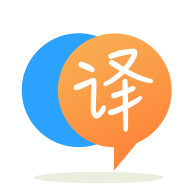
[英]Spring Boot - How to create entities and nested entities in single POST request
[英]How to create partly async post request in Spring boot?
我是Spring Boot的新手,我想創建一種異步請求。 它應該允許用戶上傳文件。 然后Spring應用程序應保存它並回答用戶文件已正確保存。
然后整個異步部分發生。 服務器應該在保存后立即開始處理文件(在后台)。 目前,它不在后台運行(用戶需要等到processFileInBackground
完成):
控制器:
@CrossOrigin
@RestController
public class ProcessFileController {
@Autowired
ProcessFileService processFileService;
@CrossOrigin
@PostMapping("/files/upload")
public ResponseEntity<String> singleFileUpload(@RequestParam("file") MultipartFile file) {
System.out.println("singleFileUpload tid: " + Thread.currentThread().getId());
bytes = file.getBytes();
// Save file...
String plainText = new String(bytes, StandardCharsets.UTF_8);
processFileInBackground(plainText);
return new ResponseEntity<>("File successfully uploaded!", HttpStatus.OK);
}
private void processFileInBackground(String plainText) {
processFileService = new ProcessFileService(plainText);
String result = processFileService.getResult();
}
}
服務:
@Service
public class ProcessFileService {
private FileProcessor fileProcessor;
public CompilerApiService(String plainText){
fileProcessor = new FileProcessor(code);
}
@Async
public String getResult(){
System.out.println("getResult tid: " + Thread.currentThread().getId());
// The call below takes a long time to finish
return fileProcessor.getResult();
}
}
組態:
@EnableAsync
@Configuration
public class AsyncConfig {
@Bean
public Executor threadPoolTaskExecutor() {
return new ConcurrentTaskExecutor(Executors.newCachedThreadPool());
}
}
Spring為您提供@Async注釋,您需要將異步邏輯分離到一個單獨的類中並使用此異步注釋您的方法,這將在一個單獨的線程中執行您的邏輯。 檢查這個https://spring.io/guides/gs/async-method/
必須要從調用者類外部調用異步方法才能在異步模式下執行,就像這樣
@CrossOrigin
@RestController
public class ProcessFileController {
@Autowired
ProcessFileService processFileService;
@CrossOrigin
@PostMapping("/files/upload")
public ResponseEntity<String> singleFileUpload(@RequestParam("file") MultipartFile file) {
bytes = file.getBytes();
// Save file...
String plainText = new String(bytes, StandardCharsets.UTF_8);
processFileInBackground(plainText);
return new ResponseEntity<>("File successfully uploaded!", HttpStatus.OK);
}
private void processFileInBackground(String plainText) {
processFileService = new ProcessFileService(plainText);
String result = processFileService.getResult();
}
}
服務
@Service
public class ProcessFileService {
private FileProcessor fileProcessor;
public CompilerApiService(String plainText){
fileProcessor = new FileProcessor(code);
}
@Async
public String getResult(){
return fileProcessor.getResult();
}
}
組態
@EnableAsync
@Configuration
public class AsyncConfig {
@Bean(name = "threadPoolExecutor")
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(7);
executor.setMaxPoolSize(42);
executor.setQueueCapacity(11);
executor.setThreadNamePrefix("threadPoolExecutor-");
executor.initialize();
return executor;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.