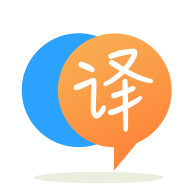
[英]How to calculate the number of available paths from point A to B using recursion
[英]How to use recursion to find and print multiple shortest paths from point A to point B? (C++)
第一次在這里發帖,如果我做錯了什么,請原諒我。 我無法弄清楚如何遞歸地找到並打印從 A 點到 B 點的所有最短路徑。
我正在制作一個程序,讓機器人使用盡可能最短的路徑從 A 點到 B 點。 該程序必須使用遞歸並找到並打印機器人從 A 點到 B 點可以采取的所有最短路徑。 例如,如果機器人從 (1, 2) 點開始並需要前往該點(3, 5),那么程序應該打印 10 條路徑,只有 5 次移動(例如 NNNEE)。 這里是最重要的兩個函數,抱歉代碼太亂了。
void Robot::FindPath(const Board &board)
{
string path = "";
vector < string > pathsVector;
MoveRobot(startingX,
startingY,
treasureX,
treasureY,
path,
"",
0,
pathsVector);
}
void Robot::MoveRobot(const int &startingX,
const int &startingY,
const int &treasureX,
const int &treasureY,
const string &path,
const string &lastMove,
const int &numMovesDirection,
vector<string> &pathVector)
{
if (startingX == treasureX && startingY == treasureY)
{
cout << path << endl;
PathCount++;
pathVector.push_back(path);
return;
}
else if (startingY == treasureY)
{
if (startingX - treasureX > 0)
{
if (lastMove == "W" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "W" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX - 1,
startingY,
treasureX,
treasureY,
path + "W",
"W",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "W")
{
MoveRobot(startingX - 1,
startingY,
treasureX,
treasureY,
path + "W",
"W",
1,
pathVector);
}
}
else if (startingX - treasureX < 0)
{
if (lastMove == "E" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "E" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX + 1,
startingY,
treasureX,
treasureY,
path + "E",
"E",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "E")
{
MoveRobot(startingX + 1,
startingY,
treasureX,
treasureY,
path + "E",
"E",
1,
pathVector);
}
}
}
else if (startingX == treasureX)
{
if (startingY - treasureY > 0)
{
if (lastMove == "S" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "S" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX,
startingY - 1,
treasureX,
treasureY,
path + "S",
"S",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "S")
{
MoveRobot(startingX,
startingY - 1,
treasureX,
treasureY,
path + "S",
"S",
1,
pathVector);
}
}
else if (startingY + treasureY > 0)
{
if (lastMove == "N" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "N" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX,
startingY + 1,
treasureX,
treasureY,
path + "N",
"N",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "N")
{
MoveRobot(startingX,
startingY + 1,
treasureX,
treasureY,
path + "N",
"N",
1,
pathVector);
}
}
}
else
{
if (startingX - treasureX > 0)
{
if (lastMove == "W" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "W" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX - 1,
startingY,
treasureX,
treasureY,
path + "W",
"W",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "W")
{
MoveRobot(startingX - 1,
startingY,
treasureX,
treasureY,
path + "W",
"W",
1,
pathVector);
}
}
else if (startingX - treasureX < 0)
{
if (lastMove == "E" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "E" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX + 1,
startingY,
treasureX,
treasureY,
path + "E",
"E",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "E")
{
MoveRobot(startingX + 1,
startingY,
treasureX,
treasureY,
path + "E",
"E",
1,
pathVector);
}
}
else if (startingY - treasureY > 0)
{
if (lastMove == "S" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "S" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX,
startingY - 1,
treasureX,
treasureY,
path + "S",
"S",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "S")
{
MoveRobot(startingX,
startingY - 1,
treasureX,
treasureY,
path + "S",
"S",
1,
pathVector);
}
}
else if (startingY + treasureY > 0)
{
if (lastMove == "N" && numMovesDirection == MaxStepsDir)
{
}
else if (lastMove == "N" &&
(numMovesDirection < MaxStepsDir ||
numMovesDirection > MaxStepsDir))
{
MoveRobot(startingX,
startingY + 1,
treasureX,
treasureY,
path + "N",
"N",
numMovesDirection + 1,
pathVector);
}
else if (lastMove != "N")
{
MoveRobot(startingX,
startingY + 1,
treasureX,
treasureY,
path + "N",
"N",
1,
pathVector);
}
}
}
}
我知道第一個 if 語句中的“返回”正在停止遞歸,但我不知道當我給出的示例中的所有 10 條最短路徑都被找到並打印時,我會在哪里結束遞歸。 因為它只打印 EENNN。 請忽略“if (lastMove == "W" && numMovesDirection == MaxStepsDir)”的 if 語句,我計划稍后填寫這些語句以使程序的不同部分工作。 非常感謝您對此的任何幫助!
我自己想出來的。 對不起,如果我浪費了任何人的時間。 我對遞歸有一個基本的誤解,也有代碼中的一個小錯誤。 我沒有意識到遞歸會通過回溯和沿着其他路徑自動打印所有最短路徑。 我的代碼中阻止這種情況發生的錯誤是在我的代碼末尾附近的大“else”中,我不小心輸入了“else if (startingY - TreasureY > 0)”。 這應該只是說“if (startingY - TreasureY > 0)”,因為那個大的 else 代碼塊的重點是改變 x 坐標和 y 坐標,如果它們都沒有在正確的位置,並把 else 如果有阻止那。 一旦將 else if 更改為 if,它會打印出我給出的示例的所有十條最短路徑。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.