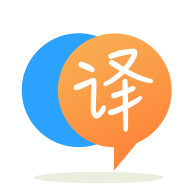
[英]How to implement multiple user types with different roles and permissions in Django?
[英]How to implement multiple roles in Django?
我想創建一個具有管理角色的應用程序,並且一個用戶可以擁有多個角色。 我在models.py中創建了兩個模型,一個用於角色,另一個用於Users。 我的models.py看起來像這樣:
class Role(models.Model):
DOCTOR = 1
NURSE = 2
DIRECTOR = 3
ENGENEER = 4
ROLE_CHOICES = {
(DOCTOR, 'doctor'),
(NURSE, 'nurse'),
(DIRECTOR, 'director'),
(ENGENEER, 'engeneer'),
}
id = models.PositiveIntegerField(choices = ROLE_CHOICES, primary_key = True)
def __str__(self):
return self.get_id_display()
class User(AbstractUser):
roles = models.ManyToManyField(Role)
現在,我要做的是從“用戶”表單中建立角色。 我的forms.py看起來像這樣:
class UserCreationForm(UserCreationForm):
fields = ('username', 'password1', 'password2')
model = User
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['username'].label = 'Insert username'
self.fields['password1'].label = 'Insert password'
self.fields['password2'].label = 'Again'
class RoleCreationForm(forms.ModelForm):
class Meta:
model = Role
fields = ('id',)
而且views.py看起來像這樣:
class UserCreateView(CreateView):
model = User
form_class = forms.UserCreationForm
template_name = 'user_form.html'
redirect_field_name = 'index.html'
success_url = reverse_lazy('accounts:index')
class RoleCreateView(CreateView):
model = Role
form_class = forms.RoleCreationForm
template_name = 'role_form.html'
redirect_field_name = 'index.html'
success_url = reverse_lazy('accounts:index')
如何在“用戶”表單中顯示所有角色並從中選擇多個角色?
我假設你UserCreationForm
是子類forms.ModelForm
還有:
1)沒有password1
或password2
在外地User
模式-只有password
。 因此,要進行密碼確認,您必須指定confirm_password
字段並覆蓋clean
方法以檢查它們是否相等。
2)要以表單形式顯示roles
,您只需在字段列表中指定'roles'
。 由於User
模型中存在roles
字段,因此django將找到正確的窗口小部件,並對其進行正確顯示和驗證。
3)您沒有寫過它,但是我想您在settings.py
添加了AUTH_USER_MODEL = '<you_app_name>.User'
? 這會將默認User
模型更改為您的應用程序模型。
4)您的UserCreateView
將以純文本形式存儲密碼,這是一個非常糟糕的主意。 閱讀有關密碼管理的更多信息,以了解如何正確存儲密碼。
class UserCreationForm(forms.ModelForm):
confirm_password = forms.CharField(widget=forms.PasswordInput())
class Meta:
fields = ('username', 'password', 'confirm_password', 'roles')
model = User
widgets = {
'password': forms.PasswordInput(),
'roles': forms.CheckboxSelectMultiple()
}
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['username'].label = 'Insert username'
self.fields['password'].label = 'Insert password'
def clean(self):
cleaned_data = super().clean()
password = cleaned_data.get("password")
confirm_password = cleaned_data.get("confirm_password")
if password != confirm_password:
raise forms.ValidationError(
"password and confirm_password does not match"
)
實際上,您可能只想創建OneToOneField
到User
且ManyToManyField
到Role
其他模型,而不是覆蓋django User
,因為替換django User
模型可能會導致問題。
查看django身份驗證視圖 。 您可以將AuthenticationForm
子類化以添加Roles
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.