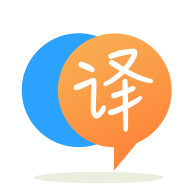
[英]Python Ping-Pong game, the ball speed randomly changes during paddle movement
[英]Python ping-pong game, speeding up the ball after paddle hit
我是新來的,我是初學者。 我從5個月開始學習編程,作為主要語言,我決定堅持使用python。 我寫了我的第一個簡單的游戲是ping-pong(基於yt教程),我想通過在每次擊球槳后加快球速並在得分時恢復到正常速度來對它進行一些改進。 我知道我把ball.dx + = 1放在錯誤的位置。 它可以加快速度,但是只有在球上升時才加速。 當它下降時,它會恢復正常速度,並且速度當然會循環,即使被計分,它仍然會加速。 感謝任何幫助。
import turtle
wn = turtle.Screen()
wn.title("gra by mati")
wn.bgcolor("black")
wn.setup(width=800, height=600)
wn.tracer(0)
# Paddle A
paddle_a = turtle.Turtle()
paddle_a.shape("square")
paddle_a.shapesize(stretch_wid=5, stretch_len=1)
paddle_a.speed(0)
paddle_a.color("white")
paddle_a.penup()
paddle_a.goto(-350, 0)
# Paddle B
paddle_b = turtle.Turtle()
paddle_b.shape("square")
paddle_b.shapesize(stretch_wid=5, stretch_len=1)
paddle_b.speed(0)
paddle_b.color("white")
paddle_b.penup()
paddle_b.goto(350, 0)
# ball
ball = turtle.Turtle()
ball.shape("square")
ball.speed(0)
ball.color("white")
ball.penup()
ball.goto(0, 0)
ball.dx = 1/5
ball.dy = 1/5
# Pen
pen = turtle.Turtle()
pen.speed(0)
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 260)
pen.write("Player A: 0 Player B: 0", align="center", font=("Courier", 24, "normal"))
# Score
score_a = 0
score_b = 0
# Function
def paddle_a_up():
y = paddle_a.ycor()
y += 20
paddle_a.sety(y)
def paddle_a_down():
y = paddle_a.ycor()
y -= 20
paddle_a.sety(y)
def paddle_b_up():
y = paddle_b.ycor()
y += 20
paddle_b.sety(y)
def paddle_b_down():
y = paddle_b.ycor()
y -= 20
paddle_b.sety(y)
#keyboard biding
wn.listen()
wn.onkeypress(paddle_a_up, "w")
wn.onkeypress(paddle_a_down, "s")
wn.onkeypress(paddle_b_up, "Up")
wn.onkeypress(paddle_b_down, "Down")
# Main game loop
while True:
wn.update()
# move the ball
ball.setx(ball.xcor() + ball.dx)
ball.sety(ball.ycor() + ball.dy)
# border checking
if ball.ycor() > 290:
ball.sety(290)
ball.dy *= -1
if ball.ycor() < -290:
ball.sety(-290)
ball.dy *= -1
if ball.xcor() > 390:
ball.goto(0, 0)
ball.dx *= -1
score_a += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
if ball.xcor() < -390:
ball.goto(0, 0)
ball.dx *= -1
score_b += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
# paddle and ball collision
if (ball.xcor() > 340 and ball.xcor() < 350) and (ball.ycor() < paddle_b.ycor() + 50 and ball.ycor() > paddle_b.ycor() -40):
ball.setx(340)
ball.dx +=1
ball.dx *= -1
if (ball.xcor() < -340 and ball.xcor() > -350) and (ball.ycor() < paddle_a.ycor() + 50 and ball.ycor() > paddle_a.ycor() -40):
ball.setx(-340)
ball.dx +=1
ball.dx *= -1
因此,在回顧了一下游戲后,我發現一個錯誤,球會“粘在”左側的球拍上,就像您說的那樣,速度並沒有重置每個得分。 我已經在幾個地方進行了調整,它似乎有了很大的改善。
首先,每次得分時我都會重置速度:
if ball.xcor() > 390:
ball.goto(0, 0)
ball.dx = 1 / 5 # reset to initial speed
score_a += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
if ball.xcor() < -390:
ball.goto(0, 0)
ball.dx = 1 / 5 # reset to initial speed
ball.dx *= -1
score_b += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
我認為可以通過在頂部設置一個變量(如initial_speed = 1/5
來改善此狀況,然后可以將ball.dx = initial_speed
放到重置的任何位置。 這樣一來,您僅需在程序頂部更改一個數字,即可在任何地方調整速度,從而不必每次重新設置時都必須追逐速度!
其次,我固定了槳碰撞
if (ball.xcor() > 340 and ball.xcor() < 350) and (ball.ycor() < paddle_b.ycor() + 50 and ball.ycor() > paddle_b.ycor() -40):
ball.setx(340)
ball.dx *= -1 # flip direction first
ball.dx -=1 # speed it up
if (ball.xcor() < -340 and ball.xcor() > -350) and (ball.ycor() < paddle_a.ycor() + 50 and ball.ycor() > paddle_a.ycor() -40):
ball.setx(-340)
ball.dx *= -1
ball.dx +=1
同樣,可以通過使變量speed_up_by = 1
,然后使用ball.dx += speed_up_by
輕松地進行調整來改善這一點。 我認為1太大了,如果您很幸運,您將無法獲得超過5個匹配。 為了回答您對為什么一個是ball.dx +=1
而另一個是ball.dx -=1
我敦促您考慮一下數字。 初始速度為.2(或1/5),然后在其達到右側后應將其反轉(至-.2,現在它向左移動),然后提高其速度(通過減1->- 1.2)。 現在,當它到達另一側時,再次將其反轉(至1.2),然后提高其速度(通過添加1-> 2.2)。 您可以看到,即使我們從速度中減去,實際上也會增加速度的大小! 我認為這也向您表明,將每個命中速度提高1真是太瘋狂了。 第一次擊球后,球的運動速度是初始速度的6倍 !!! 2次命中后,它的速度是初始速度的11倍 !!! 您可能希望每次將速度的大小增加.1。 這樣一來,擊打后的速度僅為初始速度的1.5倍。 2次命中后,速度是初始速度的兩倍……依此類推。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.