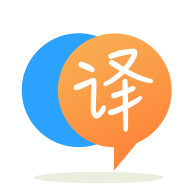
[英]Dominoes program. I can't figure out how to pull my vector into a void to print it out
[英]I can't figure out how to make my program use local variables instead of global variables
我的程序需要使用局部變量而不是全局變量。 但是,當我嘗試執行此操作時,似乎找不到正確的參數來從main和function來回傳遞數據。 我不斷收到錯誤消息,說“ int的參數類型與float類型的參數不兼容”。 請幫助我了解在這里做什么。 感謝您的寶貴時間,我非常感謝。
我試過搜索錯誤代碼,但只找到關於指針問題的答案/問題,而我還沒有學過。 我已經工作了幾個小時,只是為了使變量在“ int main”中工作,但無濟於事。
//This program asks user how many grades there are,
//inputs grades, and displays median of said grades.
//"int main" is at the bottom of the program, preceded by
//variables, function headers, and a single array.
#include <iostream>
using namespace std;
void grdTaker(float [], int);
void sortArray(float[], int);
void median(float[], int);
//Main
int main()
{
//Variables
//int grdsCounted; //Number of grades from user.
const int arraySize = 20;
int grdsCounted; //Number of grades from user.
float grades[arraySize]; //Max grades that can be entered.
grdTaker(grdsCounted, grades[]);
sortArray(grades, grdsCounted);
median(grades, grdsCounted);
system("pause");
}
void grdTaker(float array[], int size) //Function gathers grades.
{
//const int arraySize = 20;
//int grdsCounted; //Number of grades from user.
//float grades[arraySize]; //Max grades that can be entered.
cout << "You may input up to 20 grades. \n";
cout << "First enter the number of grades you have: ";
cin >> grdsCounted;
while (grdsCounted > arraySize)
{
cout << "That is more than 20 grades, try again: \n";
cin >> grdsCounted;
}
cout << "Enter each grade: \n";
//requests how many grades there are and stores them in array
for (int grdCount = 0; grdCount < grdsCounted; grdCount++)
{
cin >> grades[grdCount];
}
};
void sortArray(float array[], int size) //Function sorts array values.
{
bool swap;
float temp;
do
{
swap = false;
for (int count = 0; count < (size - 1); count++)
{
if (array[count] > array[count + 1])
{
temp = array[count];
array[count] = array[count + 1];
array[count + 1] = temp;
swap = true;
}
}
} while (swap);
}
void median(float array[], int size) //Outputs the median of entered grades.
{
int med = size / 2;
int odd = med - 1;
cout << "The median grade is: ";
if (size % 2 == 1)
{
cout << array[med] << endl;
}
else
{
cout << (array[med] + array[odd]) / 2 << endl;
}
}
您分配給浮點數的問題可能是由於在C ++中以這種方式創建了數組。 嘗試使用new
de c ++方式聲明數組。
您是否想過返回值! 看看這個! 例如,將第一個功能划分為2! 編程始終是將問題分解為小問題。
int numberGradesFromUser() {
int grdsCounted;
int arraySize = 20;
cout << "You may input up to 20 grades. \n";
cout << "First enter the number of grades you have: ";
cin >> grdsCounted;
while (grdsCounted > arraySize)
{
cout << "That is more than 20 grades, try again: \n";
cin >> grdsCounted;
}
return grdsCounted;
}
float* grdTaker(int grdsCounted) //Function gathers grades.
{
float * grades = new float[grdsCounted];
cout << "Enter each grade: \n";
//requests how many grades there are and stores them in array
for (int grdCount = 0; grdCount < grdsCounted; grdCount++)
{
cin >> grades[grdCount];
}
return grades;
};
int main()
{
//Variables
int grdsCounted; //Number of grades from user.
grdsCounted = numberGradesFromUser();
float *gradess = new float[grdsCounted];
sortArray(gradess, grdsCounted);
median(gradess, grdsCounted);
system("pause");
}
有了這個,我想其余的功能應該可以工作。 以您的方式調整它們!
同樣,優良作法是在標頭中聲明函數,或者至少在main之上聲明函數,而不是在下面聲明!
試試看:
//This program asks user how many grades there are,
//inputs grades, and displays median of said grades.
//"int main" is at the bottom of the program, preceded by
//variables, function headers, and a single array.
#include <iostream>
using namespace std;
void grdTaker(float [], int, const int);
void sortArray(float[], int);
void median(float[], int);
//Main
int main()
{
//Variables
//int grdsCounted; //Number of grades from user.
const int arraySize = 20;
int grdsCounted; //Number of grades from user.
float grades[arraySize]; //Max grades that can be entered.
grdTaker(grades,arraySize);
sortArray(grades, grdsCounted);
median(grades, grdsCounted);
system("pause");
}
void grdTaker(float array[], const int arraySize) //Function gathers grades.
{
//const int arraySize = 20;
//int grdsCounted; //Number of grades from user.
//float grades[arraySize]; //Max grades that can be entered.
int grdsCounted;
cout << "You may input up to 20 grades. \n";
cout << "First enter the number of grades you have: ";
cin >> grdsCounted;
while (grdsCounted > arraySize)
{
cout << "That is more than 20 grades, try again: \n";
cin >> grdsCounted;
}
cout << "Enter each grade: \n";
//requests how many grades there are and stores them in array
for (int grdCount = 0; grdCount < grdsCounted; grdCount++)
{
cin >> array[grdCount];
}
};
void sortArray(float array[], int size) //Function sorts array values.
{
bool swap;
float temp;
do
{
swap = false;
for (int count = 0; count < (size - 1); count++)
{
if (array[count] > array[count + 1])
{
temp = array[count];
array[count] = array[count + 1];
array[count + 1] = temp;
swap = true;
}
}
} while (swap);
}
void median(float array[], int size) //Outputs the median of entered grades.
{
int med = size / 2;
int odd = med - 1;
cout << "The median grade is: ";
if (size % 2 == 1)
{
cout << array[med] << endl;
}
else
{
cout << (array[med] + array[odd]) / 2 << endl;
}
}
說明: 我添加了ARRAYSIZE到grdTaker功能,並在那里宣布grdsCounted。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.