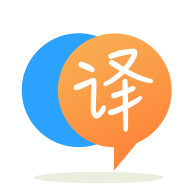
[英]Retrieve specific keys and values from a nested dictionary and assign them into a new dictionary in python 3.X
[英]How can you add entries, and retrieve, alter, or remove values from specific keys in any nested dictionary without recursion?
我正在研究一個很酷的項目,我正在用Python進行操作,我需要一種方法來做到這一點而不需要遞歸,因為這會限制它可能通過限制循環可以通過它的次數(最大遞歸深度)。 該函數需要處理任何大小的嵌套字典。 如何在任何嵌套字典中添加條目,檢索,更改或刪除特定鍵中的值? 我沒有在SO上找到一個好的答案因為它們都過於復雜或者使用遞歸。
我創建了這個簡單的模塊,演示了這個功能。 你必須知道密鑰路徑本身。 您可以從Github獲取演示並在任何項目中使用它,因為它已獲得MIT許可 。 請享用!
看到這個基准測試會很有趣。
'''
MIT License
Copyright (c) 2019 Keith Cronin
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.'''
d = {}
d['fruits'] = {}
d['fruits']['orange'] = {}
d['fruits']['orange']['price'] = 5.75
my_keystring = "fruits.orange.price"
def get_value(keystring, dictionary):
amountkeys = keystring.count('.')+1
lastfoundindex = 0
counter = 0
while counter < amountkeys:
if counter == 0:
value = dictionary[keystring[lastfoundindex:keystring.find('.')]]
elif counter == amountkeys - 1:
value = value[keystring[lastfoundindex:]]
break
else:
value = value[keystring[lastfoundindex:keystring.find('.',lastfoundindex)]]
lastfoundindex = keystring.find('.',lastfoundindex)+1
counter += 1
return value
print(F"Demo get_value(): {get_value(my_keystring, d)}\nThis is the PRICE of ORANGE in FRUITS.")
def set_value(keystring, dictionary, new_value):
amountkeys = keystring.count('.')+1
lastfoundindex = 0
counter = 0
while counter < amountkeys:
if counter == 0:
value = dictionary[keystring[lastfoundindex:keystring.find('.')]]
elif counter == amountkeys - 1:
value[keystring[lastfoundindex:]] = new_value
break
else:
value = value[keystring[lastfoundindex:keystring.find('.',lastfoundindex)]]
lastfoundindex = keystring.find('.',lastfoundindex)+1
counter += 1
value = new_value
return value
print(F"Demo set_value(): {set_value(my_keystring, d, 1.25)}\nThis is the NEW PRICE of ORANGE in FRUITS!")
def del_entry(keystring, dictionary):
amountkeys = keystring.count('.')+1
lastfoundindex = 0
counter = 0
while counter < amountkeys:
if counter == 0:
value = dictionary[keystring[lastfoundindex:keystring.find('.')]]
elif counter == amountkeys - 1:
del value[keystring[lastfoundindex:]]
break
else:
value = value[keystring[lastfoundindex:keystring.find('.',lastfoundindex)]]
lastfoundindex = keystring.find('.',lastfoundindex)+1
counter += 1
del_entry('fruits.orange.price',d)
print(F"Demo del_entry(): fruits.orange.price is now {get_value('fruits.orange', d)}!")
def add_entry(keystring, dictionary, entry_name, entry_value = None):
amountkeys = keystring.count('.')+1
lastfoundindex = 0
counter = 0
while counter < amountkeys:
if counter == 0:
value = dictionary[keystring[lastfoundindex:keystring.find('.')]]
elif counter == amountkeys - 1:
value[keystring[lastfoundindex:]][entry_name] = entry_value
break
else:
value = value[keystring[lastfoundindex:keystring.find('.',lastfoundindex)]]
lastfoundindex = keystring.find('.',lastfoundindex)+1
counter += 1
add_entry('fruits.orange', d, 'in_stock', True)
print(F"Demo add_entry()! Added a new entry called in_stock to fruits.orange, it's value is: {get_value('fruits.orange.in_stock',d)}")
那么,你也可以采取另一種方式:
d = {}
my_keystring = "fruits.orange.price"
d[my_keystring] = 5.75
print(F"Demo get value: {d[my_keystring]}\nThis is the PRICE of ORANGE in FRUITS.")
d[my_keystring] = 1.25
print(F"Demo set value: {d[my_keystring]}\nThis is the NEW PRICE of ORANGE in FRUITS!")
del d[my_keystring]
print(F"Demo del entry: fruits.orange.price is now {d.get(my_keystring)}!")
d['fruits.orange.in_stock'] = True
print(F"Demo add entry! Added a new entry called in_stock to fruits.orange, it's value is: {d['fruits.orange.in_stock']}")
哪個收益率
Demo get value: 5.75
This is the PRICE of ORANGE in FRUITS.
Demo set value: 1.25
This is the NEW PRICE of ORANGE in FRUITS!
Demo del entry: fruits.orange.price is now None!
Demo add entry! Added a new entry called in_stock to fruits.orange, it's value is: True
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.