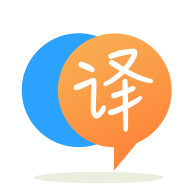
[英]Add same clickable child to top of every group expandablelistview - android
[英]ExpandableListview : want to add child list lazy loading under every group
我已經實現了expandablelistview及其工作正常。 現在,我想在每個子列表的末尾添加“加載更多”按鈕,因此,如果用戶單擊它,它將從服務器獲取更多項。 無論如何,這樣做是因為經過大量搜索后我找不到任何東西。 任何指導是非常感謝。
這是我的自定義適配器:
public class ExpandableListAdapterAssets extends BaseExpandableListAdapter implements Filterable {
List<View> groupViews;
ItemFilter mFilter;
boolean showCheckBox;
ArrayList<Asset> assetsToClaim;
private Context _context;
private List<AssetCategory> _listDataHeader; // header titles
// child data in format of header title, child title
private HashMap<Integer, List<Asset>> _listDataChild;
private RequestBackendHelper requestBackendHelper;
public ExpandableListAdapterAssets(Context context, List<AssetCategory> listDataHeader,
HashMap<Integer, List<Asset>> listChildData, boolean b)
{
this._context = context;
this._listDataHeader = listDataHeader;
this._listDataChild = listChildData;
groupViews = new ArrayList<>();
showCheckBox = b;
assetsToClaim = new ArrayList<>();
}
@Override
public Asset getChild(int groupPosition, int childPosition)
{
return this._listDataChild.get(this._listDataHeader.get(groupPosition).getId())
.get(childPosition);
}
public List<Asset> getChildList(int groupPosition)
{
return this._listDataChild.get(this._listDataHeader.get(groupPosition).getId());
}
@Override
public long getChildId(int groupPosition, int childPosition)
{
return childPosition;
}
@Override
public View getChildView(final int groupPosition, final int childPosition,
boolean isLastChild, View convertView, ViewGroup parent)
{
final Asset assetChild = getChild(groupPosition, childPosition);
if (convertView == null)
{
LayoutInflater infalInflater = (LayoutInflater) this._context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = infalInflater.inflate(R.layout.list_item, null);
}
TextView assetName = (TextView) convertView
.findViewById(R.id.txt_asset_name);
assetName.setText(assetChild.getName());
TextView assetValue = (TextView) convertView
.findViewById(R.id.txt_asset_value);
assetValue.setText(getFormattedAmount(assetChild.getValue()));
((TextView) convertView.findViewById(R.id.txt_asset_category)).setText(assetChild.getAssetCategory());
((TextView) convertView.findViewById(R.id.txt_asset_date)).setText(assetChild.getCreationDate());
if (showCheckBox)
{
CheckBox itemSelect = convertView.findViewById(R.id.chkbox_item_select);
itemSelect.setVisibility(View.VISIBLE);
itemSelect.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked)
{
if (isChecked)
{
assetsToClaim.add(getChild(groupPosition, childPosition));
Log.d("checkbox", "checked: ");
}
else
{
assetsToClaim.remove(getChild(groupPosition, childPosition));
}
}
});
}
else
{
}
return convertView;
}
@Override
public int getChildrenCount(int groupPosition)
{
if (_listDataChild == null)
return 0;
else if (_listDataChild.size() == 0)
return 0;
else
{
int position = this._listDataHeader.get(groupPosition).getId();
int count = this._listDataChild.get(position).size();
return count;
}
}
@Override
public Object getGroup(int groupPosition)
{
return this._listDataHeader.get(groupPosition);
}
@Override
public int getGroupCount()
{
return this._listDataHeader.size();
}
@Override
public long getGroupId(int groupPosition)
{
return groupPosition;
}
@Override
public View getGroupView(final int groupPosition, boolean isExpanded,
View convertView, final ViewGroup parent)
{
if (convertView == null)
{
LayoutInflater infalInflater = (LayoutInflater) this._context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = infalInflater.inflate(R.layout.list_group, null);
}
TextView lblListHeader = (TextView) convertView
.findViewById(R.id.lblListHeader);
lblListHeader.setTypeface(null, Typeface.BOLD);
lblListHeader.setText(_listDataHeader.get(groupPosition).getName());
groupViews.add(convertView);
return convertView;
}
private void requestDeleteCategory(final int groupPosition)
{
requestBackendHelper = new RequestBackendHelper(_context);
if (getChildrenCount(groupPosition) == 0)
{
requestBackendHelper.deleteCategory(_listDataHeader.get(groupPosition).getId(), _context.getSharedPreferences(MY_PREFS_NAME, Context.MODE_PRIVATE).getString("jwt", "")
, new VolleyCallBack() {
@Override
public void onSuccess(String result)
{
try
{
JSONObject parentObj = new JSONObject(result);
if (parentObj.getBoolean("status"))
{
requestBackendHelper.createErrorDialog("Category Deleted.", "success");
_listDataHeader.remove(groupPosition);
_listDataChild.remove(_listDataHeader.get(groupPosition));
notifyDataSetChanged();
}
else
{
requestBackendHelper.createErrorDialog(parentObj.getString("error"), "");
}
}
catch (JSONException e)
{
e.printStackTrace();
}
}
@Override
public void onFailure(VolleyError error)
{
requestBackendHelper.createErrorDialog(error.toString(), "");
}
});
}
else
{
requestBackendHelper.createErrorDialog("The category cannot be deleted until all assets are removed.", "");
}
}
@Override
public boolean hasStableIds()
{
return false;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition)
{
return true;
}
public List<View> getGroupViewList()
{
return groupViews;
}
private void loadEditCategoryAlertDialog(final int groupPosition)
{
View viewInflated = LayoutInflater.from(_context).inflate(R.layout.activity_add_category_dialog, null);
final EditText nameInput = viewInflated.findViewById(R.id.categoryNameEditText);
viewInflated.findViewById(R.id.categoryDescriptionEditText).setVisibility(View.GONE);
final TextView errorLabel = viewInflated.findViewById(R.id.errorTextView);
final AlertDialog dialog = new AlertDialog.Builder(_context)
.setView(viewInflated)
.setTitle("Edit Category")
.setPositiveButton(android.R.string.ok, null)
.setNegativeButton(android.R.string.cancel, null)
.setCancelable(false)
.create();
dialog.setOnShowListener(new DialogInterface.OnShowListener() {
@Override
public void onShow(DialogInterface dialogInterface)
{
Button okButton = (dialog).getButton(AlertDialog.BUTTON_POSITIVE);
okButton.setOnClickListener(new DebouncedOnClickListener() {
@Override
public void onDebouncedClick(View view)
{
final String categoryName = nameInput.getText().toString();
requestRenameCategory(categoryName, groupPosition, dialog, errorLabel);
}
});
}
});
dialog.show();
}
private void requestRenameCategory(final String categoryName, final int groupPosition, AlertDialog dialog, TextView errorLabel)
{
if (categoryName.isEmpty())
{
errorLabel.setText(R.string.add_category_validation_error);
}
else
{
if (requestBackendHelper == null)
requestBackendHelper = new RequestBackendHelper(_context);
requestBackendHelper.attemptEditAssetCategory(createEditCategoryJson(categoryName), _listDataHeader.get(groupPosition).getId()
, _context.getSharedPreferences(MY_PREFS_NAME, Context.MODE_PRIVATE).getString("jwt", "")
, new VolleyCallBack() {
@Override
public void onSuccess(String result)
{
_listDataHeader.get(groupPosition).setName(categoryName);
int size = _listDataHeader.get(groupPosition).getAssetCout();
for (Asset asset : getChildList(groupPosition))
asset.setAssetCategory(categoryName);
notifyDataSetChanged();
}
notifyDataSetChanged();
}
@Override
public void onFailure(VolleyError error)
{
}
});
dialog.dismiss();
}
}
private JSONObject createEditCategoryJson(String categoryName)
{
JSONObject messageObj = new JSONObject();
JSONObject assetCategoryObj = new JSONObject();
try
{
messageObj.put("name", categoryName);
assetCategoryObj.put("asset_category", messageObj);
return assetCategoryObj;
//contactusObj.put(message);
}
catch (JSONException e)
{
e.printStackTrace();
}
return null;
}
/**
* <p>Returns a filter that can be used to constrain data with a filtering
* pattern.</p>
*
* <p>This method is usually implemented by {@link Adapter}
* classes.</p>
*
* @return a filter used to constrain data
*/
@Override
public Filter getFilter()
{
if (mFilter == null)
{
mFilter = new ItemFilter();
}
return mFilter;
}
private class ItemFilter extends Filter {
protected FilterResults performFiltering(CharSequence constraint)
{
FilterResults results = new FilterResults();
return results;
}
/**
* <p>Invoked in the UI thread to publish the filtering results in the
* user interface. Subclasses must implement this method to display the
* results computed in {@link #performFiltering}.</p>
*
* @param constraint the constraint used to filter the data
* @param results the results of the filtering operation
* @see #filter(CharSequence, FilterListener)
* @see #performFiltering(CharSequence)
* @see FilterResults
*/
@Override
protected void publishResults(CharSequence constraint, FilterResults results)
{
}
}
}
這是我設置適配器的方式:
explistAdapter = new ExpandableListAdapterAssets(getActivity(), assetCategories, listDataChild, false);
expListView.setAdapter(explistAdapter);
您需要使用自定義BaseExpandableAdapter
插入“加載更多”按鈕。 此答案似乎相關,因為它顯示了如何提供兩種不同類型的子視圖。 棘手的事情是,當單擊“加載更多”按鈕時,必須將其刪除,然后需要將新項目與底部的一個新的“加載更多”按鈕一起附加在一起-然后是子適配器需要通知。 它的行為必須類似於虛擬列表項,其中getChildView()
提供當前的childPosition
。
剛剛看到提供的代碼; 使用Volley,您最有可能需要在第一次加載時少獲取一個項目,這樣適配器就不會由於添加了一個額外的虛擬項目而運行不同步-或至少在最后添加了view-type 2網絡請求,然后再將其添加到適配器中。 另一個選擇可能是讓getChildCount()
返回少一個; 在分頁時,在任何情況下都必須注意這個額外的項目。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.