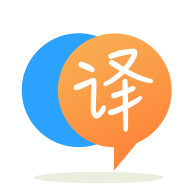
[英]How do I deserialize an array of objects using gson and JsonWriter
[英]How do I deserialize a nested JSON array using GSON?
這是我的JSON
示例:
{
"status": "ok",
"rowCount": 60,
"pageCount": 6,
"value": [{
"CustomerID": 1911,
"CustomerTypeID": 3,
...
}
]
}
我的POJO:
@SerializedName("CustomerID")
public Integer CustomerID;
@SerializedName("CustomerTypeID")
public Integer CustomerTypeID;
我想把所有東西都拉到value
之下。
如何使用 Google 的 GSON 執行此操作?
我試過像往常一樣這樣做,但由於顯而易見的原因,它沒有用:
Type collectionType = new TypeToken<ArrayList<Customer>>() {}.getType();
return gson.fromJson(json, collectionType);
您不能跳過根JSON object
。 在這種情況下,最簡單的解決方案是 - 創建根POJO
:
class Response {
@SerializedName("value")
private List<Customer> customers;
// getters, setters
}
您可以按如下方式使用它:
return gson.fromJson(json, Response.class).getCustomers();
您無需擔心編寫自己的 POJO。
只需訪問http://www.jsonschema2pojo.org/並在此處粘貼您的 JSON 數據,它會自動返回您轉換后的類,如下所示
--------------------- com.example.Example.java-------- ---------------------------
package com.example;
import java.util.List;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class Example {
@SerializedName("status")
@Expose
private String status;
@SerializedName("rowCount")
@Expose
private Integer rowCount;
@SerializedName("pageCount")
@Expose
private Integer pageCount;
@SerializedName("value")
@Expose
private List<Value> value = null;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Integer getRowCount() {
return rowCount;
}
public void setRowCount(Integer rowCount) {
this.rowCount = rowCount;
}
public Integer getPageCount() {
return pageCount;
}
public void setPageCount(Integer pageCount) {
this.pageCount = pageCount;
}
public List<Value> getValue() {
return value;
}
public void setValue(List<Value> value) {
this.value = value;
}
}
------------------------------------- com.example.Value.java-------- ---------------------------
package com.example;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class Value {
@SerializedName("CustomerID")
@Expose
private Integer customerID;
@SerializedName("CustomerTypeID")
@Expose
private Integer customerTypeID;
public Integer getCustomerID() {
return customerID;
}
public void setCustomerID(Integer customerID) {
this.customerID = customerID;
}
public Integer getCustomerTypeID() {
return customerTypeID;
}
public void setCustomerTypeID(Integer customerTypeID) {
this.customerTypeID = customerTypeID;
}
}
以上兩個類是由網站自動生成的。
import java.util.List;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class ExampleClass {
@SerializedName("status")
@Expose
private String status;
@SerializedName("rowCount")
@Expose
private int rowCount;
@SerializedName("pageCount")
@Expose
private int pageCount;
@SerializedName("value")
@Expose
private List<Value> value = null;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public int getRowCount() {
return rowCount;
}
public void setRowCount(int rowCount) {
this.rowCount = rowCount;
}
public int getPageCount() {
return pageCount;
}
public void setPageCount(int pageCount) {
this.pageCount = pageCount;
}
public List<Value> getValue() {
return value;
}
public void setValue(List<Value> value) {
this.value = value;
}
}
-------------------------Value.java------------ -----------------------
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class Value {
@SerializedName("CustomerID")
@Expose
private int customerID;
@SerializedName("CustomerTypeID")
@Expose
private int customerTypeID;
public int getCustomerID() {
return customerID;
}
public void setCustomerID(int customerID) {
this.customerID = customerID;
}
public int getCustomerTypeID() {
return customerTypeID;
}
public void setCustomerTypeID(int customerTypeID) {
this.customerTypeID = customerTypeID;
}
}
/********* 使用 Gson 解析 ******/
GsonBuilder gsonBuilder = new GsonBuilder();
gson = gsonBuilder.create();
ExampleClass resultObj = gson.fromJson(jsonObject.toString(), ExampleClass.class);
List<Value> yourListOfCustomerValues = resultObj.getValue();
您可以參考 Norman Peitek 撰寫的有關使用 Gson 映射數組和對象列表的精彩帖子
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.