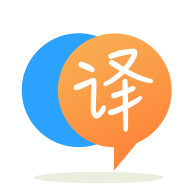
[英]Load random images from folder but without repeat using javascript math.floor( math.random) function
[英]How to create a random number generator function using JavaScript without using the Math.random() function?
我想使用 JavaScript 創建一個隨機數生成器函數,它將返回一個介於 0 和 1 之間的隨機數。是否可以在不使用Math.random()
函數的情況下創建一個自定義隨機數生成器函數?
我試過這種方法。 它有效,但我不知道它是真的隨機還是有任何模式?
var lastRand = 0.5; function rand(){ var x = new Date().getTime()*Math.PI*lastRand; var randNum = x%1; lastRand = randNum; return randNum; } // Output for(var n = 0; n < 50; n++){ console.log(rand()); }
此函數依賴於變量lastRand
來生成數字。 但我想要更有效的方法來做到這一點。
為什么不這樣呢? 對我來說似乎更隨機,因為它不依賴於值。
var lastRand, randNum; function rand(){ while(randNum == lastRand) randNum = (new Date().getTime()*Math.PI)%1; return lastRand = randNum; } // Output for(var n = 0; n < 50; n++) console.log(rand());
我有更好的解決方案。 它使用對象而不是外部變量。
var Random = { "lastRand" : 0.5, "randNum" : 0.5, "rand" : function(){ while(this.randNum == this.lastRand){ this.randNum = (new Date().getTime()*Math.PI*this.lastRand)%1; } return this.lastRand = this.randNum; } } for(var n = 0; n < 50; n++){ console.log(Random.rand()); }
這是使用不需要外部變量的函數生成器的版本。
function *makeRand() {
let seed = new Date().getTime()
while (true) {
seed = seed * 16807 % 2147483647
yield seed / 2147483647
}
}
// Usage
let rand = makeRand()
for (let i = 0; i < 5; i++) {
console.log(rand.next().value)
}
// 0.05930273982663767
// 0.7011482662992311
// 0.1989116911771296
// 0.10879361401721538
// 0.4942707873388523
資料來源: https : //www.cse.wustl.edu/~jain/iucee/ftp/k_26rng.pdf
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.