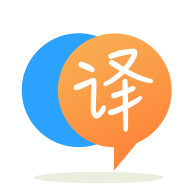
[英]Rainbow Text on an HTML page… each character is a different randomly generated color
[英]Each div another rainbow color
var testDivs = document.querySelectorAll('.test'); var testColors = ['purple', 'blue', 'green', 'orange', 'red']; for (let i = 0; i < testDivs.length; i++) { testDivs[i].style.backgroundColor = testColors[i]; }
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Document</title> </head> <body> <div style="width:50px; height:100px; float:left" class="test"></div> <div style="width:50px; height:100px; float:left" class="test"></div> <div style="width:50px; height:100px; float:left" class="test"></div> <div style="width:50px; height:100px; float:left" class="test"></div> <div style="width:50px; height:100px; float:left" class="test"></div> </body> </html>
querySelectorAll
選擇了它們)。testColors
變量)。testColors
數組中的每個元素分配一種顏色。 如果您有未知的元素數量,那么您可以在選擇它們后對其進行計數,例如: testDivs.length
(本例為 5),然后創建與元素長度一樣多的顏色。
您可以在 html 中設置每個塊的樣式:
.block{ width: 100px; height: 200px; display: inline-block; }
<div class = "block" style = "background-color: purple"></div> <div class = "block" style = "background-color: lightblue"></div> <div class = "block" style = "background-color: lightgreen"></div> <div class = "block" style = "background-color: yellow"></div> <div class = "block" style = "background-color: red"></div>
您可以根據需要添加任意數量的塊。
我將問題分為兩個問題:
首先,您需要獲得一個代碼,當您說出多個元素時,該代碼會創建匹配的顏色。 為了做到這一點,我使用了一個根據波長創建顏色代碼的代碼。 有了這個,您可以輕松地從幾個彩色 div 制作一個彩虹般的網站。 為此,我使用此代碼並使用colorSpace[0]
。
但是要獲得匹配的顏色,我需要計算適當的波長。 可見光為 450nm 至 700nm。 差異是250nm。 所以如果你有三個元素,完美的顏色應該是 450nm + n*250nm/4,n 是顏色(1 到 3)。
執行此操作的正確 Javascript 代碼是
function GetLengths(number) {
var Lengths = [];
for (i = 0; i < number; i++) {
var Number = 450 + (i+1)*250/(number+1)
Lengths.push(Number);
}
return Lengths;
}
第二個問題是讓div得到這些顏色。 這也可以用 Javascript 來實現:
var Divs = document.getElementsByClassName("WithColor");
var Length = 100/Divs.length
for (i = 0; i < Divs.length; i++) {
Divs[i].style.width = Length+"%";
Divs[i].style.background-color = "here a color";
}
現在我們必須連接創建波長的代碼、將波長轉換為顏色代碼的代碼以及將顏色代碼提供給 div 的代碼:
var Divs = document.getElementsByClassName("WithColor"); var TheWaveLengths = GetLengths(Divs.length); var Length = 100/Divs.length for (i = 0; i < Divs.length; i++) { var ColorCode = wavelengthToColor(TheWaveLengths[i])[0]; Divs[i].style.width = Length+"%"; Divs[i].style.background = ColorCode; } function GetLengths(number) { var Lengths = []; for (i = 0; i < number; i++) { var Number = 450 + (i+1)*250/(number+1) Lengths.push(Number); } return Lengths; } function wavelengthToColor(wavelength) { var r, g, b, alpha, colorSpace, wl = wavelength, gamma = 1; if (wl >= 380 && wl < 440) { R = -1 * (wl - 440) / (440 - 380); G = 0; B = 1; } else if (wl >= 440 && wl < 490) { R = 0; G = (wl - 440) / (490 - 440); B = 1; } else if (wl >= 490 && wl < 510) { R = 0; G = 1; B = -1 * (wl - 510) / (510 - 490); } else if (wl >= 510 && wl < 580) { R = (wl - 510) / (580 - 510); G = 1; B = 0; } else if (wl >= 580 && wl < 645) { R = 1; G = -1 * (wl - 645) / (645 - 580); B = 0.0; } else if (wl >= 645 && wl <= 780) { R = 1; G = 0; B = 0; } else { R = 0; G = 0; B = 0; } // intensty is lower at the edges of the visible spectrum. if (wl > 780 || wl < 380) { alpha = 0; } else if (wl > 700) { alpha = (780 - wl) / (780 - 700); } else if (wl < 420) { alpha = (wl - 380) / (420 - 380); } else { alpha = 1; } colorSpace = ["rgba(" + (R * 100) + "%," + (G * 100) + "%," + (B * 100) + "%, " + alpha + ")", R, G, B, alpha] // colorSpace is an array with 5 elements. // The first element is the complete code as a string. // Use colorSpace[0] as is to display the desired color. // use the last four elements alone or together to access each of the individual r, g, b and a channels. return colorSpace; }
.WithColor { height: 100px; display: inline-block; }
<div class="WithColor"></div> <div class="WithColor"></div> <div class="WithColor"></div> <div class="WithColor"></div> <div class="WithColor"></div>
Codes 會自動計算 div 的數量,創建匹配的顏色並調整 div 的長度。 這是一個展示其工作原理的 JSFiddle: https ://jsfiddle.net/Korne127/8hvgqkn2/1
這應該很清楚如何做你所要求的。 選擇你自己的風格。 (我不是設計師。)
<html>
<head><style> #container > div{ height: 40px; width: 200px; border: 3px solid gray; }</style></head>
<body>
<div id="container">
<div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div><div></div>
</div>
<script>
const colorNames = ["darkOrchid", "blue", "limeGreen", "orange", "orangeRed"];
// Assuming all the divs are inside the same containing element
const divNodeList = document.getElementById("container").children;
for(let i = 0; i < divNodeList.length; i++){
let div = divNodeList[i];
let colorIndex = i % colorNames.length; // Modulo operator ("%") let us wrap around
div.style.backgroundColor = colorNames[colorIndex];
};
</script>
</html>
順便說一句,您可以通過粘貼到 html 文件中來查看它的運行情況。 (在 Windows 上的 Chrome 中測試。)
這將選擇任何 div 並創建一個彩虹圖案。
const colors = ["#3d207f","#6399e7","#adce37","#fdcd38","#e87452"] let currentColor = 0 document.querySelectorAll("div").forEach(div => { div.style.backgroundColor = colors[currentColor] currentColor >= colors.length ? currentColor = 0 : currentColor++ })
<div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div> <div>lorem</div>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.