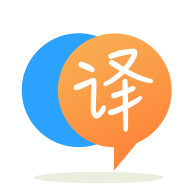
[英]return “even” if others numbers are odd and “odd” the others number are even javascript
[英]How best to determine the digit to the left of the rigthmost number for numbers with odd and even length?
我正在編寫代碼來檢查一定數量的信用卡號輸入是否有效。 我已經寫了:
function validCreditCard(value) {
// accept only digits, dashes or spaces
if (value.trim().length <= 1) return 'Value entered must be greater than 1';
if (/[^0-9\s]+/.test(value)) return false;
// Remove all white spaces
value = value.replace(/\D/g, '');
for (var i = 0; i < value.length; i++) {
// code goes here
// Loop through the string from the rightmost moving left and double the value of every second digit.
}
由於字符串的長度可以是偶數或奇數,因此我一直試圖圍繞如何從最右端向左循環遍歷字符串並使第二個數字的值加倍 。 例如,對於長度為16(偶數)的輸入,左邊的第一個數字將是第15個位置(索引14),對於長度為11的奇數輸入,左邊的第一個數字將是第10個位置(索引9)。 我編寫的程序在兩種情況下均不起作用,現在我想編寫一種程序來解決這兩種情況。 如何最好地做到這一點,而無需創建兩個單獨的檢查來查看輸入的長度是奇數還是偶數?
PS:某些在線實施對兩種情況也不起作用。
要知道哪個是“右數第二個數字”,您實際上並不需要從頭到尾進行迭代。 也可以從左到右完成。 要知道某個數字是否是這樣的“第二”數字,請將其奇/偶奇偶校驗與輸入長度的奇偶校驗相比較。
像這樣:
var parity = value.length % 2;
for (var i = 0; i < value.length; i++) {
if (i % 2 === parity) {
// "Special" treatment comes here
} else {
// "Normal" treatment comes here
}
}
但是,如果輸入的長度為奇數,也可以在輸入之前加上零,以使其為偶數長度:
if (value.length % 2) value = '0' + value;
for (var i = 0; i < value.length; i++) {
if (i % 2 === 0) {
// "Special" treatment comes here
} else {
// "Normal" treatment comes here
}
}
您要實現的稱為Luhn算法 。 因此,使用長度的奇偶校驗,它看起來可能像這樣:
function validCreditCard(value) { // Accept only digits, dashes or spaces if (/[^0-9\\s-]+/.test(value)) return false; // Get the digits only value = value.replace(/\\D/g, ""); // Need at least one digit if (!value.length) return false; var parity = value.length % 2; var sum = 0; for (var i = 0; i < value.length; i++) { sum += i % 2 === parity ? (value[i]*2) % 10 + (value[i] > '4') // Double, and add one if double > 9 : +value[i]; // Normal case } return sum%10 === 0; } console.log(validCreditCard("4024007112651582"));
在循環中,我用條件三元運算符 -替換了if...else...
構造... ? ... : ...
... ? ... : ...
在兩種情況下都需要為變量分配值(在我們的情況下sum
)時,這是一種實用的方法。
對於“普通”數字, sum
必須隨數字的值( +value[i]
)增加。 加一元運算符會將字符轉換為其數值-因此+'1'
變為1。
對於“特殊”數字, sum
必須以該數字的值( value[i]*2
)的兩倍增加。 請注意,由於乘法運算,從字符串到整數的轉換是自動發生的。
然后,我們需要處理這種雙精度值由兩位數字組成的情況。 例如:8 * 2 =16。在這種情況下,所得數字不應為6,而應為7。因此我們加上(value[i] > '4')
。 那確實是布爾表達式( false
或true
):如果double有兩位數字,則為true。 通過添加布爾值,將其分別強制為0或1-正是我們所需要的。
從最右端向左循環遍歷字符串,並將第二個數字的值翻倍
您可以使用.reverse()
.map()
和余數運算符%
let arr = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16]; var res = [...arr].reverse().map((a, i) => i % 2 ? a * 2 : a).reverse(); console.log(res);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.