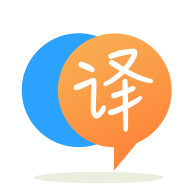
[英]How to assign types of (typeof) all elements in the object to a variable in JavaScript | Typescript?
[英]Typescript typeof value in an object
試圖強類型數據格式化函數。 功能很簡單,
輸入:
const eleOfArray = { a: 1, b: "string", c: [1, 2, 3] }
輸出:
const eleOfArray = { a: { value: 1, component: 1 }, b: { value: "string", component: "string" }, c: { value: [1, 2, 3], component: [1, 2, 3] } }
功能:
export function tableDataFormatter<T>(d: T) { const formatedData: ITableData<T> = {} Object.keys(d).forEach((key: string) => { // tslint:disable-next-line:ban-ts-ignore // @ts-ignore formatedData[key] = { // tslint:disable-next-line:ban-ts-ignore // @ts-ignore value: d[key as keyof T], component: d[key as keyof T] } }) return formatedData }
界面:
interface ITableData<T> { readonly [key: keyof T]: { readonly component: React.ReactNode readonly value: T[keyof T] } }
我在使用此代碼時遇到的問題是,當我使用tableDataFormatter
它顯示value
始終為string | number
string | number
。
用法:
public formatData<IBenefit> (data: ReadonlyArray<IBenefit>): ReadonlyArray<ITableData<IBenefit>> { return super.formatData(data).map((d: ITableData<IBenefit>) => ({ ...d, stores: { ...d.stores, component: <Addon addonValues={d.stores.value} /> // d.stores.value should be an Array but it's being shown as ReactText/string } })) }
所以我必須抑制錯誤,因為函數按預期工作,我清楚地將value
分配為readonly value: T[keyof T]
您的ITableData<T>
接口不是有效的 TypeScript。 具體來說,您正在嘗試使用限制為keyof T
的索引簽名,但唯一允許的索引簽名類型是string
和number
。 相反,您應該考慮使用映射類型而不是接口。 它會給你你想要的映射:
type ITableData<T> = {
readonly [K in keyof T]: {
readonly component: React.ReactNode
readonly value: T[K]
}
}
請注意嵌套value
屬性的類型是T[K]
而不是T[keyof T]
。 T[keyof T]
將是的所有值類型的聯合T
並且從每個鍵的每個值映射丟失。 但是T[K]
意味着對於每個鍵K
,嵌套的value
屬性與由K
索引的T
的原始屬性具有相同的類型。 這是避免string | number
string | number
問題。
然后對於tableDataFormatter()
我會更改一些注釋和斷言,如下所示:
// strip off the readonly modifier for the top level properties
type Mutable<T> = { -readonly [K in keyof T]: T[K] };
// explicitly declare that the function returns ITableData<T>
export function tableDataFormatter<T extends Record<keyof T, React.ReactNode>>(
d: T
): ITableData<T> {
// assert that the return value will be ITableData<T> but make it
// mutable so we can assign to it in the function without error
const formatedData = {} as Mutable<ITableData<T>>;
// assert that Object.keys(d) returns an array of keyof T types
// this is not generally safe but is the same as your "as keyof T"
// assertions and only needs to be done once.
(Object.keys(d) as Array<keyof T>).forEach(key => {
formatedData[key] = {
value: d[key],
component: d[key]
}
})
return formatedData
}
希望它以您現在期望的方式工作。 祝你好運!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.