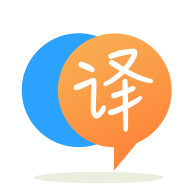
[英]How to extract the ID attribute of a DOM element, if i know corresponding text through Selenium Webdriver and Java
[英]How to extract the display attribute of an element using Selenium Webdriver and Java
無法在div中找到隱藏的元素
<div id="divDuplicateBarcodeCheck" class="spreadsheetEditGui" style="z-
index: 1200; width: 640px; height: 420px; top: 496.5px; left: 640px;
display:block"> ==$0
我想找到顯示元素,但是該元素是隱藏的,我也為此編寫了代碼。
String abc=d.findElement(By.xpath("//div[@id='divDuplicateBarcodeCheck']/"))
.getAttribute("display");
System.out.println(abc);
Thread.sleep(3000);
if(abc.equalsIgnoreCase("block"))
{
d.findElement(By.id("duplicateBarcodeCheck")).click();
System.out.println("duplicate barcode Close");
}
else
{ System.out.println("Barcode selected");}
沒有諸如display
這樣的屬性。 它是style
屬性的一部分。
您可以找到元素並獲取其屬性樣式:
String style = d.findElement(By.xpath("//div[@id='divDuplicateBarcodeCheck']")).getAttribute("style");
if(style.contains("block")) {
d.findElement(By.id("duplicateBarcodeCheck")).click();
System.out.println("duplicate barcode Close");
} else {
System.out.println("Barcode selected");}
}
或者,您可以直接使用cssSelector
找到該元素(也可以使用xpath找到):
WebElement abc = d.findElement(By.cssSelector("div[id='divDuplicateBarcodeCheck'][style*='display: block']"))
請注意,如果找不到該元素,則以上內容將引發NoSuchElementException
。 您可以使用try-catch
塊執行類似的操作,就像在if-else
語句中那樣:
try {
d.findElement(By.cssSelector("div[id='divDuplicateBarcodeCheck'][style*='display: block']"));
d.findElement(By.id("duplicateBarcodeCheck")).click();
System.out.println("duplicate barcode Close");
} catch (NoSuchElementException e) {
System.out.println("Barcode selected");
}
如果您正確無誤,則嘗試存檔檢查是否顯示元素。 您可以使用純硒和java來執行以下操作:
// the @FindBy annotation provides a lazy implementation of `findElement()`
@FindBy(css = "#divDuplicateBarcodeCheck")
private WebElement barcode;
@Test
public void example() {
driver.get("http://some.url");
waitForElement(barcode);
// isDisplay() is natively provided by type WebElement
if (barcode.isDisplayed()) {
// do something
} else {
// do something else
}
}
private void waitForElement(final WebElement element) {
final WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOf(element));
}
您的測試(端到端UI測試!)不應遵循display:none
或display:block
類的實現細節。 想象一下實現將被更改為通過javascript或其他方式刪除元素。 良好的硒測試應始終嘗試盡可能代表真實的用戶觀點。 表示如果UI仍然具有相同的行為,則您的測試仍應成功。 因此,您應該進行更一般的檢查-是否顯示元素。
這是Seleniums WebElement接口的基本功能之一,或更確切地說,它是isDisplayed()
方法。
引用Selenium Java Docs :
boolean isDisplayed()
是否顯示此元素? 此方法避免了必須解析元素的“樣式”屬性的問題。 返回:是否顯示元素
此外,我建議為此類事情編寫一些小的輔助方法,以我的經驗,這是您會經常遇到的常見用例。 例如,輔助方法可能看起來像這樣:
boolean isElementVisible(final By by) {
return driver.findElement(by).isDisplayed();
}
boolean isElementVisible(final WebElement element) {
return element.isDisplayed();
}
如果您使用諸如FluentLenium或Selenide之類的Selenium抽象,則它們將變得更加方便,因為它們為諸如assertJ,hamcrest,junit之類的知名斷言庫提供斷言擴展和自定義匹配器之類的東西。
例如,使用FluentLenium和AssertJ(我個人可以推薦一個堆棧),您的問題的答案就這么簡單:
// check if element is displayed
assertThat(el("#divDuplicateBarcodeCheck")).isDisplayed();
// check if element is not displayed
assertThat(el("#divDuplicateBarcodeCheck")).isNotDisplayed();
還有一些想法:
如果可能,還應該使用CSS選擇器代替xPath選擇器。 CSS選擇器不那么脆弱 ,它將加快您的測試速度並提高可讀性 。
您應該看看隱式等待,而不是使用線程睡眠(不好的做法)。 您可以自己再次實現這樣的輔助方法,例如:
void waitForElement(final WebElement element) {
final WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOf(element));
}
void waitForElement(final By by) {
final WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.visibilityOfElementLocated(by));
}
void waitForElementIsInvisible(final By by) {
final WebDriverWait wait = new WebDriverWait(driver, 5);
wait.until(ExpectedConditions.invisibilityOfElementLocated(by));
}
或(我會建議)為此使用一個庫,例如Awaitility
如果您正在尋找更擴展的示例,可以在這里查看:
似乎需要刪除xpath的末尾有一個多余的/
。 此外,您需要為Web的visibilityOfElementLocated()
OfElementLocated visibilityOfElementLocated()
引入WebDriverWait 。 因此,您的代碼行實際上將是:
String abc = new WebDriverWait(d, 20).until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//label[contains(.,'Leave Balance')]//following::div[@id='applyleave_leaveBalance']"))).getAttribute("style");
System.out.println(abc);
if(abc.contains("block"))
{
d.findElement(By.id("duplicateBarcodeCheck")).click();
System.out.println("duplicate barcode Close");
}
else
{
System.out.println("Barcode selected");
}
實際上, if()
塊仍然是開銷,您可以使用以下方法實現相同的目的:
try {
new WebDriverWait(d, 20).until(ExpectedConditions.elementToBeClickable(By.xpath("//label[contains(.,'Leave Balance')]//following::div[@id='applyleave_leaveBalance']"))).click();
System.out.println("duplicate barcode Close");
} catch (NoSuchElementException e) {
System.out.println("Barcode selected");
}
Q1。 我想找到顯示元素,但是該元素是隱藏的,我也為此編寫了代碼。
A1。 根據您的以下代碼:
<div id="divDuplicateBarcodeCheck" class="spreadsheetEditGui" style="z- index: 1200; width: 640px; height: 420px; top: 496.5px; left: 640px; display:block"> ==$0
它看起來並不隱藏,問題在於您使用了不正確的xpath和element getter。
采用:
String abc = d.findElement(By.xpath("//div[@id='divDuplicateBarcodeCheck']"))
.getCssValue("display");
代替:
=> .getAttribute("display");
使用JavascriptExecutor的替代方法:
JavascriptExecutor jse = (JavascriptExecutor) d;
String displayProperty = (String) jse.executeScript("return
document.getElementById('divDuplicateBarcodeCheck').style.display");
System.out.println("Display property is: "+displayProperty);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.