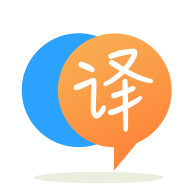
[英]Given a set of letters, how to create all possible combinations of a word with these letters, duplicated with a specified number?
[英]Given a word and a number for each letter of this word, how to create a full tree of every combinations?
假設我有一個單詞“ stack”,並且每個字母都有一個數字,該數字將定義字母必須重復的次數。 在JSON文件中,我將具有以下內容:
{
"s": 4,
"t": 3,
"a": 2,
"c": 5,
"k": 2
}
由此,我想生成所有可能組合的完整樹,即:
Depth 1: stack
Depth 2: stack, sstack, ssstack, sssstack
Depth 3: stack, sttack, stttack, sstack, ssttack, sstttack,ssstack, sssttack, ssstttack, sssstack, ssssttack, sssstttack
Depth 4: ... with 'a'
Depth 5: ... with 'c'
Depth 6: ... with 'k'
這將提供4 * 3 * 2 * 5 * 2 = 240個可能性。 另外,我有幾天前在這里問過的人提供的這些功能,對此我做了一些修改:
def all_combinations(itr):
lst = list(itr)
for r in range(1, len(lst) + 1):
for perm in itertools.combinations(lst, r=r):
yield perm
def all_repeats(n, letters, word):
for rep in all_combinations(letters):
yield ''.join(char * n if char in rep else char for char in word)
這給了我:
word = 'stack'
for i in range(1,5):
liste.append(''.join(list(all_repeats(i, ['s'], word))))
Output: ['stack', 'sstack', 'ssstack', 'sssstack']
由此,給定JSON文件中的一對(字母,數字),我如何遞歸調用此函數來創建所有可能性?
幾個版本,首先我們使用列表,因為它更易於索引
options = [
("s", 4),
("t", 3),
("a", 2),
("c", 5),
("k", 2),
]
現在我們可以獲得長格式的版本,以幫助了解發生了什么:
output = ['']
for letter, repeats in options:
tmp = []
for num in range(repeats):
postfix = letter * (num+1)
for cur in output:
tmp.append(cur + postfix)
output = tmp
如果print(output)
您將得到期望的結果。 我建議在調試器中運行或插入大量print
語句以了解發生的情況
作為第二個版本,我們可以使用itertools
其縮短為:
from itertools import product
tmp = [[l*(i+1) for i in range(num)] for l, num in options]
output = [''.join(l) for l in product(*tmp)]
您甚至可以將所有內容放在一起,但是我認為它有點太難以理解
遞歸解決方案也很合適,但我會留給您
您還可以使用遞歸:
data = {'s': 4, 't': 3, 'a': 2, 'c': 5, 'k': 2}
def combos(d):
yield ''.join(map(''.join, d))
for i in data:
if any(c[0] == i and len(c) < data[i] for c in d):
yield from combos([c+[i] if c[0] == i and len(c) < data[i] else c for c in d])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.