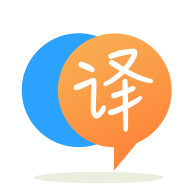
[英]Split a string into an array of words, punctuation and spaces in JavaScript
[英]How to split a string without spaces into an array of words?
所以我在freecodecamp.org上通過編寫電話檢查器算法來練習javascript。 當僅提供的電話號碼是一串數字時,我成功檢查了它。 現在,我被卡住了,並且不知道如何檢查提供的電話號碼中是否包含諸如“ sixnineone”之類的單詞。 因此,我想將其拆分為“六個九個一”或將其與數字對象數組轉換為“ 691”。
這是問題所在:
我試圖通過該網站獲得一些提示,但它們只能使用我不太理解的正則表達式解決問題。
這是我所做的:
function telephoneCheck(str) {
let phoneNum = str.toLowerCase().replace(/[^1-9a-z]/g, "");
let numbers = [
{0: "o"},
{1: "one"},
{2: "two"},
{3: "tree"},
{4: "four"},
{5: "five"},
{6: "six"},
{7: "seven"},
{8: "eight"},
{9: "nine"}
];
if (phoneNum.match(/[1-9]/)) {
phoneNum = phoneNum.split('')
if (phoneNum.length === 10) {
phoneNum.unshift(1);
}
for (let i = 0; i < phoneNum.length; i++) {
phoneNum[i] = Number(phoneNum[i]);
}
if (phoneNum.length === 11 && phoneNum[0] === 1) {
return true;
} else {
return false;
}
}
if (phoneNum.match(/[a-z]/)) {
console.log(phoneNum);
}
}
console.log(telephoneCheck("sixone"));
在解決問題時,據說唯一的解決方案就是他們的解決方案,但是如果我認為正確,那么可能會有另一種解決方案。
一種方法是使用Map,然后將名稱用作鍵,將值用作數字。
然后從地圖中提取鍵,對鍵進行排序,以使最長的字符串排在最前面,並創建一個帶有捕獲組和交替字符的正則表達式
正則表達式最終看起來像:
(three|seven|eight|four|five|nine|one|two|six|o)
然后使用此正則表達式拆分字符串。 當映射不包含鍵時,映射所有項以除去所有非數字,並從數組中除去所有空值。
最后,使用鍵從地圖中獲取值。
let map = new Map([ ["o", 0], ["one", 1], ["two", 2], ["three", 3], ["four", 4], ["five", 5], ["six", 6], ["seven", 7], ["eight", 8], ["nine", 9] ]); let regex = new RegExp("(" + [...map.keys()] .sort((a, b) => b.length - a.length) .join('|') + ")"); let strings = [ "69ooooneotwonine", "o", "testninetest", "10001", "7xxxxxxx6fivetimesfifefofourt", "test" ].map(s => s.split(regex) .map(x => !map.has(x) ? x.replace(/\\D+/, '') : x) .filter(Boolean) .map(x => map.has(x) ? map.get(x) : x) .join('')); console.log(strings);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.