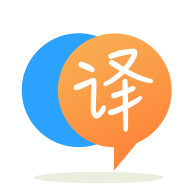
[英]Optional int parameter 'page' is present but cannot be translated into a null value due to being declared as a primitive type.
[英]Optional int parameter '' is present but cannot be translated into a null value due to being declared as a primitive type
BrandDao.java
package dao;
import java.util.List;
import model.pojo.Brands;
import model.util.HibernateUtil;
import org.hibernate.HibernateException;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
public class BrandDao {
public static List<Brands> list() {
List<Brands> brands = null;
try {
Session session = HibernateUtil.getSessionFactory().openSession();
Query query = session.createQuery("from Brands");
brands = query.list();
session.close();
}
catch (HibernateException ex) {
ex.printStackTrace();
}
return brands;
}
public Brands getBrandById(int brandId) {
SessionFactory sessionFactory = HibernateUtil.getSessionFactory();
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Brands brand = (Brands) session.get(Brands.class, brandId);
tx.commit();
session.close();
return brand;
}
public Brands addBrand(Brands brand) {
try {
SessionFactory sessionFactory = HibernateUtil.getSessionFactory();
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
session.save(brand);
tx.commit();
session.close();
} catch (HibernateException ex) {
ex.printStackTrace();
}
return brand;
}
}
BrandsController.java
package controller;
import dao.BrandDao;
import java.io.IOException;
import java.util.List;
import model.pojo.Brands;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class BrandsController {
ModelAndView mav = new ModelAndView();
@RequestMapping(value="/admin/brands")
public ModelAndView index() {
List<Brands> brands = BrandDa.list();
mav.addObject("brands", brands);
mav.setViewName("brands-admin");
return mav;
}
@RequestMapping(value="/admin/brands/add", method = RequestMethod.POST)
public String addBrand(@RequestParam("photo") MultipartFile photo, @RequestParam("brandName") String name) throws IOException {
Brands brand = new Brands();
BrandDao brandDao = new BrandDa();
byte[] photoBytes = photo.getBytes();
brand.setBrandLogo(photoBytes);
brand.setBrandName(name);
brandDao.addBrand(brand);
return "redirect:/admin/brands";
}
@RequestMapping(value="/admin/brands/getBrand", method = RequestMethod.GET)
@ResponseBody
public Brands getBrand(int brandId) {
BrandDao brandDao = new BrandDa();
return brandDao.getBrandById(brandId);
}
}
品牌,admin.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@taglib prefix="form" uri="http://www.springframework.org/tags/form"%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>House of Beauty</title>
<link rel="stylesheet" href="${pageContext.request.contextPath}/resources/css/styles.css" />
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
<script src="https://code.jquery.com/jquery-3.4.0.min.js"></script>
<script>
$(document).ready(function() {
$('#getBrand').on('click', function(event) {
event.preventDefault();
var href = $(this).attr('href');
$.get(href, function(brand, status) {
$('#BrandForm #name').val(brand.getBrandName());
$('#BrandForm #photo').val(brand.getBrandLogo());
});
$('#BrandForm').modal('show');
});
});
</script>
</head>
<body>
<div class="modal fade" id="BrandForm" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title w-100" id="myModalLabel">Edit brand</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form method="post" enctype="multipart/form-data" action="${pageContext.request.contextPath}/admin/brands/update">
<div class="form-group">
<input type="text" name="brandName" id="name" placeholder="Name" cssClass="form-control"/>
</div>
<div class="form-group">
<input type="file" name="photo" id="photo"/>
</div>
<div class="row form-bottom">
<div class="col">
<input type="submit" value="Save" class="btn" id="btnAdd">
<input type="submit" value="Delete" class="btn" id="btnAdd">
</div>
</div>
<form>
</div>
</div>
</div>
</div>
<div class="modal fade" id="AddBrandForm" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title w-100" id="myModalLabel">Add brand</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form method="post" enctype="multipart/form-data" action="${pageContext.request.contextPath}/admin/brands/add">
<div class="form-group">
<input type="text" name="brandName" placeholder="Name" cssClass="form-control"/>
</div>
<div class="form-group">
<input type="file" name="photo"/>
</div>
<div class="row form-bottom">
<div class="col">
<input type="submit" value="Add" class="btn" id="btnAdd">
</div>
</div>
<form>
</div>
</div>
</div>
</div>
<header>
...
<div id="main_content">
<div class="container">
<div class="row header-content">
<div class="col title-page">
<h3>Brands</h3>
</div>
<div class="col">
<a href="#AddBrandForm" data-dismiss="modal" data-toggle="modal">Add brand</a>
</div>
</div>
<hr>
<div class="row">
<c:forEach items="${brands}" var="brand">
<div class="col">
<div class="brand-item">
<a href="${pageContext.request.contextPath}/admin/brands/getBrand?id=<c:out value="${brand.getBrandId()}"/>" id="getBrand">
<div class="image">
<img src="${pageContext.request.contextPath}/images?id=<c:out value="${brand.getBrandId()}"/>" />
</div>
<p><c:out value="${brand.getBrandName()}"/></p>
</a>
</div>
</div>
</c:forEach>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</body>
</html>
我正在嘗試從模式中編輯一個品牌,就像我添加它一樣。 我對彈簧和冬眠都是全新的,我不知道我是不是以正確的方式做到了。 點擊品牌鏈接,打開模態,我收到此錯誤:
HTTP Status 500 - Internal Server Error
type Exception report
messageInternal Server Error
descriptionThe server encountered an internal error that prevented it from fulfilling this request.
exception
org.springframework.web.util.NestedServletException: Request processing failed; nested exception is java.lang.IllegalStateException: Optional int parameter 'brandId' is present but cannot be translated into a null value due to being declared as a primitive type. Consider declaring it as object wrapper for the corresponding primitive type.
root cause
java.lang.IllegalStateException: Optional int parameter 'brandId' is present but cannot be translated into a null value due to being declared as a primitive type. Consider declaring it as object wrapper for the corresponding primitive type.
note The full stack traces of the exception and its root causes are available in the GlassFish Server Open Source Edition 4.1.1 logs.
GlassFish Server Open Source Edition 4.1.1
請,如果有人可以幫助我如何獲得品牌的價值並能夠通過模態進行編輯,我將非常感謝您的幫助。 提前致謝。
在您的控制器類中,'brandId'接收空值,而您將其定義為'int'類型,該類型不能為null(因為您的錯誤語句也提到)。 從'int'更改為'Integer'。
而且,不確定在請求中獲取此參數的位置。 我知道你也需要定義路徑參數。 (這只是觀察)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.