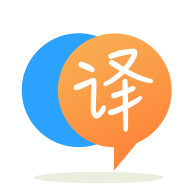
[英]C: Why can I not initialize structs inside of arrays with curly brackets?
[英]C - Pointer inside curly brackets and double pointers
我目前正在學習一些有關基本數據結構的知識,並完成了C中的練習。本實驗室正在研究雙鏈表,這是要附加的基本功能。 數據結構對我來說很有意義,但我的困惑在於代碼。 為什么這里有一個指向列表的雙指針(**)而不是一個*的指針是合適的。 還有為什么(* list)放在括號中?
我一直在研究指針並通過教程。 我理解指針的含義,但是我不確定為什么在這里使用雙指針是合適的。
void append(struct node ** list, int num,)
{
struct node *temp, *current = *list;
if(*list == NULL)
{
*list = (struct node *) malloc(sizeof(struct node));
(*list) -> prev = NULL;
(*list) -> data = num;
(*list) -> next = NULL;
}
else
{
while(current -> next != NULL)
{
current = current -> next;
}
temp = (struct node *) malloc(sizeof(struct node));
temp -> data = num;
temp -> next = NULL;
temp -> prev = current;
current -> next = temp;
}
}
給您有關該結構的信息,這里是它的屬性:
struct node
{
int data;
struct node * prev;
struct node * next;
};
為什么在這里有一個指向列表的雙指針(**)而不是僅一個*
因為我們要更改指針值並將其返回給調用者。 使用相同的方法:
void f(int *x) {
*x = 5;
}
int y;
f(&y);
printf("%d\n", y); // will print 5
同樣的方法
static int x_mem = 5;
void f(int **x) {
// x is a pointer to (pointer to int)
*x = &x_mem;
}
int *y; // pointer to int
f(&y);
printf("%d %p %p\n", **y, (void*)y, (void*)&x_mem); // will print 5 and two same addresses of `x_mem` variable.
在您的函數中,如果列表頭為空,則為列表頭分配內存。 您需要將該指針返回給調用者,以便調用者知道列表頭從何處開始。 所以你也是:
*list = (struct node *) malloc(sizeof(struct node));
還有為什么(* list)放在括號中?
因為->
首先被評估,然后是*
。 這意味着:
*a->b
解析為:
*(a->b)
即:
struct A_s {
int *m;
};
struct A_s *a = malloc(sizeof(struct A_s));
a->m = malloc(sizeof(int));
*a->m = 5;
但是,您首先要取消引用指針並訪問底層結構。 即您有:
struct A_s a_mem;
struct A_s *a = &a_mem;
struct A_s **b = &a;
(*b)->m = malloc(sizeof(int)); // equivalent to `a->m` or `(*a).m` or `(**b).m`
*(*b)->m = 5; // equivalent to `*((*b)->m) = ` or `*(a->m) = ` or `*a->m`
如果要將輸入參數更改為C中的函數,則需要指針。 因此,如果要更改指針,則需要一個指向該指針的指針。 沒有雙指針, *list = (struct node *) malloc(sizeof(struct node));
將不可能。
還有為什么(* list)放在括號中?
因為*list->data
*(list->data) otherwise
將被解釋為*(list->data) otherwise
。 如果要避免所有這些參數,請使用如下所示的臨時指針:
void foo(int ** bar) {
int * ptr = *bar;
ptr->x = 42; // Equivalent to (*bar)->x=42;
}
並且不要轉換malloc。 編寫*list = (struct node *) malloc(sizeof(struct node));
的適當方法*list = (struct node *) malloc(sizeof(struct node));
是*list = malloc(sizeof *list);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.