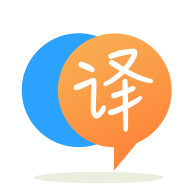
[英]ArrayList “cannot be resolved” when trying to access an ArrayList in a method, Java
[英]NullPointer when trying to access a Java ArrayList<User> that is NOT null
我的UserController中有一個ArrayList,用於存儲我的所有User實例。
我可以使用setUserList成功設置ArrayList,因為我可以循環並使用currentUser.getFirstName()打印用戶的名字
我從JavaFX按鈕觸發我的Login函數,並且在嘗試打印以前大小為ArrayList並打印用戶名的ArrayList時收到NullPointer異常。
UserController的:
public class UserController {
public ArrayList<User> userList; //should contain one user thomas but gives null pointer
//static ArrayList<User> userList; //this does not return a null pointer
public void setUserList(ArrayList list){
userList = list;
for (User user : userList) {
User currentUser = (User) user;
String firstName = currentUser.getFirstName();
System.out.println("Users in the UserController: " + firstName); //prints the user thomas
}
}
public void login(){
try {
System.out.println(userList.size()); //null pointer
} catch (Exception e) {
System.out.println(e);
}
}
}
UserInterface包含登錄方法
public class UserInterface extends Application {
User loggedInUser;
UserRepo userRepo = new UserRepo();
UserController userController = new UserController();
Connection conn;
//login.fxml
@FXML
public TextField InputEmailAddress;
@FXML
public TextField InputPassword;
@Override
public void start(Stage stage) throws Exception {
String connectionURL = "jdbc:derby://localhost:1527/gymManager";
String userName = "root";
String userPassword= "root";
try {
conn = DriverManager.getConnection(connectionURL, userName, userPassword);
if(conn != null){
System.out.println("Connected to the database");
ArrayList<User> list = userRepo.read(conn);
userController.setUserList(list); //here is where I set the ArrayList<Users> in the USerController
Parent root = FXMLLoader.load(getClass().getResource("login.fxml"));
Scene scene = new Scene(root);
stage.setScene(scene);
stage.show();
}
} catch(SQLException e){
System.out.println("Exception:" + e.toString());
}
}
public static void main(String[] args) {
launch(args);
}
public void login(ActionEvent event) throws Exception{
userController.login();
}
}
login.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import java.lang.String?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.image.Image?>
<?import javafx.scene.image.ImageView?>
<?import javafx.scene.layout.Pane?>
<?import javafx.scene.text.Font?>
<Pane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="720.0" prefWidth="1080.0" styleClass="main-pane" xmlns:fx="http://javafx.com/fxml/1" fx:controller="classmanager.UserInterface">
<children>
<Button fx:id="loginButton" layoutX="583.0" layoutY="451.0" onAction="#login" mnemonicParsing="false" prefHeight="56.0" prefWidth="191.0" text="Log In">
<font>
<Font name="Montserrat Regular" size="13.0" />
</font>
<styleClass>
<String fx:value="white-btn" />
<String fx:value="bg-blue" />
<String fx:value="rounded-btn" />
</styleClass></Button>
<Button layoutX="784.0" layoutY="451.0" mnemonicParsing="false" prefHeight="56.0" prefWidth="158.0" text="I'm new here">
<font>
<Font name="Montserrat Regular" size="13.0" />
</font>
<styleClass>
<String fx:value="outline-btn" />
<String fx:value="rounded-btn" />
</styleClass></Button>
<Button layoutX="563.0" layoutY="514.0" mnemonicParsing="false" prefHeight="38.0" prefWidth="231.0" styleClass="transparent-btn" text="I've forgotten my password">
<font>
<Font name="Montserrat Regular" size="13.0" />
</font></Button>
<Label layoutX="581.0" layoutY="273.0" prefHeight="18.0" prefWidth="411.0" text="Book classes and manage your membership details here" textFill="#9a9a9a">
<font>
<Font name="Montserrat Regular" size="14.0" />
</font>
</Label>
<Label layoutX="577.0" layoutY="180.0" prefHeight="18.0" prefWidth="353.0" text="Welcome to the" textFill="#1a73b5">
<font>
<Font name="Montserrat Medium" size="30.0" />
</font>
</Label>
<Label layoutX="577.0" layoutY="217.0" prefHeight="50.0" prefWidth="411.0" text="Village Hotel Gym" textFill="#1a73b5">
<font>
<Font name="Montserrat Medium" size="40.0" />
</font>
</Label>
<ImageView fitHeight="730.0" fitWidth="544.0" layoutX="-25.0" layoutY="-4.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../login-banner.jpg" />
</image>
</ImageView>
<TextField fx:id="InputEmailAddress" layoutX="581.0" layoutY="325.0" prefHeight="50.0" prefWidth="386.0" promptText="Email address" styleClass="login-input">
<opaqueInsets>
<Insets left="20.0" />
</opaqueInsets>
<font>
<Font name="Montserrat Regular" size="13.0" />
</font>
</TextField>
<TextField fx:id="InputPassword" layoutX="581.0" layoutY="384.0" prefHeight="50.0" prefWidth="386.0" promptText="Password" styleClass="login-input">
<opaqueInsets>
<Insets left="20.0" />
</opaqueInsets>
<font>
<Font name="Montserrat Regular" size="13.0" />
</font>
</TextField>
</children>
</Pane>
我簡直不明白為什么UserController類中的一個方法(setUserList)會告訴我ArrayList包含1個用戶,而另一個方法(登錄)將返回NullPointer
謝謝你的任何提示
請更改您的用戶控制器,看看它是否正常工作:
public class UserController {
public ArrayList<User> userList = new ArrayList<>(); //should contain one user thomas but gives null pointer
//static ArrayList<User> userList; //this does not return a null pointer
public void setUserList(ArrayList list){
userList.addAll(list);
for (User user : userList) {
User currentUser = (User) user;
String firstName = currentUser.getFirstName();
System.out.println("Users in the UserController: " + firstName); //prints the user thomas
}
}
public void login(){
try {
System.out.println(userList.size()); //null pointer
} catch (Exception e) {
System.out.println(e);
}
}
}
我打賭在fxml加載時創建了另一個UserInterface
實例,所以你有另一個空的UserController
實例。 將您的UserController
用作login.fxml的控制器。
fx:controller
設置為UserController
(我猜這些類在同一包中)。 <Pane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="720.0" prefWidth="1080.0" styleClass="main-pane" xmlns:fx="http://javafx.com/fxml/1" fx:controller="classmanager.UserController">
UserController
: public class UserController {
public ArrayList<User> userList; //should contain one user thomas but gives null pointer
//static ArrayList<User> userList; //this does not return a null pointer
//login.fxml
@FXML
public TextField InputEmailAddress;
@FXML
public TextField InputPassword;
...
UserInterface
實例化userController
: public class UserInterface extends Application {
User loggedInUser;
UserRepo userRepo = new UserRepo();
UserController userController;
Connection conn;
UserInterface#start()
: public void start(Stage stage) throws Exception {
String connectionURL = "jdbc:derby://localhost:1527/gymManager";
String userName = "root";
String userPassword= "root";
try {
conn = DriverManager.getConnection(connectionURL, userName, userPassword);
if(conn != null){
System.out.println("Connected to the database");
ArrayList<User> list = userRepo.read(conn);
FXMLLoader loader = new FXMLLoader(getClass().getResource("login.fxml"));
Parent root = loader.load();
userController = loader.getController();
userController.setUserList(list);
...
UserInterface
刪除login()
方法並在UserController
重寫login()
,如下所示: @FXML
protected void login(ActionEvent event){
try {
System.out.println(userList.size()); //null pointer
} catch (Exception e) {
System.out.println(e);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.