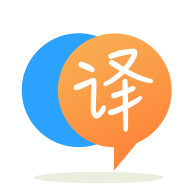
[英]How can I update entry widget entries in a toplevel window in tkinter?
[英]How can i update a tkinter window with a new defined variable?
我想創建兩個窗口,第一個窗口中有一個按鈕,第二個窗口中有一個標簽。 當我按下按鈕時,第二個窗口中的標簽應該刷新並顯示變量加1。
我不想關閉第二個窗口然后重新打開它。 有什么想法可以做到嗎?
import tkinter as tk
text = 1
root = tk.Tk()
root2 = tk.Tk()
label = tk.Label(root, bg="green", text=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
def plus():
global text
text = text + 1
print(text)
button = tk.Button(root2, bg="blue", text="Button", command=plus)
button.pack()
root.mainloop()
root2.mainloop()
此代碼應該執行基礎知識,但仍然缺少刷新第二個窗口的行。
希望有人能告訴我如何:)
您不能在一個線程中運行兩個主循環。 您對root.mainloop()
調用會阻塞,直到您關閉窗口。 關閉窗口后,它將退出該方法並運行root2.mainloop()
因為它是下一行代碼。
一個根窗格可以有多個窗口,您不需要創建root2
。 你想要制作兩個Toplevel窗口
要擴展已經給出的答案,您應該使用Toplevel而不是兩個根窗口。 另外,為了更改標簽的文本,您可以使用label['text'] = str(text)
來更新值。
import tkinter as tk
text = 1
root = tk.Tk()
root2 = tk.Toplevel()
def plus():
global text
text = text + 1
label['text'] = str(text)
label = tk.Label(root, bg="green", text=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
button = tk.Button(root2, bg="blue", text="Button", command=plus)
button.pack()
root.mainloop()
如果我明白了,試試這個,每次打開一個窗口,頂部,新窗口中的標簽增加1
import tkinter as tk
class Test(tk.Toplevel):
def __init__(self, parent, count):
super().__init__()
self.parent = parent
self.count = tk.IntVar()
self.count.set(count)
self.title("I'm a new toplevel.")
self.init_ui()
def init_ui(self):
self.label = tk.Label(self, textvariable=self.count).pack()
self.close_me = tk.Button(self, text= "Close me", command = self.on_close_me).pack()
self.close_parent = tk.Button(self, text= "Close parent", command = self.on_close_parent).pack()
def on_close_me(self):
self.destroy()
def on_close_parent(self):
self.parent.on_close()
class App(tk.Frame):
def __init__(self,):
super().__init__()
self.master.title("Hello World")
self.count =0
self.init_ui()
def init_ui(self):
self.pack(fill=tk.BOTH, expand=1,)
f = tk.Frame()
w = tk.Frame()
tk.Button(w, text="Open", command=self.callback).pack()
tk.Button(w, text="Close", command=self.on_close).pack()
f.pack(side=tk.LEFT, fill=tk.BOTH, expand=0)
w.pack(side=tk.RIGHT, fill=tk.BOTH, expand=0)
def callback(self):
self.count +=1
obj = Test(self,self.count)
def on_close(self,evt=None):
self.master.destroy()
if __name__ == '__main__':
app = App()
app.mainloop()
使用Toplevel()
窗口而不是使用Tk()
作為輔助窗口。 Tkinter是單線程的,因此使用一個mainloop()
就足以滿足所有窗口的要求。
現在,當您要求在一個窗口中有一個按鈕和在第二個窗口中更新的標簽時,如果關閉第二個窗口,您也可以重新打開窗口而不會丟失任何進度。
這是我的方式。
import tkinter as tk
text = 1
root = tk.Tk()
root2 = tk.Toplevel()
label = tk.Label(root2, bg="green", text=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
def plus():
global text, root2, label
if not root2.winfo_exists(): # checks if the window is closed or not
root2 = tk.Toplevel()
label = tk.Label(root2, bg="green", text=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
text += 1
label['text'] = text
button = tk.Button(root, bg="blue", text="Button", command=plus)
button.pack()
root.mainloop()
使用IntVar()
更新所有標簽值。 可以使用StringVar()
作為sting類型:
樣品
import tkinter as tk
root = tk.Tk()
root2 = tk.Toplevel()
# This is a textvariable IntVar can also use StringVar for sting input
text = tk.IntVar()
text.set(1) # Set the initial to 1
# Use 'textvariable' instad of text
label = tk.Label(root2, bg="green", textvariable=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
label2 = tk.Label(root, textvariable=text)
label2.pack()
def plus():
global text, root2, label
if not root2.winfo_exists(): # checks if the window is closed or not
root2 = tk.Toplevel()
label = tk.Label(root2, bg="green", textvariable=text, fg="blue")
label.place(relx=0.1, rely=0.1, relheight=0.3, relwidth=0.3)
text.set( text.get()+1 ) # incrementing by 1 ( text.get() will return the value )
button = tk.Button(root, bg="blue", text="Button", command=plus)
button.pack()
root.mainloop()
我希望你能從我的答案中找到有用的東西。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.