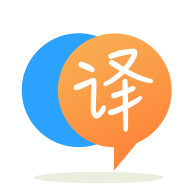
[英]How do i click an element in a div and get the text content of that element which is derived from an object in array which was looped to the DOM
[英]React Native: In an array of Text Inputs which are looped then displayed, how to get nth element and modify those elements separately?
在這個模塊中,我試圖創建一個類似於twitter中的調查模塊。
首先,文本輸入邊框的顏色是灰色,當我聚焦(單擊)文本輸入時,只有其中一個(單擊一個)必須是藍色。 當我鍵入文本時,想法相同,它們都不應該獲得相同的值。 通過單擊加號圖標,我應該能夠獲得我創建的每個文本輸入值(字符串)
我應該使用平面列表還是列表視圖,而不是for循環? React-Native Listview,按行並更改該行樣式,我也嘗試根據此示例解決它。 我稍微改變了這個例子,我能夠改變被點擊的邊框的顏色。 但是我仍然無法獲得這些價值...
有解決方案的建議嗎? 謝謝。
這是我的代碼;
changeInputBorderColor = () => {
const newinputBorderColor = cloneDeep(this.state.inputBorderColor);
newinputBorderColor.bar = '#04A5F5';
this.setState({inputBorderColor: {bar: newinputBorderColor.bar}});
};
changeInputBorderColor2 = () => {
this.setState({
inputBorderColor: {
bar: 'grey'
}
})
};
incrementInputCount = () => {
if (this.state.inputCounter < 5) {
this.setState(prevState => {
return {inputCounter: prevState.inputCounter + 1}
});
console.log(this.state.inputCounter);
}
else {
this.setState(prevState => {
return {inputCounter: prevState.inputCounter}
});
alert("Maximum soru sayısına ulaştınız");
}
};
render() {
let surveyOptions = [];
for (let i = 0; i < this.state.inputCounter; i++) {
console.log(this.state.inputCounter);
surveyOptions.push(
<View key={i}>
<View>
<TextInput
style={[styles._surveyTextInput, {borderColor: this.state.inputBorderColor.bar}]}
onChangeText={(text) => this.setState({text})}
value={this.state.text}
onFocus={this.changeInputBorderColor}
onBlur={this.changeInputBorderColor2}
placeholder={"Secenek " + (i + 1)}
/>
</View>
</View>
)
}
return (
<View style={styles._surveyMainContainer}>
<View style={{flex: 0.8}}>
{surveyOptions}
<TouchableOpacity style={{position: 'absolute', right: 5, top: 5}}>
<Ionicons name={"ios-close-circle"}
size={30}
color={'black'}
/>
</TouchableOpacity>
<TouchableOpacity style={{position: 'absolute', right: 5, top: 45}}
onPress={this.incrementInputCount}>
<Ionicons name={"ios-add-circle"}
size={30}
color={'blue'}
/>
</TouchableOpacity>
</View>
<View style={{flex: 0.2}}>
<View
style={styles.renderSeparator}
/>
<Text style={{fontWeight: 'bold', margin: 5}}>Anket süresi</Text>
</View>
</View>
);
}
您可以使用.map
進行此操作,但是必須正確設置它,以便每個TextInput
都具有自己的狀態值。 當前,您正在為每個TextInput
設置相同的狀態值,這將導致每個TextInput
具有相同的值。 顯然不是您想要的。
textArray
)中創建一個初始數組,該數組的所有值均為空字符串,這將用於存儲每個TextInput
的值。 focusedIndex
設置為null TextInput
索引與當前focusedIndex
進行比較 textArray
並創建TextInput
組件。 確保每個TextInput
都有自己的狀態值。 onFocus
和TextInput
的onFocus
和onBlur
中設置focusedIndex的值。 當它模糊時,我們應該將該值設置為null,以便在關閉鍵盤時刪除邊框顏色。 所以我們可以做如下的事情
export default class App extends React.Component {
constructor(props) {
super(props);
// construct an array with the number of textInputs we require,
// each value an empty string
// set this array in state
// set the focusedIndex to null
let textArray = Array(6).fill('');
this.state = {
textArray: textArray,
focusedIndex: null
}
}
// this function will handle setting of the state when each TextInput changes
onChangeText = (text, index) => {
// as there are going to be a lot of setState calls
// we need access the prevState before we set the next state.
this.setState(prevState => {
prevState.textArray[index] = text
return {
textArray: prevState.textArray
}
}, () => console.log(this.state.textArray))
}
// handle the border color
handleBorderColor = (index) => {
return index === this.state.focusedIndex ? 'red' : 'grey'
}
render() {
// here we map the items in the `this.state.textArray`
// notice that each TextInput is give a specific value in state
// that will stop the overlap
return (
<View style={styles.container}>
{this.state.textArray.map((text, index) => {
return <TextInput
style={{height: 40, marginVertical: 10, borderColor: this.handleBorderColor(index), borderWidth: 1}}
onChangeText={text => this.onChangeText(text, index)}
value={this.state.textArray[index]}
placeholder={`placeholder for ${index}`}
onFocus={() => this.setState({focusedIndex: index})}
onBlur={() => this.setState({focusedIndex: null})}
/>
})}
</View>
);
}
}
然后,如果您想訪問TextInput
的特定值,則可以這樣做
let value = this.state.textArray[index]; // where the index is the value you want
這是一個示例小吃,顯示了運行https://snack.expo.io/@andypandy/map-multiple-textinputs的代碼
絕對值得閱讀以下有關狀態的文章,因為我在此示例中使用了這些屬性。
https://medium.learnreact.com/setstate-is-asynchronous-52ead919a3f0 https://medium.learnreact.com/setstate-takes-a-callback-1f71ad5d2296 https://medium.learnreact.com/setstate-takes-一個功能56eb940f84b6
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.