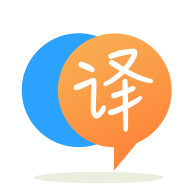
[英]Push nested Json Values with unknown path into Array in Javascript
[英]Push nested JSON values to array
我有一個以下類型的 JSON 數組:
"team": [
{
"paid": {
"refugee": 2018,
"local": 29000,
"international": 12000
}
},
{
"unpaid": {
"refugee": 2019,
"local": 39000,
"international": 19000
}
}
]
我想將匹配鍵的值推送到一個數組中,以便我最終得到以下新數組:
var refugees = [2018, 2019]
var local = [29000, 39000]
var international = [12000, 19000]
等等..
這樣做的簡單方法是什么? 我過去曾為此成功使用過 jQuery,但只需要一個 Javascript 解決方案:
$.each(team, function (i, v) {
var teams = v;
console.log(teams);
$.each(v, function (i, v) {
refugees.push(v.refugee);
local.push(v.local);
international.push(v.international);
});
});
嘗試這個
var a={"team" : [ { "paid": { "refugee": 2018, "local": 29000, "international": 12000 } }, { "unpaid": { "refugee": 2019, "local": 39000, "international": 19000 } } ]} var refugee=[]; var local=[]; var international=[]; a.team.map((e)=>{ if(e.paid) { refugee.push(e.paid.refugee); local.push(e.paid.local); international.push(e.paid.international) } else { refugee.push(e.unpaid.refugee); local.push(e.unpaid.local); international.push(e.unpaid.international) } }) console.log(local) console.log(international) console.log(refugee)
您可以使用reduce。
因此,這里的想法是我們使用一個鍵並將其映射到輸出對象。 我們一直檢查鍵是否已經在輸出對象中,我們將值推入該特定鍵,如果不是,則添加一個帶有value的新屬性。
let obj = {"team":[{"paid":{"refugee":2018,"local":29000,"international":12000}},{"unpaid":{"refugee":2019,"local":39000,"international":19000}}]} let op = obj.team.reduce((output,current)=>{ let temp = Object.values(current)[0] let values = Object.keys(temp) values.forEach(ele=>{ if(output[ele]){ output[ele].push(temp[ele]) } else { output[ele] = [temp[ele]] } }) return output; }, {}) console.log(op)
如果您想要一些單線,類似這樣的事情將起作用:
let local = team.reduce((acc, item) => acc.concat(Object.values(item).map(val => val.local)), []);
let refugee = team.reduce((acc, item) => acc.concat(Object.values(item).map(val => val.refugee)), []);
以最簡單的形式,您可以獲取對象屬性的正確路徑並進行設置。 在您的用例中,很有可能不會那么簡單。 您極有可能需要使用某種方法來找到要尋找的對象,然后在此處設置它的形式。 .find
, .filter
和.includes
是用於在數組中搜索內容的出色工具。
這里還需要注意的一件事是, refugee
和local
的當前價值不是數組格式 。 話雖如此, .push
並不是一個選擇。 您首先需要將屬性值設置為數組,然后可以使用Array.push
const json = {"team": [ { "paid": { "refugee": 2018, "local": 29000, "international": 12000 } }, { "unpaid": { "refugee": 2019, "local": 39000, "international": 19000 } } ]}; json.team[0].paid.refugee = [2018, 2019]; json.team[0].paid.local = [2900, 3900]; console.log(json);
使用Array#reduce,Object#values,Object#entries,擴展語法,解構和Map
const data={"team":[{"paid":{"refugee":2018,"local":29000,"international":12000}},{"unpaid":{"refugee":2019,"local":39000,"international":19000}}]} const res = data.team.reduce((a,c)=>{ Object.values(c) .map(Object.entries) .flat() .forEach(([k,v])=>{ const arr = a.get(k) || []; arr.push(v) a.set(k, arr); }) return a; }, new Map()) //get all console.log([...res.values()]); //get by type console.log(res.get('refugee')); console.log(res.get('local')); console.log(res.get('international'));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.