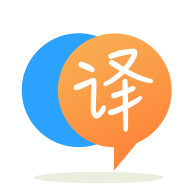
[英]How to get a list of Stomp queues or/and topics (their names) as a client?
[英]How to get all the queues and topics from solace
我想從安慰中發現所有目的地(隊列和主題)
我嘗試使用MBeanServerConnection並在名稱后進行查詢(但我沒有找到使用此名稱的正確方法)或JNDI查找Destination dest =(Destination)context.lookup(Dest_name),但我沒有隊列的名稱/主題。 我正在使用solace-jms庫。
我正在搜索這樣的東西:(但要安慰,而不是activeMq) 從activeMQ獲取所有隊列
為此,您將需要通過管理界面使用SEMP。
示例命令:
curl -d '<rpc><show><queue><name>*</name></queue></show></rpc>' -u semp_username:semp_password http://your_management_ip:your_management_port/SEMP
curl -d '<rpc><show><topic-endpoint><name>*</name></topic-endpoint></show></rpc>' -u semp_username:semp_password http://your_management_ip:your_management_port/SEMP
請注意,為了簡化起見,我使用curl
,但是任何應用程序都可以執行HTTP POST來執行這些命令。 如果使用的是Java,則可以參考Solace API示例中的SempHttpSetRequest
示例。
關於SEMP的文檔可以在這里找到。
但是,這里更大的問題是為什么您需要發現所有目的地?
消息代理的功能之一是使發布者和使用者脫鈎。
如果您需要了解是否將持久消息發布到沒有使用者的主題中,則可以使用發布應用程序的客戶端配置文件中的“拒絕訂閱的味精到發件人”設置。 這意味着,如果發布者嘗試發布有關主題不匹配的訂閱者的消息,則發布者將獲得否定確認。
您可以參考https://docs.solace.com/Configuring-and-Managing/Configuring-Client-Profiles.htm上的“處理不匹配的保證消息”以獲取更多詳細信息。
這是一些可能有用的源代碼。 正確配置設備后,還可以通過JMS在主題“#SEMP /(路由器)/ SHOW”上使用SEMP。
/**
* Return the SolTopicInfo for this topic (or all topics if 'topic' is null).
*
* @param session
* @param endpointName
* @return
*/
public static SolTopicInfo[] getTopicInfo(JCSMPSession session, String endpointName, String vpn,
String sempVersion) {
XMLMessageConsumer cons = null;
XMLMessageProducer prod = null;
Map<String, SolTopicInfo> tiMap = new HashMap<String, SolTopicInfo>();
try {
// Create a producer and a consumer, and connect to appliance.
prod = session.getMessageProducer(new PubCallback());
cons = session.getMessageConsumer(new SubCallback());
cons.start();
if (vpn == null) vpn = (String) session.getProperty(JCSMPProperties.VPN_NAME);
if (sempVersion == null) sempVersion = getSempVersion(session);
// Extract the router name.
final String SEMP_SHOW_TE_TOPICS = "<rpc semp-version=\""
+ sempVersion
+ "\"><show><topic-endpoint><name>"
+ endpointName
+ "</name><vpn-name>"+ vpn + "</vpn-name></topic-endpoint></show></rpc>";
RpcReply teTopics = sendRequest(session, SEMP_SHOW_TE_TOPICS);
for (TopicEndpoint2 te : teTopics.getRpc().getShow().getTopicEndpoint().getTopicEndpoints()
.getTopicEndpointArray()) {
SolTopicInfo ti = new SolTopicInfo();
ti.setBindCount(te.getInfo().getBindCount());
//qi.setDescription(qt.getInfo().getNetworkTopic());
ti.setEndpoint(te.getName());
ti.setMessageVPN(te.getInfo().getMessageVpn());
ti.setTopic(te.getInfo().getDestination());
ti.setDurable(te.getInfo().getDurable());
ti.setInSelPres(te.getInfo().getIngressSelectorPresent());
ti.setHwmMB(formatter.format(te.getInfo().getHighWaterMarkInMb()));
ti.setSpoolUsageMB(formatter.format(te.getInfo().getCurrentSpoolUsageInMb()));
ti.setMessagesSpooled(te.getInfo().getNumMessagesSpooled().longValue());
String status = te.getInfo().getIngressConfigStatus().substring(0, 1).toUpperCase();
status += " " + te.getInfo().getEgressConfigStatus().substring(0, 1).toUpperCase();
status += " " + te.getInfo().getIngressSelectorPresent().substring(0, 1).toUpperCase();
status += " " + te.getInfo().getType().substring(0, 1).toUpperCase();
ti.setStatus(status);
tiMap.put(ti.getEndpoint(), ti);
}
} catch (JCSMPException e) {
throw new RuntimeException(e.getMessage(), e);
} finally {
if (cons != null)
cons.close();
if (prod != null)
prod.close();
}
return tiMap.values().toArray(new SolTopicInfo[0]);
}
/**
* Return the SolQueueInfo for this queue (or all queues if 'queue' is null).
*
* @param session
* @param queue
* @param vpn (if null, use the session's vpn name)
* @param sempVersion, if null use 'soltr/7_1_1'
* @return
*/
public static SolQueueInfo[] getQueueInfo(JCSMPSession session, String queue, String vpn,
String sempVersion) {
XMLMessageConsumer cons = null;
XMLMessageProducer prod = null;
Map<String, SolQueueInfo> qiMap = new HashMap<String, SolQueueInfo>();
try {
// Create a producer and a consumer, and connect to appliance.
prod = session.getMessageProducer(new PubCallback());
cons = session.getMessageConsumer(new SubCallback());
cons.start();
if (vpn == null) vpn = (String) session.getProperty(JCSMPProperties.VPN_NAME);
if (sempVersion == null) sempVersion = getSempVersion(session);
// Extract the router name.
final String SEMP_SHOW_QUEUE_SUBS = "<rpc semp-version=\""
+ sempVersion
+ "\"><show><queue><name>"
+ queue
+ "</name><vpn-name>"+ vpn + "</vpn-name><subscriptions/><count/><num-elements>200</num-elements></queue></show></rpc>";
RpcReply queueSubs = sendRequest(session, SEMP_SHOW_QUEUE_SUBS);
for (QueueType qt : queueSubs.getRpc().getShow().getQueue().getQueues().getQueueArray()) {
SolQueueInfo qi = new SolQueueInfo();
qi.setBindCount(qt.getInfo().getBindCount());
//qi.setDescription(qt.getInfo().getNetworkTopic());
qi.setName(qt.getName());
qi.setMessageVPN(qt.getInfo().getMessageVpn());
qi.setDurable(qt.getInfo().getDurable());
qi.setEgSelPres(qt.getInfo().getEgressSelectorPresent());
qi.setHwmMB(formatter.format(qt.getInfo().getHighWaterMarkInMb()));
qi.setMessagesSpooled(qt.getInfo().getNumMessagesSpooled().longValue());
qi.setSpoolUsageMB(formatter.format(qt.getInfo().getCurrentSpoolUsageInMb()));
String status = qt.getInfo().getIngressConfigStatus().substring(0, 1).toUpperCase();
status += " " + qt.getInfo().getEgressConfigStatus().substring(0, 1).toUpperCase();
status += " " + qt.getInfo().getAccessType().substring(0, 1).toUpperCase();
status += " " + qt.getInfo().getEgressSelectorPresent().substring(0, 1).toUpperCase();
status += " " + qt.getInfo().getType().substring(0, 1).toUpperCase();
status += qt.getInfo().getDurable() ? " D" : " N";
qi.setStatus(status);
for (Subscription sub : qt.getSubscriptions().getSubscriptionArray()) {
qi.addSubscription(sub.getTopic());
}
qiMap.put(qi.getName(), qi);
}
} catch (JCSMPException e) {
throw new RuntimeException(e.getMessage(), e);
} finally {
if (cons != null)
cons.close();
if (prod != null)
prod.close();
}
return qiMap.values().toArray(new SolQueueInfo[0]);
}
private static String getSempVersion(JCSMPSession session)
{
String retval = "soltr/7_1_1";
try {
String peerVersion = (String)session.getCapability(CapabilityType.PEER_SOFTWARE_VERSION);
if (peerVersion != null)
{
retval = "soltr/";
String[] version = peerVersion.split("\\.");
retval += version[0];
retval += "_" + version[1];
if (!version[2].equals("0")) retval += "_" + version[2];
}
} catch (Throwable e) {
System.err.println(e);
}
return retval;
}
private static RpcReply sendRequest(JCSMPSession session,
final String requestStr) {
try {
// Set up the requestor and request message.
String routerName = (String) session
.getCapability(CapabilityType.PEER_ROUTER_NAME);
final String SEMP_TOPIC_STRING = String.format("#SEMP/%s/SHOW",
routerName);
final Topic SEMP_TOPIC = JCSMPFactory.onlyInstance().createTopic(
SEMP_TOPIC_STRING);
Requestor requestor = session.createRequestor();
BytesXMLMessage requestMsg = JCSMPFactory.onlyInstance().createMessage(
BytesXMLMessage.class);
requestMsg.writeAttachment(requestStr.getBytes());
BytesXMLMessage replyMsg = requestor
.request(requestMsg, 5000, SEMP_TOPIC);
String replyStr = new String();
if (replyMsg.getAttachmentContentLength() > 0) {
byte[] bytes = new byte[replyMsg.getAttachmentContentLength()];
replyMsg.readAttachmentBytes(bytes);
replyStr = new String(bytes, "US-ASCII");
}
RpcReplyDocument doc = RpcReplyDocument.Factory.parse(replyStr);
RpcReply reply = doc.getRpcReply();
if (reply.isSetPermissionError()) {
throw new RuntimeException(
"Permission Error: Make sure SEMP over message bus SHOW commands are enabled for this VPN");
}
if( reply.isSetParseError() ) {
throw new RuntimeException( "SEMP Parse Error: " + reply.getParseError() );
}
if( reply.isSetLimitError() ) {
throw new RuntimeException( "SEMP Limit Error: " + reply.getLimitError() );
}
if( reply.isSetExecuteResult() && reply.getExecuteResult().isSetReason() ) { // axelp: encountered this error on invalid 'queue' name
throw new RuntimeException( "SEMP Execution Error: " + reply.getExecuteResult().getReason() );
}
return reply;
} catch (JCSMPException e) {
throw new RuntimeException(e.getMessage(), e);
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e.getMessage(), e);
} catch (XmlException e) {
throw new RuntimeException(e.getMessage(), e);
}
}
您可以使用以下SEMPv2命令獲取特定於消息VPN的隊列和主題。
curl -s -X GET -u semp_user:semp_pass management_host:management_port/SEMP/v2/monitor/msgVpns/{vpn-name}/queues?select="queueName"
curl -s -X GET -u semp_user:semp_pass management_host:management_port/SEMP/v2/monitor/msgVpns/{vpn-name}/topicEndpoints?select="topicEndpointName"
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.