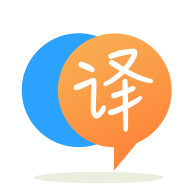
[英]search and find the index of a string in multidimensional array in javascript
[英]Find a coordinate in a multidimensional array using string search
嘗試在這個問題上給一個選擇,不要讓步! 僅使用indexOf和一些整數數學
下面的代碼似乎很有希望,但失敗了。
任何具有更高數學技能的人都想解決它嗎?
var letterVariations = [ [' ','0','1','2','3','4','5','6','7','8','9'], ['A','a','B','b','C','c','D','d','E','e',';'], ['Â','â','F','f','G','g','H','h','Ê','ê',':'], ['À','à','I','i','J','j','K','k','È','è','.'], ['L','l','Î','î','M','m','N','n','É','é','?'], ['O','o','Ï','ï','P','p','Q','q','R','r','!'], ['Ô','ô','S','s','T','t','U','u','V','v','“'], ['W','w','X','x','Y','y','Ù','ù','Z','z','”'], ['@','&','#','[','(','/',')',']','+','=','-'], ]; var string = JSON.stringify(letterVariations); var pos = string.indexOf("u") console.log(Math.floor((pos/10)%8),pos%10) // fails, how to fix? pos = string.indexOf("M") console.log(Math.floor((pos/10)%8),pos%10)
function findPos(array, symbol) { const string = array.toString().replace(/,/g, ''); const pos = string.indexOf(symbol) const d = (array[0] || []).length const x = pos % d; const y = Math.floor(pos / d) return { x, y } } const array = [ [' ', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9'], ['A', 'a', 'B', 'b', 'C', 'c', 'D', 'd', 'E', 'e', ';'], ['Â', 'â', 'F', 'f', 'G', 'g', 'H', 'h', 'Ê', 'ê', ':'], ['À', 'à', 'I', 'i', 'J', 'j', 'K', 'k', 'È', 'è', '.'], ['L', 'l', 'Î', 'î', 'M', 'm', 'N', 'n', 'É', 'é', '?'], ['O', 'o', 'Ï', 'ï', 'P', 'p', 'Q', 'q', 'R', 'r', '!'], ['Ô', 'ô', 'S', 's', 'T', 't', 'U', 'u', 'V', 'v', '“'], ['W', 'w', 'X', 'x', 'Y', 'y', 'Ù', 'ù', 'Z', 'z', '”'], ['@', '&', '#', '[', '(', '/', ')', ']', '+', '=', '-'], ]; console.log(findPos(array,' ')) //=> [0, 0] console.log(findPos(array,'M')) //=> [4, 4] console.log(findPos(array,'u')) //=> [6, 7] console.log(findPos(array,'-')) //=> [8, 10]
您可以連接字符串,並將內部數組的長度用作除數或余數運算符的值。 這僅適用於具有單個字符的字符串。
var letterVariations = [ [' ', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9'], ['A', 'a', 'B', 'b', 'C', 'c', 'D', 'd', 'E', 'e', ';'], ['Â', 'â', 'F', 'f', 'G', 'g', 'H', 'h', 'Ê', 'ê', ':'], ['À', 'à', 'I', 'i', 'J', 'j', 'K', 'k', 'È', 'è', '.'], ['L', 'l', 'Î', 'î', 'M', 'm', 'N', 'n', 'É', 'é', '?'], ['O', 'o', 'Ï', 'ï', 'P', 'p', 'Q', 'q', 'R', 'r', '!'], ['Ô', 'ô', 'S', 's', 'T', 't', 'U', 'u', 'V', 'v', '“'], ['W', 'w', 'X', 'x', 'Y', 'y', 'Ù', 'ù', 'Z', 'z', '”'], ['@', '&', '#', '[', '(', '/', ')', ']', '+', '=', '-'] ], string = letterVariations.map(a => a.join('')).join(''), pos = string.indexOf("u"); console.log(Math.floor(pos / 11), pos % 11); pos = string.indexOf("M") console.log(Math.floor(pos / 11), pos % 11);
這將產生正確的結果。 無需進行字符串化,您可以展平數組並使用indexOf
獲取位置:
var letterVariations = [ [' ', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9'], ['A', 'a', 'B', 'b', 'C', 'c', 'D', 'd', 'E', 'e', ';'], ['Â', 'â', 'F', 'f', 'G', 'g', 'H', 'h', 'Ê', 'ê', ':'], ['À', 'à', 'I', 'i', 'J', 'j', 'K', 'k', 'È', 'è', '.'], ['L', 'l', 'Î', 'î', 'M', 'm', 'N', 'n', 'É', 'é', '?'], ['O', 'o', 'Ï', 'ï', 'P', 'p', 'Q', 'q', 'R', 'r', '!'], ['Ô', 'ô', 'S', 's', 'T', 't', 'U', 'u', 'V', 'v', '“'], ['W', 'w', 'X', 'x', 'Y', 'y', 'Ù', 'ù', 'Z', 'z', '”'], ['@', '&', '#', '[', '(', '/', ')', ']', '+', '=', '-'], ]; var flattened = letterVariations.flat() var findLetter = function(letter) { var pos = flattened.indexOf(letter), x = Math.floor((pos / 10) % 8), y = (pos - (pos % 11)) / 11; return { letter: letter, x: x, y: y } } console.log(findLetter(' ')) //=> [0, 0] console.log(findLetter('M')) //=> [4, 4] console.log(findLetter('u')) //=> [6, 7] console.log(findLetter('-')) //=> [8, 10]
這是一個版本:
var letterVariations = [ [' ','0','1','2','3','4','5','6','7','8','9'], ['A','a','B','b','C','c','D','d','E','e',';'], ['Â','â','F','f','G','g','H','h','Ê','ê',':'], ['À','à','I','i','J','j','K','k','È','è','.'], ['L','l','Î','î','M','m','N','n','É','é','?'], ['O','o','Ï','ï','P','p','Q','q','R','r','!'], ['Ô','ô','S','s','T','t','U','u','V','v','“'], ['W','w','X','x','Y','y','Ù','ù','Z','z','”'], ['@','&','#','[','(','/',')',']','+','=','-'], ]; const findLetterIn = letterVariations => { const width = letterVariations[0].length * 4 + 2; const alpha = JSON.stringify(letterVariations) return (char, pos = alpha.indexOf(char)) => pos > -1 ? [Math.floor((pos - 1) / width), (((pos - 1) % width) - 2)/4] : [-1, -1] } const findLetter = findLetterIn (letterVariations) console.log(findLetter(' ')) //=> [0, 0] console.log(findLetter('M')) //=> [4, 4] console.log(findLetter('u')) //=> [6, 7] console.log(findLetter('-')) //=> [8, 10]
這里的width
與行的寬度有關。
的4
■找做u ~> "u",
在+ 2
具有與添加做[
和]
的開始和結束(以及附加的,
后]
,但除去一個之前。)的- 1
與忽略開頭[
,而- 2
與刪除前導,"
,有關,對於第一個,則與前導["
。
您可以通過將1添加到返回數組的兩個元素中來切換到基於1的索引。
基於@GluePear的答案
您甚至可以在此解決方案中使用多字符
function findPos(array, symbol) { const string = array.flat(); const pos = string.indexOf(symbol) const d = (array[0] || []).length const x = pos % d; const y = Math.floor(pos / d) return { x, y } } const array = [ [' ','0','1','2','3','4','5','6','7','8','9'], ['A','a','B','b','C','c','D','d','E','e',';'], ['Â','â','F','f','G','g','H','h','Ê','ê',':'], ['À','à','I','i','J','j','K','k','È','è','.'], ['L','l','Î','î','M','m','N','n','É','é','?'], ['O','o','Ï','ï','P','p','Q','q','R','r','!'], ['Ô','ô','S','s','T','t','U','u','V','v','“'], ['W','w','X','x','Y','y','Ù','ù','Z','z','”'], ['@','&','#','[','(','/',')',']','+','=','-'], ]; console.log(findPos(array, '-'))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.