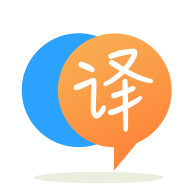
[英]How to enable functionality for dynamically, auto-generated buttons in php?
[英]Adding functionality to buttons generated via a php/html script
我對PHP / HTML / CSS編程非常陌生,在下面所附的代碼中,我試圖創建網站管理面板的基本功能。
我想做的是打印出帶有所有行和列的表,並增加一個帶有控件的列,該控件將允許我從數據庫中刪除該行。 最終,我想為每個用戶更改名稱,密碼和管理員特權。
另外,我真的不知道如何使每個按鈕保持一個將其連接到其相應行的值。
也許由於我是一位經驗不足的程序員,我的所有嘗試都失敗了或刪除了最后一行(也許因為它是$email
變量名下的最后一個值)。 一位朋友建議使用JavaScript或遷移到其他平台(他的建議是Angular JS)以實現我的目標,但到目前為止,我真的想使用PHP使其保持簡單(如果確實如此)和CSS。
這是管理面板的圖像:
這是我的表生成器(或者與我設法得到的一樣好):
<?php
include "connection.php";
$sql = "SELECT * FROM users;";
$result = $conn->query($sql);
if ($result->num_rows > 0)
{
echo "<table class='sqltable'>
<tr class='sqltable'>
<th class='sqltable'>ID</th>
<th class='sqltable'>EMAIL</th>
<th class='sqltable'>NAME</th>
<th class='sqltable'>IS ADMIN</th>
<th class='sqltable'>PASSWORD</th>
<th class='sqltable'>CONTROLS</th>
</tr>";
// output data of each row
while($row = $result->fetch_assoc())
{
echo "<tr class='sqltable'>
<td class='sqlcell'>".$row["ID"]."</td>
<td class='sqlcell'>".$row["EMAIL"]."</td>
<td class='sqlcell'>".$row["FIRST_NAME"]." ".$row["MID_NAME"]." ".$row["LAST_NAME"]."</td>
<td class='sqlcell'>".$row["IS_ADMIN"]."</td>
<td class='sqlcell'>".$row["PASSWORD"]."</td>
<td class='sqlcell'>
<center>
<div style='border: 1px solid lightgray;' method='POST'>
<input type='hidden' name='ID' value='".$row['ID']." '/>
<input type='button' name='delete' value='DEL ".$row['ID']." '/>
</div>
</center>
</td>
</tr>";
}
echo "</table>";
}
else
{
echo "DATABASE IS EMPTY!";
}
$conn->close();
if (isset($_POST['delete']))
{ //if a delete request received
$id = $_POST['id']; //primary key of this row
/////// Connectivity /////////
$servername = "127.0.0.1";
$username = "root";
$password = "";
$db = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $db);
//check connection
if ($conn)
{
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
//compose sql statement
$stmt = mysqli_prepare($conn, "DELETE FROM users WHERE ID=?");
mysqli_stmt_bind_param($stmt,'i',$id); //now add the $id to the statement 'i' stands for integer
mysqli_stmt_execute($stmt);
mysqli_stmt_close($stmt);
mysqli_close($conn); //connection closed
}
?>
這就是我開始做的事情,而且我已經很確定自己走了錯誤的路線。
function delete()
{
$del = "DELETE FROM '".$table."' WHERE EMAIL='".$email."';";
$conn->query($del);
}
好吧,首先,php腳本是服務器端腳本,這意味着您的按鈕不會觸發刪除功能,或者,它將向存在刪除操作的服務器發送請求。
怎么做? 只需在要顯示刪除按鈕的表格單元內呈現一個表單(即html元素),然后將方法定義為post(了解有關http請求方法的更多信息),然后可以包含id值(或該表的主鍵)。
<form method="post">
<input type='submit' name='delete' />
<input type='hidden' name="id" value="$row['id']" />
</form>
因此此表單告訴瀏覽器:每當用戶單擊“刪除”按鈕時,提交包含一個隱藏輸入的此表單,其中包含要刪除的元素的ID。
現在我們去服務器端,在文件的開頭:
<?php
if (isset($_POST['delete'])){ //if a delete request received
$id = $_POST['id']; //primary key of this row
//establish connection to mysql
$mysqli = new mysqli('localhost', 'my_user', 'my_password', 'world');
//check connection
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
//compose sql statement
$stmt = $mysqli->prepare("DELETE FROM users WHERE ID=?");
$stmt->bind_param('i',$id); //now add the $id to the statement 'i' stands for integer
$stmt->execute();
$stmt->close();
$mysqli->close() //connection closed
}
?>
上面的代碼以OOP編寫,也可以以過程樣式編寫。
<?php
if (isset($_POST['delete'])){ //if a delete request received
$id = $_POST['id']; //primary key of this row
//establish connection to mysql
$mysqli = mysqli_connect('localhost', 'my_user', 'my_password', 'world');
//check connection
if (!$mysqli) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
//compose sql statement
$stmt = mysqli_prepare($mysqli, "DELETE FROM users WHERE ID=?");
mysqli_stmt_bind_param($stmt,'i',$id); //now add the $id to the statement 'i' stands for integer
mysqli_stmt_execute($stmt);
mysqli_stmt_close($stmt);
mysqli_close($mysqli) //connection closed
}
?>
現在,當我實現問題中發布的代碼時,我做了幾處更改以使其正常運行,所有小問題,將在此處進行詳細說明。
<?php
if (isset($_POST['delete'])) //first: test if any delete request, delete and then render the table
{ //if a delete request received
$id = $_POST['id']; //primary key of this row, where 'id' index must be case-sensitively equal to the hidden input name 'id'
/////// Connectivity /////////
$servername = "localhost";
$username = "root";
$password = "root";
$db = "user_delete";
// Create connection (procedural style)
$conn = mysqli_connect($servername, $username, $password, $db);
//check connection
if (!$conn) //if NOT connected
{
printf("Connect failed: %s\n", mysqli_connect_error()); //print error
exit(); //exit the program 'in this case you wouldn't see the table either'
}
//compose sql statement
$stmt = mysqli_prepare($conn, "DELETE FROM users WHERE ID=?"); //notice that sql statements are NOT case sensitive
mysqli_stmt_bind_param($stmt,'i',$id); //now add the $id to the statement 'i' stands for integer
mysqli_stmt_execute($stmt);
mysqli_stmt_close($stmt);
mysqli_close($conn); //connection closed, row deleted
}
include "connection.php";
$sql = "SELECT * FROM users;";
$result = $conn->query($sql);
if ($result->num_rows > 0)
{
echo "<table class='sqltable'>
<tr class='sqltable'>
<th class='sqltable'>ID</th>
<th class='sqltable'>EMAIL</th>
<th class='sqltable'>NAME</th>
<th class='sqltable'>IS ADMIN</th>
<th class='sqltable'>PASSWORD</th>
<th class='sqltable'>CONTROLS</th>
</tr>";
// output data of each row
while($row = $result->fetch_assoc())
{
echo "<tr class='sqltable'>";
echo "<td class='sqlcell'>".$row["id"]."</td>"; //php is case-sensitive so you should use $row['ID'] according to your scheme
echo "<td class='sqlcell'>".$row["email"]."</td>";//php is case-sensitive so you should use $row['EMAIL'] according to your scheme
echo "<td class='sqlcell'>".$row["name"]."</td>";//for simplicity, I made one field, change it according to your scheme
echo "<td class='sqlcell'>".$row["is_Admin"]."</td>";//php is case-sensitive so you should use $row['IS_ADMIN'] according to your scheme
echo "<td class='sqlcell'>".$row["password"]."</td>";//same as above
echo "<td class='sqlcell'>
<center>
<div style='border: 1px solid lightgray;'>";
echo "<form method='POST'>"; //must be added in a form with method=post
echo "<input type='hidden' name='id' value='".$row['id']." '/>"; //differntiate between input name `id` and mysql field name you have `ID`, input field name is the index you will fetch in line 4: $_POST['id']
echo "<input type='submit' name='delete' value='DEL ".$row['id']." '/>"; //type: submit, not button
echo "</form>
</div>
</center>
</td>
</tr>";
}
echo "</table>";
}
else
{
echo "DATABASE IS EMPTY!";
}
//all done
$conn->close();
?>
更新:現在這是相同的代碼,全部是OOP樣式並重新使用連接:
<?php
include "connection.php";
if (isset($_POST['delete'])) //first: test if any delete request, delete and then render the table
{ //if a delete request received
$id = $_POST['id']; //primary key of this row, where 'id' index must be case-sensitivly equal to the hidden input name 'id'
//check connection
if (mysqli_connect_errno()) //if connection error existed
{
printf("Connect failed: %s\n", mysqli_connect_error()); //print error
exit(); //exit the program 'in this case you wouldn't see the table either'
}
//compose sql statement
$stmt = $conn->prepare("DELETE FROM users WHERE ID=?"); //notice that sql statements are NOT case sensitive
$stmt->bind_param('i',$id); //now add the $id to the statement 'i' stands for integer
$stmt->execute();
$stmt->close();
}
$sql = "SELECT * FROM users;";
$result = $conn->query($sql);
if ($result->num_rows > 0)
{
echo "<table class='sqltable'>
<tr class='sqltable'>
<th class='sqltable'>ID</th>
<th class='sqltable'>EMAIL</th>
<th class='sqltable'>NAME</th>
<th class='sqltable'>IS ADMIN</th>
<th class='sqltable'>PASSWORD</th>
<th class='sqltable'>CONTROLS</th>
</tr>";
// output data of each row
while($row = $result->fetch_assoc())
{
echo "<tr class='sqltable'>";
echo "<td class='sqlcell'>".$row["id"]."</td>"; //php is case-sensitive so you should use $row['ID'] according to your scheme
echo "<td class='sqlcell'>".$row["email"]."</td>";//php is case-sensitive so you should use $row['EMAIL'] according to your scheme
echo "<td class='sqlcell'>".$row["name"]."</td>";//for simplicity, I made one field, change it according to your scheme
echo "<td class='sqlcell'>".$row["is_Admin"]."</td>";//php is case-sensitive so you should use $row['IS_ADMIN'] according to your scheme
echo "<td class='sqlcell'>".$row["password"]."</td>";//same as above
echo "<td class='sqlcell'>
<center>
<div style='border: 1px solid lightgray;'>";
echo "<form method='POST'>"; //must be added in a form with method=post
echo "<input type='hidden' name='id' value='".$row['id']." '/>"; //differntiate between input name `id` and mysql field name you have `ID`, input field name is the index you will fetch in line 4: $_POST['id']
echo "<input type='submit' name='delete' value='DEL ".$row['id']." '/>"; //type: submit, not button
echo "</form>
</div>
</center>
</td>
</tr>";
}
echo "</table>";
}
else
{
echo "DATABASE IS EMPTY!";
}
//all done
$conn->close();
?>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.