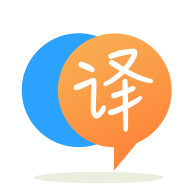
[英]How to check for words in a string even if the order is different - PYTHON
[英]How to check if a string is a substring of another even if not in order?
有沒有一種方法可以檢查一個字符串是否包含另一個字符串,但不一定按照正確的順序進行:
"hel my frend" in "hello my friend" = true
"hel my frend" in "my friend hello" = true
"hel my frend" in "h.e.l. .m.y. .f.r.e.n.d" = true
"hel my frend" in "my friend hello" = true
將子字符串更改為每個字符之間帶有.*
的正則表達式,然后使用re.search
。
import re
def fuzzy_substring(needle, haystack):
regex = ".*".join(re.escape(char) for char in needle)
return re.search(regex, haystack)
如果子字符串中的任何字符在正則表達式中具有特殊含義,則必須使用re.escape
。
您可以使用以編程方式構造的正則表達式:
import re
def find_string(where, target):
re_string = '.*'.join(target)
REGEX = re.compile(re_string)
return REGEX.findall(where)
print(find_string("hello my friend", "hel my frend"))
print(find_string("my friend hello", "hel my frend"))
print(find_string("h.e.l. .m.y. .f.r.e.n.d", "hel my frend"))
將打印:
['hello my friend']
[]
['h.e.l. .m.y. .f.r.e.n.d']
因此,如果函數的結果包含0個元素,則等於False,如果大於等於1,則為True。
如果我正確理解了您的問題和示例,則可以使用colections.Counter
對兩個字符串中的字符進行計數,看看從第一個字符減去第二個字符后是否還有字符。
from collections import Counter
def isin(a, b):
return not Counter(a) - Counter(b)
積極的例子:
isin("hel my frend", "hello my friend") # True
isin("hel my frend", "my friend hello") # True
isin("hel my frend", "h.e.l. .m.y. .f.r.e.n.d") # True
負樣本:
isin("hel my frgend", "hello my friend") # False
isin("hel my frend", "my frind hello") # False
isin("hel my frend", "h.e.l. .m.. .f.r.e.n.d") # False
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.