解決方案是可以的!
非const
引用參數(例如int&
)只能引用“左值”,即命名變量。
auto takes_nonconst_reference = [](int&){};
auto takes_const_reference = [](const int&){};
auto takes_value = [](int){};
auto returns_int = []{return 42;};
int foo = 1;
// OK
takes_nonconst_reference(foo);
takes_const_reference(foo);
takes_const_reference(returns_int());
takes_value(foo);
takes_value(returns_int());
// compilation error, value returned from a function is not a named variable
takes_nonconst_reference(returns_int());
在這種特殊情況下,由於您的類存儲了構造函數參數的副本,因此您應該按值傳遞它( int
,而不是int&
或const int&
)。
不要傳遞int&
,它不能綁定到常量或臨時變量,因為它們不能被修改 - 改用const int&
。
實際上,對於簡單類型,您應該更喜歡按值傳遞,而讓優化器擔心提供最佳實現。
您的Foo
類型是書面的垃圾。 這會導致您的錯誤。
Foo(int &x) {
this->x = x;
}
這里(a)絕對沒有理由通過引用引用x
,並且(b)更少的理由通過非const
引用引用x
。
以下任何一項均可修復Foo
和您的錯誤。
Foo(int const&x) {
this->x = x;
}
Foo(int const&x_in):x(x_in) {
}
Foo(int x) {
this->x = x;
}
Foo(int x_in):x(x_in) {
}
並且,如果該值實際上不是int
,則很便宜:
Foo(int x) {
this->x = std::move(x);
}
Foo(int x_in):x(std::move(x_in)) {
}
這些是針對您的問題的6種獨立解決方案。
對於int
我將使用#4; 對於非int
#6。
在Foo
之外解決此問題不是一個好主意,因為您因為 Foo
寫錯了而收到錯誤。 您其余的代碼很好,請避免破壞良好的代碼。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.