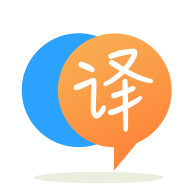
[英]How to parse and print a matching search in a line of text, plus the next 2 lines after a match in Python
[英]Python: how to parse the next line?
我對Python沒有經驗,因此我提出了幫助我改善代碼的要求。
我正在嘗試解析“名稱”下的“史蒂夫”:
xxxx xxxx xxxx Name
zzzz zzzz zzzz Steve
我的代碼如下所示:
for line in myfile.readlines():
[..]
if re.search(r'Name =', line):
print("Destination = ")
samples+=line[15:19]
nextline = "y"
if nextline == 'y':
samples+=line[15:19]
最終,我將打印所有內容:
[..]
for s in samples:
myfile2.write(s)
它確實有效,但是我不敢相信沒有更聰明的方法可以做到這一點(例如,一旦滿足條件,就可以訪問下一行)。
這是我需要解析的文件的示例。 但是結構可能會有所不同,例如
#This is another example
Name =
Steve
任何幫助表示贊賞。
LIST.TXT:
zzzz zzzz zzzz Abcde
xxxx xxxx xxxx Name
zzzz zzzz zzzz Steve
zzzz zzzz zzzz Efghs
接着:
logFile = "list.txt"
with open(logFile) as f:
content = f.readlines()
# you may also want to remove empty lines
content = [l.strip() for l in content if l.strip()]
# flag for next line
nextLine = False
for line in content:
find_Name = line.find('Name') # check if Name exists in the line
if find_Name > 0 # If Name exists, set the next_line flag
nextLine = not nextLine
else:
if nextLine: # If the flag is set, grab the Name
print(line.split(" ")[-1]) # Grabbing the last word of the line
nextLine = not nextLine
OUTPUT:
Steve
不要重新發明輪子。 使用csv
模塊,例如與DictReader
一起使用:
import csv
with open("input") as f:
reader = csv.DictReader(f, delimiter=" ")
for line in reader:
print(line["Name"])
假設“史蒂夫”並不總是在字面上“名稱”之下,因為如果其他列中的項目更長或更短,而同一列中的項目更長或更短,則位置可能會有所不同。 此外,這還假定帶有"Name"
行將是文件中的第一行。
如果不是這種情況,並且Name
可以出現在任何行中,並且您只希望在該行下面的名稱,則可以僅在for
循環所使用的同一迭代器上調用next
:
import re
with open("input") as f:
for line in f: # note: no readlines!
if re.search(r'\bName\b', line): # \b == word boundary
pos = line.split().index("Name")
name = next(f).split()[pos]
print(name)
LIST.TXT:
zzzz zzzz zzzz Abcde
xxxx xxxx xxxx Name
zzzz zzzz zzzz Steve
zzzz zzzz zzzz Efghs
如下例所示:
logFile = "list.txt"
with open(logFile) as f:
lines = f.readlines()
for line in lines:
# split using space
result = line.split(" ")
# you can access the name directly:
# name = line.split(" ")[3]
# python array starts at 0
# so by using [3], you access the 4th column.
print result[3]
或者,您可以使用numpy從數據字典中僅打印第4列:
import numpy
logFile = "list.txt"
data = []
with open(logFile) as f:
lines = f.readlines()
for line in lines:
result = line.split(" ")
data.append(result)
matrix = numpy.matrix(data)
print matrix[:,[3]]
您可以在此處閱讀有關此內容的更多信息: StackOverflow問題 一些矩陣信息
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.