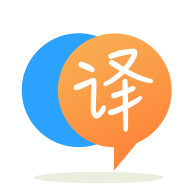
[英]In Python, what's the correct way to instantiate a class from a variable?
[英]What's the correct way to decrease a variable within a function?
有沒有辦法讓這個工作,或者我必須找到一種不同的邏輯方式? 作為我遇到的問題的一個例子,我寫得非常快。 每次猜測函數運行時,我想添加一個猜測。 問題是,當我退出函數然后重新進入時,猜測計數器重置為 0。而且我不能在定義的函數之外擁有變量“猜測”,這讓我感到困惑。
這樣做的正確方法是什么?
def guess():
x = 5
guesses = 0
while guesses < 5:
guess = input("Guess: ")
if guess == x:
print("You win")
else:
print("try again")
guesses = guesses + 1
movement()
def movement():
choice = input("left or guess")
if choice == "left":
movement()
if choice == "guess":
guess()
movement()
我希望能夠離開函數並重新輸入而無需重置 guesses 變量。
處理此問題的常用方法是將您的信息封裝在類的實例中。 該實例將保存諸如猜測次數和您想要維護的任何其他狀態等屬性。 這些方法將創建包括操縱這些屬性的行為。
在這里您可以創建一個新實例,包括傳遞初始猜測次數和答案的選項:
class Guess:
def __init__(self, answer = 2, guesses = 5): # 2 and 5 are deafaults if nothing is passed in
self.guesses = guesses
self.answer = answer
def guess(self):
guess = input("Guess: ")
if guess == self.answer:
print("you win")
else:
self.guesses -= 1
print(f"You have {self.guesses} left")
self.movement()
def movement(self):
choice = input("left or guess? ")
if choice == "left":
self.movement()
if choice == "guess":
self.guess()
g = Guess(answer = 5, guesses = 2) # make a new game using the passed in values
g.movement() # start game
我喜歡 OOP(面向對象編程),所以我會選擇另一個答案。 話雖如此,如果您願意,Python 有一些對此有用的東西,稱為generator
。 把它想象成一個記住狀態的函數。
def my_gen():
x = 0
while True:
# Yield acts just like a normal return, the functions stops
# after returning x.
yield x
# Next time the generator is called it will resume
# right here, add it will remember all the values
# it previously had (i.e. it remembers the last value for x.
x += 1
# Note a generator is called differently then a normal function
# then a normal function
g = my_gen()
print(next(g)) # prints 0
print(next(g)) # prints 1
print(next(g)) # prints 2
還包括發電機如何停止:
def my_gen2():
x = 2
while x > 0:
yield x
x -= 1
# Note that when a generator function
# has no more yields it will throw a
# StopIteration Exception
g = my_gen2()
print(next(g)) # prints 2
print(next(g)) # prints 1
print(next(g)) # This will cause an StopIteration Exception
# you can get around this either by:
g = my_gen2()
for x in g: # A for loop automatically stops on StopIteration
print(x)
# Or catch the Exception
try:
g = my_gen2()
for _ in range(5): # Just calling next enough times for it to break
print(next(g))
except StopIteration:
print("can't call generator any more")
您的代碼:
def guess():
x = 5
guesses = 0
num_tries = 1
while guesses < num_tries:
guess = input("Guess: ")
if guess == x:
print("You win")
yield 0
else:
guesses += 1
if guesses == num_tries:
yield 0 # Game over
else:
print("Try again")
yield 1 # Game can continue
# Don't add unneeded recusion.
# Python has a limited stack. Don't consume it
# for something you should do in a loop.
def play():
g = guess()
while True:
choice = input("left or guess: ")
print(choice)
if choice == "left":
continue
elif choice == "guess":
try:
if not next(g):
print("Game over")
break
except StopIteration:
print("Somehow called to many times")
break
else:
print("Invalid Entry")
play()
def guess(guesses = 0,x = 5):
choice = input("left or guess")
guesses = guesses + 1
if choice == "left":
return guess(guesses=guesses)
elif choice == "guess":
guessint = int(input("Guess(int): "))
if guessint == x:
print("You win")
print('number:', guesses)
return guesses
else:
print("try again")
return guess(guesses=guesses)
guess()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.