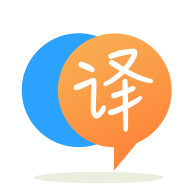
[英]TypeScript how to create a generic type alias for a generic function?
[英]How to create a specific alias based on a generic typescript interface
考慮以下接口:
export interface FooInterface<T, O extends FooOptions<T>> {
items: T[];
options: O;
}
interface FooOptions<T> {
doSomething: (e: T) => void;
}
現在,每當我需要對此做些事情時,我都需要重復T。例如:
const t: FooInterface<string, FooOptions<string>> = {
items: ["a","b","c"],
options: {doSomething: (e) => {
console.log(e);
}}
}
現在,重復很無聊,是否有可能創建一個類型或接口,其中T對於這兩種類型都應該是字符串,或者以某種方式重新定義接口,所以我改為這樣寫:
const t: FooInterfaceString
//or
const t: FooInterfaceOfType<string>
怎么樣 :
type FooInterfaceString = FooInterface<string, FooOptions<string>>;
另外,如果適合您的設計,可以考慮以下方法:
export interface FooInterface<T> {
items: T[];
options: FooOptions<T>;
}
type FooInterfaceString = FooInterface<string>;
這里的其他答案是正確的,但是我想補充一點,如果您通常(但不總是)僅將FooOptions<T>
作為O
的類型傳遞,則可以考慮使用通用參數默認值 :
interface FooOptions<T> {
doSomething: (e: T) => void;
}
// note how O has a default value now
export interface FooInterface<T, O extends FooOptions<T> = FooOptions<T>> {
items: T[];
options: O;
}
這樣,當您打算將其作為FooOptions<T>
時,就FooOptions<T>
O
參數:
const t: FooInterface<string> = {
items: ["a", "b", "c"],
options: {
doSomething: (e) => {
console.log(e);
}
}
}
並且,如果您實際上希望O
比FooOptions<T>
更具體(這就是為什么要將其作為單獨的參數,對嗎?),您仍然可以這樣做:
interface MoreOptions {
doSomething: (e: string) => void;
doNothing: () => void;
}
const u: FooInterface<string, MoreOptions> = {
items: ["d", "e", "f"],
options: {
doSomething(e) { console.log(e); },
doNothing() { console.log("lol nvm"); }
}
}
哦,如果T
通常是string
那么您也可以為其添加默認值,然后不帶參數的FooInterface
將解釋為FooInterface<string, FooOptions<string>>
:
export interface FooInterface<T = string, O extends FooOptions<T> = FooOptions<T>> {
items: T[];
options: O;
}
const t: FooInterface = {
items: ["a", "b", "c"],
options: {
doSomething: (e) => {
console.log(e);
}
}
}
好的,希望對您有所幫助。 祝好運!
您可以使用TypeScript別名 :
// Declaration
type FooInterfaceOfType<T> = FooInterface<T, FooOptions<T>>;
// Usage
const t: FooInterfaceOfType<string>;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.