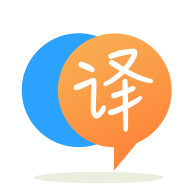
[英]How can I fix the swapBack function to get the result I intended?
[英]How would I fix this if statement in C to get it working as intended?
在“聯系人管理系統”的這段代碼中,我很難獲得一行的預期輸出。 基本上,在這一部分中,當您添加新聯系人時,它會要求您“請輸入公寓編號”,如下所示:
if (yes() == 1)
{
printf("Please enter the contact's apartment number: ");
address->apartmentNumber = getInt();
if (address->apartmentNumber > 0)
{
}
else
{
printf("*** INVALID INTEGER *** <Please enter an integer>: ");
address->apartmentNumber = getInt();
}
}
else
{
address->apartmentNumber = 0;
}
現在,根據我的任務,您應該輸入單詞“ bison”(而不是數字,得到它?),該單詞將顯示輸出:
* INVALID INTEGER *請輸入一個整數:
對於上下文,這部分工作正常。 但是,您將被指示輸入整數“ -1200”,然后將彈出提示
*無效的公寓號*請輸入一個正數:
這是我遇到的問題,因為簡單地說,無論是在if語句中還是在if語句之外,我都不知道將其放在哪里。 我不確定,並希望對此有所幫助。
我試圖自己解決問題,但這只是使我獲得了無效整數輸出的兩倍,而不是這個正確的無效公寓號碼聲明。 這是我的(失敗)嘗試:
if (yes() == 1)
{
printf("Please enter the contact's apartment number: ");
address->apartmentNumber = getInt();
if (address->apartmentNumber > 0)
{
}
else
{
printf("*** INVALID INTEGER *** <Please enter an integer>: ");
address->apartmentNumber = getInt();
}
if (address->apartmentNumber < 0)
{
}
else
{
printf("*** INVALID APARTMENT NUMBER *** <Please enter a positive number>: ");
address->apartmentNumber = getInt();
}
else
{
address->apartmentNumber = 0;
}
編輯:對於那些要求getInt()和yes()代碼的人,這里:
getInt()
int getInt(void)
{
int num;
char nl;
scanf("%d%c", &num, &nl);
while (nl != '\n') {
clearKeyboard();
printf("*** INVALID INTEGER *** <Please enter an integer>: ");
scanf("%d%c", &num, &nl);
}
return num;
}
是的():
int yes(void)
{
int yesno, flag;
char c, nl;
scanf("%c%c", &c, &nl);
do {
if (nl != '\n') {
clearKeyboard();
printf("*** INVALID ENTRY *** <Only (Y)es or (N)o are acceptable>: ");
flag = 1;
scanf("%c%c", &c, &nl);
}
else if (c != 'Y' && c != 'y' && c != 'N' && c != 'n') {
printf("*** INVALID ENTRY *** <Only (Y)es or (N)o are acceptable>: ");
flag = 1;
scanf("%c%c", &c, &nl);
}
else if (nl == '\n' && (c == 'Y' || c == 'y' || c == 'N' || c == 'n'))
{
flag = 0;
}
} while (flag == 1);
if (c == 'Y' || c == 'y') {
yesno = 1;
}
else {
yesno = 0;
}
return yesno;
}
getInt
將處理非整數(文本和單詞)輸入,並提示用戶鍵入整數,直到輸入為止。
因此,您的代碼需要更像這樣:
if (yes() == 1)
{
int validNumber = 0;
while (validNumber == 0)
{
printf("Please enter the contact's apartment number: ");
address->apartmentNumber = getInt();
if (address->apartmentNumber > 0)
{
validNumber = 1;
}
else
{
printf("* INVALID APARTMENT NUMBER * Please enter a positive number:\n");
}
}
}
您的getint()
函數非常脆弱。 用戶僅輸入一次非整數值是什么意思? 接受特定類型的輸入時,通常最好連續循環直到收到有效輸入(或用戶取消輸入)為止,並始終防止輸入的輸入不是您期望的(例如當貓踩鍵盤時) ...)
如果正確使用,可以使用scanf
。 這意味着您有責任每次都檢查scanf
的返回 。 您必須處理三個條件
(return == EOF)
用戶通過按Ctrl + d生成手動EOF
(或在Windows Ctrl + z上 ,但請參見CTRL + Z在Windows 10(早期版本)中不會生成 (return == EOF)
來取消輸入; (return < expected No. of conversions)
發生匹配或輸入失敗。 對於匹配失敗,您必須考慮輸入緩沖區中剩余的每個字符。 (向前掃描輸入緩沖區,讀取並丟棄字符,直到找到'\\n'
或EOF
); 最后 (return == expected No. of conversions)
表示讀取成功-然后由您檢查輸入是否滿足任何其他條件(例如,正整數,正浮點,在所需范圍內等)。 。 將其與getint()
函數一起使用並傳遞一個字符串以顯示為用戶提示(如果不是NULL
),則可以執行以下操作:
int getint (int *value, const char *prompt)
{
/* loop continually until good input or canceled */
for (;;) {
int rtn; /* variable for return from scanf */
if (prompt) /* if not NULL */
fputs (prompt, stdout); /* display prompt */
rtn = scanf ("%d", value); /* attempt read */
if (rtn == EOF) { /* check for manual EOF */
fputs ("<user canceled input>\n", stderr);
return 0;
}
empty_stdin(); /* all other cases - empty input buffer */
if (rtn == 1) /* good input, break */
break;
/* otherwise matching failure */
fputs (" error: invalid integer input.\n", stderr);
}
return *value; /* value also available through pointer */
}
( 注意:從函數返回的值是對函數是否成功(返回1
)或用戶是否通過EOF
取消(返回0
)的驗證,整數值可通過指針value
提供給調用方)
輔助函數empty_stdin()
簡單來說就是:
void empty_stdin (void)
{
int c = getchar();
while (c != '\n' && c != EOF)
c = getchar();
}
有多種方法可以將getint()
放在一起以根據需要進行調整,只要您能正確處理上述所有三種情況,就可以隨意使用任意方式。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.