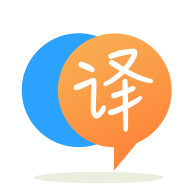
[英]Combine sub-arrays by using as a key a sub-string found in the first element of each sub-array
[英]Replicate Array Of Arrays And Modify Same Element Of Each Sub-Array
我試圖復制數組的數組,然后修改每個子數組的相同元素。
以下代碼用於復制數組的初始數組:
const array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
const n = 2; // replicate twice
let replicated_arrays = [];
for (let i = 0; i < n; i++) {
replicated_arrays.push(array);
}
replicated_arrays = [].concat.apply([], replicated_arrays); // flatten to make one array of arrays
然后,以下代碼用於修改每個數組的第二個元素:
const init = 10;
replicated_arrays.forEach(function(element, index, entireArray) {
entireArray[index][1] = init + index;
});
所需的輸出是:
[[1, 10, 3], [4, 11, 6], [7, 12, 9], [1, 13, 3], [4, 14, 6], [7, 15, 9]]
但是,上面的代碼產生以下內容:
[[1, 13, 3], [4, 14, 6], [7, 15, 9], [1, 13, 3], [4, 14, 6], [7, 15, 9]]
如果復制陣列是手動創建的,則forEach會正確更新:
let replicated_arrays = [[1, 2, 3], [4, 5, 6], [7, 8, 9], [1, 2, 3], [4, 5, 6], [7, 8, 9]];
因此,我懷疑這與push方法有關,該方法創建對初始數組的兩個實例的引用,從而將最終值集(13、14和15)應用於這兩個實例。
作為push方法的替代方法,我嘗試了map方法(例如,按照任意次數重復數組(javascript)的方法 ),但是產生了相同的結果。
任何關於正在進行的事情或如何使其正常工作的見解或建議,將不勝感激。
您需要復制內部數組,因為您需要丟失相同的對象引用。
為了進行推送,您可以展開數組並在以后省略展平。
const array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]; const n = 2; // replicate twice let replicated_arrays = []; for (let i = 0; i < n; i++) { replicated_arrays.push(...array.map(a => a.slice())); // spread array } // no need for this! replicated_arrays = [].concat.apply([], replicated_arrays); const init = 10; replicated_arrays.forEach(function(element, index) { element[1] = init + index; // access element directly without taking the outer array }); console.log(replicated_arrays);
代替concat使用reduce方法將保留相同的引用。
const array = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
const n = 2; // replicate twice
let replicated_arrays = [];
for (let i = 0; i < n; i++) {
replicated_arrays.push(array);
}
replicated_arrays = replicated_arrays.reduce(function(a, b){
return a.concat(b);
}, []);
replicated_arrays.forEach((_ae,i) => {
_ae[1] = 10 + i;
})
console.log(replicated_arrays);
output: [[1, 10, 3], [4, 11, 6], [7, 12, 9], [1, 13, 3], [4, 14, 6], [7, 15, 9]]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.